How do you implement rate limiting in Node.js?
Rate limiting in Node.js involves restricting the number of requests that a client can make to a server within a specified time window. Rate limiting helps prevent abuse, protect server resources, and maintain system stability by enforcing usage limits on clients.
There are several approaches to implementing rate limiting in Node.js:
- Middleware: You can implement rate limiting as middleware in your Express.js application using libraries like express-rate-limit or rate-limiter-flexible. These libraries allow you to set up rate limiting policies based on IP address, user, route, or custom criteria, and enforce limits on requests per minute, hour, or any other specified timeframe. Here’s a basic example using the express-rate-limit middleware:
javascript
Copy code
const express = require('express'); const rateLimit = require('express-rate-limit'); const app = express(); // Apply rate limiting middleware const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // limit each IP to 100 requests per windowMs message: 'Too many requests from this IP, please try again later' }); app.use(limiter); // Define your routes app.get('/api/data', (req, res) => { res.send('Data requested'); }); // Start the server app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, the express-rate-limit middleware is applied to all routes of the Express.js application. It limits each IP address to 100 requests per 15-minute window (windowMs). If a client exceeds this limit, they receive a 429 Too Many Requests response with the specified message.
- Distributed Rate Limiting: For distributed applications or APIs deployed across multiple servers or instances, you can implement distributed rate limiting using external services or databases. Services like Redis or Memcached can be used to store request counters and expiry times, allowing all instances of the application to share rate limit information.
- Custom Middleware: If you prefer a custom solution, you can implement rate limiting logic directly in your middleware or route handlers. This involves tracking request counts and timestamps for each client and enforcing rate limits based on predefined thresholds.
Here’s a simplified example of custom rate limiting middleware:
javascript
Copy code
const express = require('express'); const app = express(); const MAX_REQUESTS = 100; const WINDOW_DURATION = 15 * 60 * 1000; // 15 minutes const requestTracker = {}; app.use((req, res, next) => { const { ip } = req; const currentTime = Date.now(); // Initialize request tracker for IP if not exists if (!requestTracker[ip]) { requestTracker[ip] = []; } // Remove expired requests from the tracker requestTracker[ip] = requestTracker[ip].filter((timestamp) => currentTime - timestamp <= WINDOW_DURATION); // Check if the number of requests exceeds the limit if (requestTracker[ip].length >= MAX_REQUESTS) { return res.status(429).send('Too many requests from this IP, please try again later'); } // Add current request timestamp to the tracker requestTracker[ip].push(currentTime); // Proceed to the next middleware next(); }); // Define your routes app.get('/api/data', (req, res) => { res.send('Data requested'); }); // Start the server app.listen(3000, () => { console.log('Server is running on port 3000'); });
This custom middleware tracks the number of requests from each IP address within a specified time window. If a client exceeds the maximum number of requests (MAX_REQUESTS), they receive a 429 Too Many Requests response.
By implementing rate limiting in Node.js, developers can protect their applications from abuse, ensure fair resource allocation, and maintain optimal performance and availability.
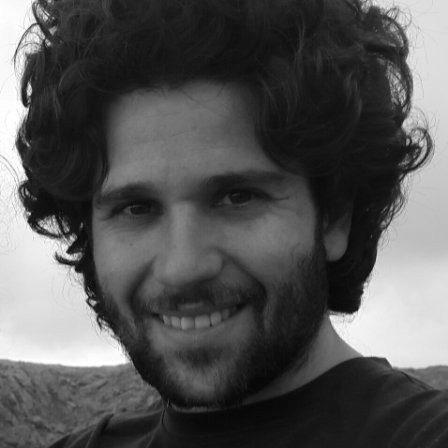
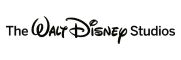