Navigating the Realm of Hiring Node.js Developers: A Comprehensive Node Developer Interview Questions Guide
Node.js, the groundbreaking runtime environment, has revolutionized server-side JavaScript programming. To build exceptional back-end systems and applications, hiring skilled Node.js developers is pivotal. This guide serves as your compass through the hiring voyage, empowering you to assess candidates’ technical prowess, problem-solving abilities, and Node.js expertise. Let’s set sail on this exploration, uncovering essential interview questions and strategies to identify the perfect Node.js developer for your team.
Table of Contents
1. How to Hire Node.js Developers
Embarking on the quest to hire Node.js developers? Follow these steps for a successful journey:
- Job Requirements: Define specific job requirements, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Node.js proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Node.js Developers to Look For
When evaluating Node.js developers, be on the lookout for these core skills:
- Node.js Mastery: A strong grasp of Node.js concepts, event-driven architecture, and asynchronous programming.
- Express.js Proficiency: Familiarity with building RESTful APIs and web applications using the Express.js framework.
- Database Expertise: Knowledge of working with databases, querying languages like SQL, and using ORM libraries like Sequelize.
- API Integration: Experience integrating with third-party APIs and services to enhance application functionality.
- Authentication and Authorization: Skill in implementing secure user authentication and authorization mechanisms.
- Testing Know-How: Proficiency in writing unit tests and using testing frameworks like Mocha or Jest.
- Problem-Solving Skills: Ability to dissect intricate problems and devise effective solutions.
3. Overview of the Node.js Developer Hiring Process
Here’s an overview of the Node.js developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create compelling job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting Node.js Developer Interview Questions
Develop a comprehensive set of interview questions covering Node.js intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Node.js Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ Node.js skills:
Q1. Explain the concept of the Event Loop in Node.js and how it works.
A: The Event Loop is a fundamental concept in Node.js that enables asynchronous, non-blocking operations. It allows Node.js to handle multiple tasks concurrently, enhancing performance.
Q2. Write a Node.js function that reads a file asynchronously and returns its content.
const fs = require('fs'); function readFileAsync(filePath, callback) { fs.readFile(filePath, 'utf8', (err, data) => { if (err) { callback(err, null); return; } callback(null, data); }); }
Q3. What is middleware in the context of Node.js applications, and how is it used in frameworks like Express.js?
A: Middleware in Node.js is a series of functions that are executed sequentially in the request-response cycle. In Express.js, middleware functions can perform tasks such as logging, authentication, and error handling.
Q4. Explain the purpose of npm (Node Package Manager) and how it is used in Node.js projects.
A: npm is a package manager for Node.js that allows developers to install, manage, and share reusable code packages. It is used to manage project dependencies, run scripts, and publish packages to the npm registry.
Q5. Write a Node.js route handler using Express.js that returns a JSON response with a list of items.
const express = require('express'); const app = express(); app.get('/items', (req, res) => { const items = ['item1', 'item2', 'item3']; res.json(items); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Q6. Explain the concept of promises in Node.js and how they help manage asynchronous operations.
A: Promises in Node.js provide a way to handle asynchronous operations in a more structured and readable manner. They allow you to represent a value that might be available now, or in the future, or never.
Q7. How does Node.js handle concurrency and scalability?
A: Node.js is designed to handle concurrency through its non-blocking, event-driven architecture. It uses a single-threaded event loop to handle multiple asynchronous tasks concurrently.
Q8. Write a Node.js script that connects to a MongoDB database using the Mongoose library and performs a basic query.
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/mydatabase', { useNewUrlParser: true }); const Item = mongoose.model('Item', { name: String }); Item.find({}, (err, items) => { if (err) { console.error(err); return; } console.log('Items:', items); });
Q9. What is the purpose of the require
function in Node.js, and how is it used to load modules?
A: The require
function in Node.js is used to load external modules or files. It searches for the specified module in the Node.js core modules, local modules, and third-party modules installed via npm.
Q10. Write a Node.js function that makes an HTTP GET request to an external API and returns the response data.
const http = require('http'); function fetchData(url, callback) { http.get(url, response => { let data = ''; response.on('data', chunk => { data += chunk; }); response.on('end', () => { callback(null, data); }); }).on('error', error => { callback(error, null); }); }
5. Hiring Node.js Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected Node.js developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By harnessing the expertise of CloudDevs, you can effortlessly identify and hire exceptional Node.js developers, ensuring your team possesses the skills required to build remarkable back-end systems and applications.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess Node.js developers comprehensively. Whether you’re orchestrating efficient server-side logic or crafting powerful APIs, securing the right Node.js developers for your team is the foundation of your project’s triumph.
Table of Contents
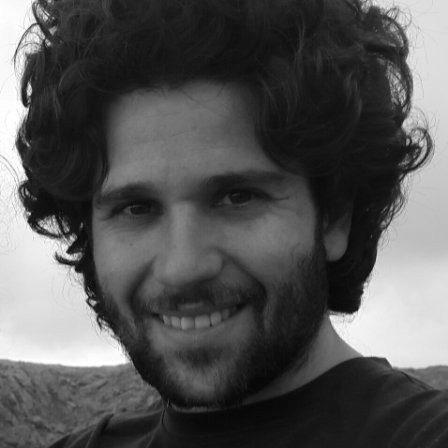
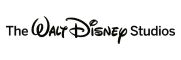