What is the purpose of the DNS module in Node.js?
The dns module in Node.js provides functions for performing DNS (Domain Name System) lookups and resolving domain names to IP addresses or vice versa. It allows Node.js applications to interact with the DNS infrastructure and resolve domain names to their corresponding IP addresses, which is essential for network communication and connectivity.
Some key functionalities provided by the dns module include:
- Hostname Resolution:
-
- The dns.lookup function resolves a hostname (domain name) to its corresponding IP address asynchronously. It supports both IPv4 and IPv6 addresses.
Example:
javascript
Copy code
const dns = require('dns'); dns.lookup('example.com', (err, address, family) => { if (err) throw err; console.log(`IP address: ${address}`); console.log(`IP version: IPv${family}`); });
- Reverse DNS Lookup:
-
- The dns.reverse function performs a reverse DNS lookup by resolving an IP address to its corresponding hostname(s) asynchronously.
Example:
javascript
Copy code
const dns = require('dns'); dns.reverse('8.8.8.8', (err, hostnames) => { if (err) throw err; console.log(`Hostnames: ${hostnames.join(', ')}`); });
- Custom DNS Resolvers:
-
- Node.js applications can configure custom DNS resolvers using the dns.setServers function to specify custom DNS server addresses for resolving domain names.
- Error Handling:
-
- The dns module provides error handling mechanisms to handle DNS lookup errors, network issues, or timeouts gracefully.
The dns module is particularly useful for building networked applications in Node.js that rely on domain names for communication, such as web servers, HTTP clients, email clients, or DNS servers. It enables applications to resolve domain names dynamically, perform network operations, and establish connections with remote hosts effectively. Additionally, the dns module integrates seamlessly with other Node.js modules and network-related APIs, facilitating robust and reliable network communication.
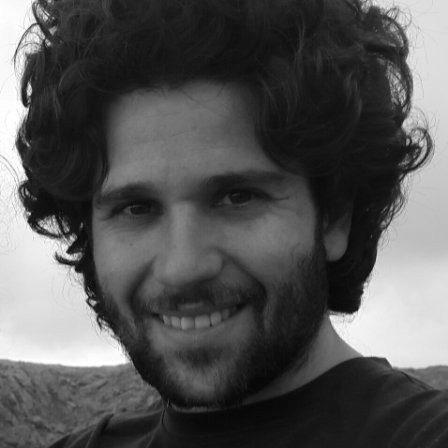
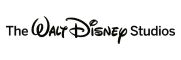