Implementing Internationalization in Node.js Applications
In today’s interconnected world, reaching a global audience is essential for the success of any online application. Whether you’re a startup aiming to expand internationally or an established company catering to diverse markets, implementing internationalization (i18n) in your Node.js applications is crucial. Internationalization involves designing your application to support multiple languages and cultural preferences, ensuring a seamless user experience regardless of location or language proficiency.
Understanding Internationalization
At its core, internationalization is about making your application adaptable to different languages, regions, and cultural conventions. It involves separating text and other locale-specific elements from the application’s source code, allowing them to be easily replaced or customized for different locales.
Key Components of Internationalization
1. Language Strings
All text displayed to users should be stored separately from the application code, typically in resource files or a database. These language strings are then dynamically loaded based on the user’s language preference.
2. Locale Detection
Detecting the user’s locale is essential for serving localized content. This can be achieved through HTTP headers, user preferences, or even geolocation.
3. Date and Time Formatting
Dates, times, and other locale-specific formats should be displayed according to the user’s preferences.
4. Number Formatting
Numeric values, such as currency, should be formatted based on the user’s locale conventions, including decimal separators and digit grouping.
Implementing Internationalization in Node.js
Now let’s dive into how you can implement internationalization in your Node.js applications:
1. Use a Library or Framework
Utilizing libraries like i18next, Intl.js, or frameworks like Express.js with middleware such as i18n-express can simplify the internationalization process. These tools provide features for loading language files, managing locales, and rendering localized content.
2. Organize Language Strings
Store language strings in separate JSON or JavaScript files organized by language and module. For example, you might have en.json for English and fr.json for French, each containing key-value pairs for different text elements.
3. Implement Locale Detection
Detect the user’s locale based on their preferences or browser settings. Express.js middleware like i18n-express can automatically handle locale detection based on request headers or query parameters.
4. Localize Content
Replace static text in your templates with dynamic placeholders linked to language strings. For example:
// English res.render('index', { title: req.t('welcome.title') }); // French res.render('index', { title: req.t('welcome.title') });
5. Format Dates and Numbers
Use the Intl.DateTimeFormat and Intl.NumberFormat APIs to format dates, times, and numbers according to the user’s locale. For example:
const date = new Date(); const formattedDate = new Intl.DateTimeFormat(req.locale).format(date);
Conclusion
Implementing internationalization in Node.js applications is essential for catering to a global audience. By separating language-specific elements, detecting user locales, and localizing content, you can create a more inclusive and user-friendly experience for your international users.
For further reading on internationalization in Node.js, check out these resources:
- Express.js Internationalization Middleware
- Mozilla Developer Network – JavaScript Internationalization API
- i18next Documentation
Start internationalizing your Node.js applications today and unlock new opportunities for growth and engagement on a global scale!
Table of Contents
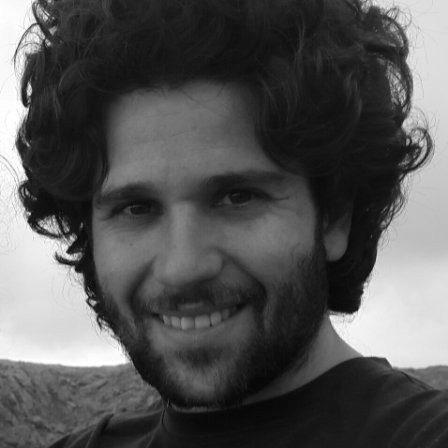
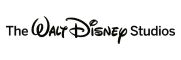