How do you handle file uploads in Node.js?
Handling file uploads in Node.js typically involves using middleware libraries such as multer or formidable along with Express.js to parse and process incoming multipart/form-data requests containing file uploads.
Here’s how to handle file uploads using multer middleware in an Express.js application:
Install the multer package using npm:
Copy code npm install multer
In your Node.js application, import the multer middleware and configure it to specify the destination directory for uploaded files:
- javascript
Copy code const express = require('express'); const multer = require('multer'); const app = express(); // Configure multer middleware const storage = multer.diskStorage({ destination: 'uploads/', filename: (req, file, cb) => { cb(null, file.originalname); } }); const upload = multer({ storage: storage });
Define a route handler to handle file uploads using the upload middleware:
javascript Copy code app.post('/upload', upload.single(' file'), (req, res) => { // Access uploaded file via req.file if (!req.file) { return res.status(400).send('No file uploaded'); } res.send('File uploaded successfully'); }); bash Copy code
In the HTML form on the client-side, set the enctype attribute to multipart/form-data and include an input field of type file:
```html <form action="/upload" method="post" enctype="multipart/form-data"> <input type="file" name="file"> <button type="submit">Upload</button> </form>
With this setup, when a user submits the form with a file selected, the multer middleware will handle parsing the multipart/form-data request and saving the uploaded file to the specified destination directory. The route handler then receives the uploaded file object in req.file and can perform further processing, such as saving the file to a database or serving it to the client.
Handling file uploads in Node.js requires careful consideration of security, file size limits, and storage requirements. Middleware libraries like multer provide options to configure these settings and ensure secure and efficient file uploads in Node.js applications.
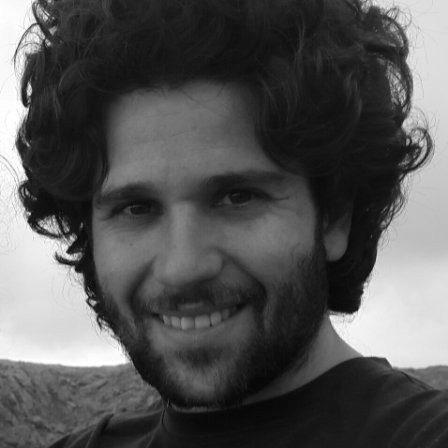
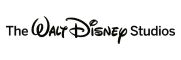