How do you handle sessions in Node.js?
Handling sessions in Node.js involves managing user sessions to maintain state between HTTP requests. Sessions are crucial for maintaining user authentication, tracking user activity, and storing user-specific data. There are several approaches to handling sessions in Node.js, but one common method is to use middleware libraries like express-session with Express.js.
Here’s how you can handle sessions in Node.js with express-session:
- Install express-session: First, install the express-session middleware package using npm:
Copy code npm install express-session
- Use express-session middleware: In your Express.js application, use the express-session middleware by requiring it and adding it to the middleware stack:
javascript Copy code const express = require('express'); const session = require('express-session'); const app = express(); app.use(session({ secret: 'your-secret-key', resave: false, saveUninitialized: true }));
Here, secret is a string used to sign the session ID cookie, resave determines whether to save the session on every request even if it hasn’t changed, and saveUninitialized determines whether to save uninitialized (new, but not modified) sessions.
- Accessing Session Data: Once the session middleware is set up, you can access session data within your route handlers using req.session. For example, to set a session variable:
javascript Copy code app.get('/login', (req, res) => { req.session.user = { username: 'example' }; res.send('Logged in'); });
- Destroying Sessions: To destroy a session and clear session data, you can use req.session.destroy():
javascript Copy code app.get('/logout', (req, res) => { req.session.destroy(); res.send('Logged out'); });
By using express-session, you can easily implement session management in Node.js applications, allowing you to maintain user sessions securely and manage user-specific data.
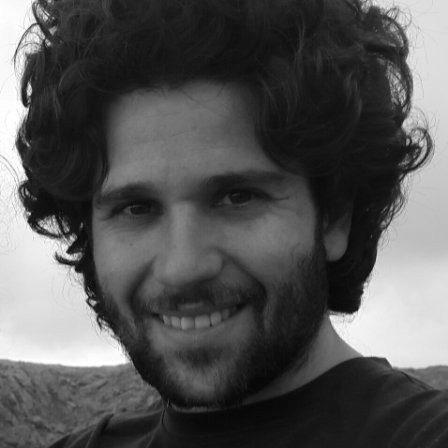
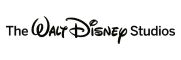