Developing IoT Applications with Node.js and MQTT
In the rapidly expanding world of the Internet of Things (IoT), Node.js has emerged as a powerhouse for developing scalable and efficient applications. When combined with the lightweight messaging protocol MQTT (Message Queuing Telemetry Transport), Node.js offers a robust platform for building IoT solutions that can handle massive amounts of data transfer efficiently. In this guide, we’ll delve into the fundamentals of developing IoT applications with Node.js and MQTT, explore some real-world examples, and discuss best practices for building scalable and reliable systems.
Understanding Node.js and MQTT
Node.js, built on Chrome’s V8 JavaScript engine, is renowned for its event-driven, non-blocking I/O model, making it perfect for handling asynchronous operations commonly found in IoT applications. Its extensive package ecosystem through npm (Node Package Manager) provides developers with a wide array of tools and libraries for building IoT solutions rapidly.
On the other hand, MQTT is a lightweight messaging protocol designed for low-bandwidth, high-latency, or unreliable networks, which are typical characteristics of IoT environments. It operates on a publish-subscribe model, where clients connect to a broker and publish messages to topics or subscribe to receive messages from topics.
Setting Up a Node.js Environment for IoT Development
Before diving into IoT application development with Node.js and MQTT, it’s essential to set up your development environment. Ensure you have Node.js installed on your system, along with npm for managing dependencies. You can download and install Node.js from the official website or use a package manager like npm or yarn.
Once Node.js is set up, create a new project directory and initialize a new Node.js project using the following commands:
mkdir iot-project cd iot-project npm init -y
Next, install the MQTT.js library, a popular MQTT client for Node.js, using npm:
npm install mqtt
With the environment set up, let’s move on to building our IoT application.
Developing IoT Applications with Node.js and MQTT
Imagine you’re building a smart home system where various sensors and actuators communicate with a central hub to control and monitor devices remotely. Node.js, coupled with MQTT, offers an efficient way to implement such a system.
In your Node.js application, you’ll establish a connection to an MQTT broker (e.g., Mosquitto) and subscribe to specific topics to receive sensor data or commands. You’ll also publish messages to control actuators or send notifications based on sensor readings.
Here’s a simplified example of a Node.js script that subscribes to a temperature sensor topic and publishes commands to control a smart light:
const mqtt = require('mqtt'); // Connect to MQTT broker const client = mqtt.connect('mqtt://broker.example.com'); client.on('connect', () => { console.log('Connected to MQTT broker'); // Subscribe to temperature sensor topic client.subscribe('sensors/temperature', (err) => { if (!err) { console.log('Subscribed to temperature sensor topic'); } }); }); // Handle incoming messages client.on('message', (topic, message) => { // Parse message and take appropriate action console.log(`Received message on topic ${topic}: ${message.toString()}`); // Example: Control smart light based on temperature if (topic === 'sensors/temperature') { const temperature = parseFloat(message.toString()); if (temperature > 25) { client.publish('actuators/light', 'on'); } else { client.publish('actuators/light', 'off'); } } }); // Publish commands to control smart light // client.publish('actuators/light', 'on'); // Example command
In this example, the script connects to an MQTT broker, subscribes to the sensors/temperature topic, and reacts to incoming temperature readings by publishing commands to control a smart light.
Real-World Examples
- Smart Agriculture: In agriculture, IoT sensors can monitor soil moisture, temperature, and humidity, allowing farmers to optimize irrigation and crop growth. Node.js and MQTT can facilitate real-time data collection and analysis for precision farming.
- Industrial Automation: Manufacturers utilize IoT devices to monitor machinery health, track inventory, and streamline production processes. Node.js, with its asynchronous nature, is well-suited for handling concurrent operations in industrial IoT applications.
- Smart Cities: Municipalities deploy IoT sensors for traffic management, waste management, and environmental monitoring. Node.js enables developers to build scalable and responsive applications to address the complex challenges of urban environments.
Conclusion
Developing IoT applications with Node.js and MQTT empowers developers to create scalable, efficient, and responsive systems for a wide range of use cases. By leveraging the asynchronous nature of Node.js and the lightweight messaging protocol MQTT, developers can build real-time, event-driven IoT solutions that meet the demands of today’s interconnected world.
External Links:
Start building your IoT applications today with Node.js and MQTT, and unlock endless possibilities in the world of connected devices.
Table of Contents
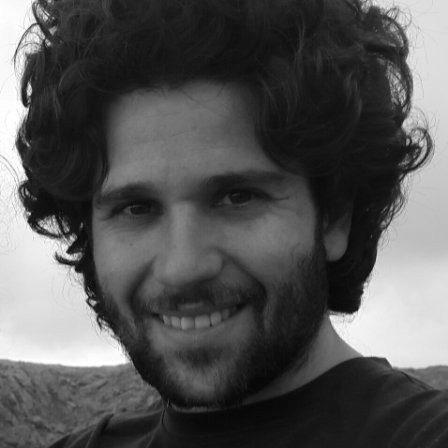
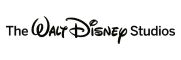