Leveraging the Power of Promises in Node.js Development
Asynchronous programming lies at the heart of modern web development, especially in the Node.js ecosystem. Traditional callback-based patterns can lead to callback hell and code that’s hard to read and maintain. This is where Promises come to the rescue. Promises offer a more structured and readable way to handle asynchronous operations, making your Node.js applications cleaner, more efficient, and less prone to errors. In this article, we’ll delve into the world of Promises, exploring their significance and how to leverage their power in your Node.js development journey.
Table of Contents
1. Understanding the Asynchronous Nature of Node.js
Node.js, built on the V8 JavaScript engine, is designed to handle a large number of concurrent connections efficiently. However, this concurrency introduces an asynchronous programming model that enables non-blocking operations, allowing the server to handle multiple requests simultaneously without getting bogged down. While this design is powerful, it also introduces complexity, especially when dealing with asynchronous code.
Traditional callback-based approaches can lead to deeply nested and hard-to-read code. This is often referred to as “callback hell,” where the code becomes a tangled mess of callbacks within callbacks. Promises offer a solution to this problem by providing a more structured and organized way to work with asynchronous operations.
2. Introducing Promises
Promises are a fundamental concept in JavaScript that provides a cleaner way to handle asynchronous operations. A Promise represents a value that might be available now, or in the future, or never. It has three states:
- Pending: The initial state; the promise is neither fulfilled nor rejected.
- Fulfilled: The promise has resolved, and the value is available.
- Rejected: An error occurred, and the promise is rejected.
- Promises allow you to chain asynchronous operations and handle their results and errors more gracefully. This leads to code that’s easier to read, understand, and maintain.
3. Creating a Promise
Creating a promise is straightforward. The Promise constructor takes a function with two parameters: resolve and reject. Inside this function, you perform your asynchronous operations and call resolve when they’re successful or reject when an error occurs.
javascript const fetchData = new Promise((resolve, reject) => { // Simulating an asynchronous operation setTimeout(() => { const data = "Some fetched data"; if (data) { resolve(data); // Operation succeeded } else { reject("Error fetching data"); // Operation failed } }, 1000); });
4. Chaining Promises
One of the significant advantages of Promises is their ability to be chained together. This is accomplished using the .then() method, which allows you to attach callbacks to handle the fulfillment of the Promise.
javascript fetchData .then((data) => { console.log("Fetched data:", data); return data.toUpperCase(); }) .then((uppercasedData) => { console.log("Uppercased data:", uppercasedData); }) .catch((error) => { console.error("Error:", error); });
In this example, if the initial data fetch succeeds, the first .then() block is executed, and it returns the uppercased version of the data. The second .then() block then processes the uppercased data. If an error occurs at any stage, the .catch() block handles it.
5. Handling Errors
Error handling is a crucial aspect of any application. Promises provide a consistent and elegant way to handle errors across asynchronous operations. The .catch() method at the end of a promise chain can capture any errors that occurred in any previous step.
javascript fetchData .then((data) => { // Processing data }) .catch((error) => { console.error("Error:", error); });
6. Promisifying Callback-based Functions
Many Node.js APIs still use the callback pattern. However, you can easily convert these APIs into promise-based functions using a technique called “promisification.” Node.js provides a utility module called util that includes a promisify function, which can be used to convert callback-based functions into promise-based ones.
javascript const fs = require("fs"); const util = require("util"); const readFile = util.promisify(fs.readFile); readFile("file.txt") .then((data) => { console.log("File data:", data.toString()); }) .catch((error) => { console.error("Error reading file:", error); });
7. Handling Multiple Promises
There are scenarios where you need to wait for multiple promises to complete before proceeding. This can be achieved using Promise.all(), which takes an array of promises and returns a new promise that fulfills when all the input promises are fulfilled.
javascript const promise1 = fetchData(); const promise2 = fetchAnotherData(); Promise.all([promise1, promise2]) .then((results) => { const [result1, result2] = results; console.log("Result 1:", result1); console.log("Result 2:", result2); }) .catch((error) => { console.error("Error:", error); });
8. Async/Await: Syntactic Sugar for Promises
ES6 introduced the async/await syntax, which provides a more synchronous-looking way to work with promises. Under the hood, async/await is built on top of promises, making asynchronous code appear almost synchronous.
javascript async function fetchDataAndProcess() { try { const data = await fetchData(); console.log("Fetched data:", data); const processedData = await process(data); console.log("Processed data:", processedData); } catch (error) { console.error("Error:", error); } } fetchDataAndProcess();
Conclusion
Promises are a powerful tool in Node.js development that allow you to write cleaner, more organized, and error-resilient asynchronous code. By understanding the basics of promises, chaining them together, handling errors, and leveraging async/await, you can significantly improve the readability and maintainability of your codebase. As the Node.js ecosystem continues to evolve, mastering the art of promises will empower you to build robust and efficient applications.
Incorporating promises into your Node.js projects is a skill that pays off handsomely. It not only streamlines your code but also enhances collaboration among team members. So, take the time to dive deep into promises and elevate your Node.js development to new heights!
Table of Contents
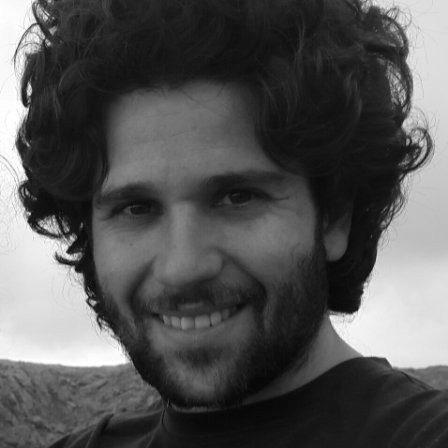
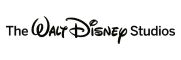