What is CSRF protection in Node.js?
CSRF (Cross-Site Request Forgery) is a security vulnerability that occurs when an attacker tricks a user’s browser into making unintended HTTP requests on behalf of the user. CSRF attacks typically target state-changing requests, such as changing a user’s password or transferring funds, by exploiting the user’s authenticated session.
CSRF protection in Node.js involves implementing measures to prevent or mitigate CSRF attacks. One common method of CSRF protection is to use CSRF tokens, which are unique tokens generated by the server and embedded in HTML forms or HTTP headers. When a user submits a form or makes a request, the server verifies the presence and validity of the CSRF token to ensure that the request originated from the intended user.
Here’s how you can implement CSRF protection in Node.js with the csurf middleware:
- Install csurf: First, install the csurf middleware package using npm:
Copy code npm install csurf
- Use csurf middleware: In your Express.js application, use the csurf middleware by requiring it and adding it to the middleware stack:
javascript Copy code const express = require('express'); const csrf = require('csurf'); const app = express(); app.use(csrf({ cookie: true }));
- This will add a CSRF token to the response locals (res.locals.csrfToken), which you can include in your HTML forms.
- Include CSRF token in HTML forms: In your HTML forms, include the CSRF token as a hidden input field:
html Copy code <form action="/submit" method="post"> <input type="hidden" name="_csrf" value="<%= csrfToken %>"> <!-- Other form fields --> <button type="submit">Submit</button> </form>
- Verify CSRF token on the server: When handling form submissions or requests, verify the CSRF token sent by the client:
javascript Copy code app.post('/submit', (req, res) => { if (req.body && req.body._csrf === req.csrfToken()) { // CSRF token is valid, process the request } else { // CSRF token is invalid or missing, handle the error } });
By using csurf middleware and CSRF tokens, you can effectively protect your Node.js applications from CSRF attacks, ensuring the integrity and security of user interactions.
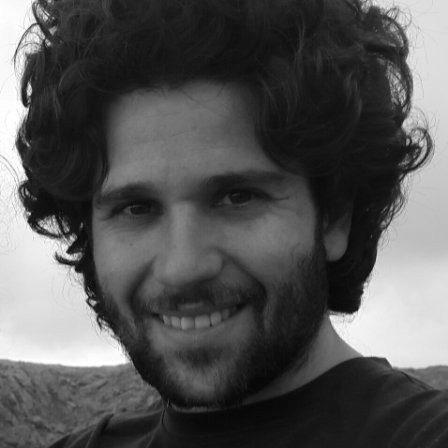
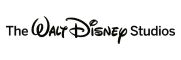