Creating Webhooks with Node.js: A Comprehensive Tutorial
In today’s interconnected digital landscape, real-time communication between web services has become imperative for seamless integration and automation. Webhooks serve as a fundamental component in achieving this connectivity, enabling applications to receive instant notifications when specific events occur.
In this comprehensive tutorial, we’ll delve into the world of webhooks with Node.js, a popular JavaScript runtime known for its efficiency and versatility. By the end, you’ll have a solid understanding of how to implement webhooks in your Node.js applications, empowering you to streamline data flow and enhance the responsiveness of your systems.
What are Webhooks?
Webhooks are user-defined HTTP callbacks triggered by specific events. They enable real-time communication between web applications by allowing one application to send data to another in response to an event. This mechanism eliminates the need for constant polling, resulting in more efficient and responsive systems.
Setting Up Your Node.js Environment
Before diving into webhook implementation, ensure you have Node.js installed on your system. You can download it from the official website: Node.js
Once Node.js is installed, create a new directory for your project and initialize a new Node.js project using the following commands:
mkdir webhook-tutorial cd webhook-tutorial npm init -y
Implementing Webhooks
Now, let’s implement a basic webhook receiver using Node.js and Express, a minimalist web framework for Node.js.
- Install Dependencies:
Start by installing the necessary dependencies—Express and body-parser—to handle incoming webhook payloads:
npm install express body-parser
Create Server:
Set up a basic Express server to listen for incoming webhook requests:
const express = require('express'); const bodyParser = require('body-parser'); const app = express(); app.use(bodyParser.json()); app.post('/webhook', (req, res) => { console.log('Received webhook payload:', req.body); // Handle webhook payload res.status(200).send('Webhook received successfully'); }); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Test Your Webhook:
To test your webhook, you can use tools like ngrok to expose your local server to the internet temporarily:
ngrok http 3000
- Copy the generated forwarding URL (e.g., https://your-ngrok-id.ngrok.io) and use it to configure your webhook provider to send requests to your server.
Advanced Usage and Best Practices
- Security: Implement authentication and validation mechanisms to ensure that incoming webhook requests are genuine and from trusted sources.
- Error Handling: Handle errors gracefully and implement retry mechanisms to handle failed webhook deliveries.
- Logging and Monitoring: Set up logging and monitoring to track webhook activity and diagnose issues effectively.
Conclusion
In this tutorial, we’ve explored the fundamentals of creating webhooks with Node.js. By leveraging this powerful mechanism, you can facilitate seamless communication between your applications and unlock a world of possibilities for automation and integration.
Ready to supercharge your applications with webhooks? Dive deeper into the documentation of your favorite services and start integrating webhooks into your Node.js projects today!
External Resources:
Now, go forth and revolutionize your applications with the power of webhooks and Node.js! Happy coding!
Table of Contents
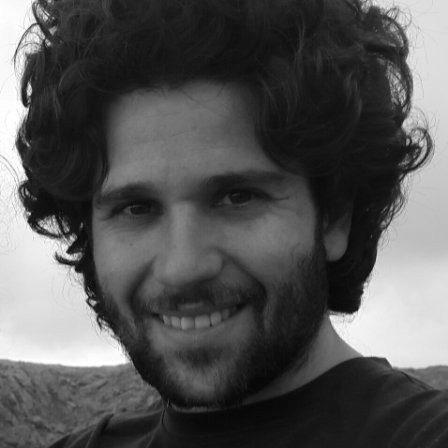
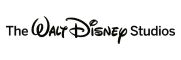