Node.js Q & A
How do you handle form validation in Node.js?
Form validation is a critical aspect of web development to ensure that user-submitted data meets specific requirements and is safe to process. In Node.js applications, form validation can be implemented using various techniques and libraries to validate input data on the server-side before processing it further or storing it in a database.
Here’s how you can handle form validation in a Node.js application:
- Manual Validation: You can perform form validation manually by writing custom validation logic in your route handlers or middleware functions. This involves checking each input field against predefined rules or regular expressions to ensure that it meets the required criteria, such as data type, length, format, and presence.
javascript Copy code app.post('/submit', (req, res) => { const { username, email, password } = req.body; // Validate username if (!username || username.length < 3) { return res.status(400).send('Invalid username'); } // Validate email if (!email || !isValidEmail(email)) { return res.status(400).send('Invalid email'); } // Validate password if (!password || password.length < 6) { return res.status(400).send('Invalid password'); } // Process valid form data // ... });
- Validation Libraries: Alternatively, you can use validation libraries like Joi, Validator.js, express-validator, or Yup to simplify form validation in your Node.js application. These libraries provide predefined validation rules, custom validation functions, and middleware for validating request data, making it easier to handle complex validation scenarios.
javascript Copy code const { body, validationResult } = require('express-validator'); app.post('/submit', body('username').isLength({ min: 3 }).withMessage('Username must be at least 3 characters long'), body('email').isEmail().withMessage('Invalid email'), body('password').isLength({ min: 6 }).withMessage('Password must be at least 6 characters long'), (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Process valid form data // ... });
- Sanitization: In addition to validation, it’s essential to sanitize user input to prevent security vulnerabilities such as XSS (Cross-Site Scripting) attacks. Sanitization involves removing or escaping potentially dangerous characters from user input to prevent malicious code execution.
By implementing robust form validation techniques in your Node.js application, you can ensure that user-submitted data is accurate, secure, and suitable for further processing, reducing the risk of errors and security vulnerabilities.
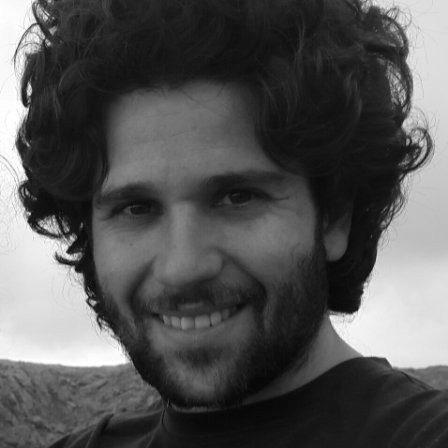
Previously at
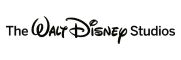
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.