Node.js Q & A
What is the purpose of the querystring module in Node.js?
The querystring module in Node.js provides utilities for parsing and formatting URL query strings. It allows developers to handle query parameters passed in the URL and convert them to JavaScript objects for easier manipulation. The primary purpose of the querystring module is to parse query strings from URLs and serialize JavaScript objects into query strings.
Key functionalities of the querystring module include:
- Parsing Query Strings:
-
-
- The querystring.parse() method parses a URL query string and returns an object representing the key-value pairs of query parameters. It handles URL encoding and decoding automatically.
- Example:
- javascript
-
- Copy code
const querystring = require('querystring'); const queryString = 'name=John&age=30'; const parsedQuery = querystring.parse(queryString); console.log(parsedQuery); // Output: { name: 'John', age: '30' }
- Formatting JavaScript Objects:
-
- The querystring.stringify() method serializes a JavaScript object into a URL query string. It converts key-value pairs of the object into a URL-encoded string suitable for appending to a URL.
- Example:
- javascript
- Copy code
const querystring = require('querystring'); const obj = { name: 'John', age: 30 }; const queryString = querystring.stringify(obj); console.log(queryString); // Output: 'name=John&age=30'
- Customizing Serialization:
-
-
- The querystring.stringify() method supports options to customize serialization behavior, such as specifying delimiters, equals signs, and encoding options.
- Example:
- javascript
-
- Copy code
const querystring = require('querystring'); const obj = { name: 'John', age: 30 }; const queryString = querystring.stringify(obj, ';', ':'); console.log(queryString); // Output: 'name:John;age:30'
- Handling Nested Objects:
-
- The querystring.stringify() method supports nested objects, allowing developers to represent complex data structures in query strings.
- Example:
- javascript
- Copy code
const querystring = require('querystring'); const obj = { user: { name: 'John', age: 30 } }; const queryString = querystring.stringify(obj); console.log(queryString); // Output: 'user[name]=John&user[age]=30'
The querystring module simplifies the process of working with URL query strings in Node.js applications. It is commonly used in web servers, APIs, and middleware to parse incoming requests, extract query parameters, and serialize response data into URL-encoded strings.
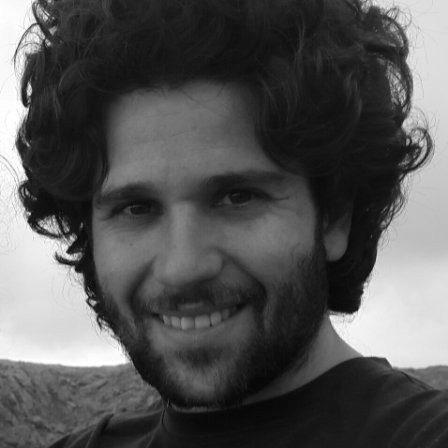
Previously at
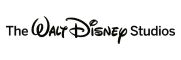
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.