Node.js Q & A
How do you handle memory leaks in Node.js?
Memory leaks in Node.js can occur due to improper memory management, circular references, long-lived objects, or external dependencies. Handling memory leaks effectively involves identifying memory leaks, analyzing memory usage, and implementing corrective actions to prevent memory consumption from exceeding available resources. Here’s how you can handle memory leaks in Node.js:
- Memory Profiling:
-
-
- Use built-in memory profiling tools like the Node.js Inspector, Chrome DevTools, or third-party profilers to analyze memory usage, detect memory leaks, and identify memory-hungry objects or functions.
-
- Heap Snapshots:
-
-
- Capture heap snapshots during runtime to monitor memory allocations, object references, and memory growth over time. Analyze heap snapshots to identify memory leaks, detect memory spikes, and track memory usage patterns.
-
- Memory Monitoring:
-
-
- Implement memory monitoring mechanisms to track memory usage metrics, including heap size, heap usage, and memory allocations. Use monitoring libraries or custom metrics instrumentation to collect memory usage data and monitor memory trends.
-
- Garbage Collection Optimization:
-
-
- Optimize garbage collection settings and strategies to reclaim unused memory more efficiently. Adjust garbage collection thresholds, intervals, and algorithms based on application characteristics, workload patterns, and memory usage requirements.
-
- Resource Cleanup:
-
-
- Ensure proper resource cleanup and disposal of objects, resources, and event listeners to prevent memory leaks. Use removeListener() to remove event listeners, clearInterval() to clear intervals, and close() to close file descriptors, streams, or connections.
-
- Avoid Global Variables:
-
-
- Minimize the use of global variables and long-lived objects to reduce memory retention and avoid unintentional memory leaks. Limit the scope and lifetime of variables, functions, and objects to prevent excessive memory consumption.
- Circular Reference Detection:
- Identify and eliminate circular references between objects to prevent memory leaks caused by unreachable object chains. Break circular references manually or use weak references, weak maps, or proxy objects to mitigate memory retention issues.
-
- Memory Leak Detection Libraries:
-
-
- Utilize memory leak detection libraries like memwatch-next, heapdump, or leakage to automatically detect and report memory leaks in Node.js applications. Integrate leak detection mechanisms into testing, profiling, and monitoring workflows for proactive memory leak management.
-
- Continuous Testing and Profiling:
-
-
- Conduct regular performance testing, memory profiling, and code reviews to identify and address memory leaks early in the development lifecycle. Integrate memory leak detection into CI/CD pipelines and automated testing frameworks for continuous memory monitoring.
-
- Version Upgrades and Bug Fixes:
-
- Keep Node.js runtime, dependencies, and third-party modules up to date to leverage performance improvements, memory optimizations, and bug fixes. Monitor release notes, changelogs, and security advisories for Node.js updates addressing memory leak issues.
By following these best practices, developers can effectively manage memory leaks in Node.js applications, optimize memory usage, and ensure efficient utilization of system resources. Proactive memory leak detection, monitoring, and mitigation strategies help maintain application stability, reliability, and performance over time.
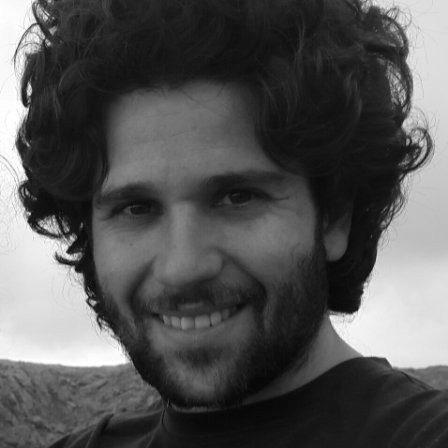
Previously at
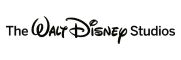
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.