Performance Tuning Node.js Applications: Tips and Techniques
Introduction to Node.js Performance Tuning
Node.js is widely recognized for its efficiency and scalability, but like any technology, it requires careful tuning to achieve optimal performance. Performance tuning in Node.js involves identifying and addressing bottlenecks, optimizing code, and ensuring that the application runs efficiently under various workloads. This article explores key techniques and best practices for tuning Node.js applications.
Understanding Node.js Performance Challenges
Node.js operates on a single-threaded event loop, which means that while it’s highly efficient for I/O-bound tasks, it can be vulnerable to performance issues if the event loop gets blocked. Common challenges include handling high concurrency, optimizing CPU-bound tasks, and managing memory usage.
1. Monitoring and Profiling Your Node.js Application
The first step in performance tuning is to understand how your application behaves under load. Monitoring and profiling tools can help you identify bottlenecks and areas that need optimization.
Example: Using `node –inspect` for Profiling
Node.js comes with a built-in inspector that you can use to profile your application.
```bash node --inspect index.js ```
You can then connect to `chrome://inspect` in your Chrome browser to view the performance profile of your application. This allows you to see the CPU usage, memory allocation, and event loop delays.
2. Optimizing the Event Loop
The event loop is the core of Node.js performance. Ensuring that the event loop is not blocked by long-running tasks is crucial.
Example: Offloading CPU-Intensive Tasks
For CPU-bound tasks, consider using the `worker_threads` module to offload work to separate threads.
```javascript const { Worker } = require('worker_threads'); function runService(workerData) { return new Promise((resolve, reject) => { const worker = new Worker('./worker.js', { workerData }); worker.on('message', resolve); worker.on('error', reject); worker.on('exit', (code) => { if (code !== 0) reject(new Error(`Worker stopped with exit code ${code}`)); }); }); } runService({ task: 'some CPU intensive task' }) .then(result => console.log(result)) .catch(err => console.error(err)); ```
This approach ensures that CPU-intensive tasks do not block the event loop.
3. Managing Memory and Garbage Collection
Memory management is another critical aspect of performance tuning. Node.js uses the V8 engine, which has its garbage collection mechanism, but inefficient memory usage can still lead to performance issues.
Example: Using Heap Snapshots to Identify Memory Leaks
You can use the `heapdump` module to take heap snapshots and analyze memory usage.
```javascript const heapdump = require('heapdump'); setInterval(() => { heapdump.writeSnapshot((err, filename) => { console.log(`Heap snapshot written to ${filename}`); }); }, 60000); ```
Heap snapshots can be analyzed using Chrome DevTools to identify memory leaks and optimize memory usage.
4. Implementing Caching Strategies
Caching can significantly improve the performance of your Node.js applications by reducing the need to recompute results or fetch data from slow resources.
Example: Using In-Memory Caching with `node-cache`
`node-cache` is a simple in-memory caching module for Node.js.
```javascript const NodeCache = require('node-cache'); const cache = new NodeCache({ stdTTL: 100, checkperiod: 120 }); function getCachedData(key) { const value = cache.get(key); if (value) { return value; } else { // Assume fetchData is a function that fetches data const data = fetchData(); cache.set(key, data); return data; } } ```
By caching frequently accessed data, you can reduce latency and improve response times.
5. Scaling Node.js Applications
Scaling is an essential consideration for performance tuning. Node.js applications can be scaled horizontally by running multiple instances behind a load balancer.
Example: Using the Cluster Module to Scale
The `cluster` module allows you to create multiple worker processes to handle requests concurrently.
```javascript const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`Worker ${worker.process.pid} died`); cluster.fork(); }); } else { http.createServer((req, res) => { res.writeHead(200); res.end('Hello World\n'); }).listen(8000); } ```
This approach leverages multiple CPU cores, enhancing the application’s ability to handle high traffic.
Conclusion
Performance tuning in Node.js is a multifaceted process that involves monitoring, profiling, and optimizing various aspects of your application. By following the tips and techniques outlined in this article, you can significantly enhance the performance of your Node.js applications, ensuring they run efficiently under different workloads.
Further Reading
Table of Contents
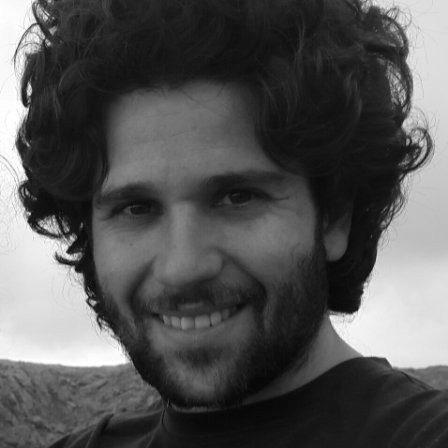
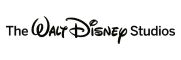