Node.js Q & A
What are the differences between readFile and createReadStream in Node.js?
Both fs.readFile and fs.createReadStream are methods provided by the fs module in Node.js for reading data from files, but they differ in their approach and usage:
- readFile:
-
- fs.readFile reads the entire contents of a file into memory as a buffer or string before invoking the callback function with the data.
- It is suitable for reading small to medium-sized files where the entire contents can fit into memory without causing performance issues.
Example:
javascript
Copy code
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Copy code
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Copy code const fs = require('fs'); fs.readFile('file.txt', 'utf8', (err, data) => { if (err) throw err; console.log(data); });
- createReadStream:
-
- fs.createReadStream creates a readable stream for reading data from a file in chunks or buffers. It reads data asynchronously and emits events as chunks become available.
- It is suitable for reading large files or processing data sequentially without loading the entire file into memory at once.
Example:
javascript
Copy code
const fs = require('fs');
const readStream = fs.createReadStream('file.txt', 'utf8');
readStream.on('data', (chunk) => {
console.log(chunk);
});
const fs = require('fs');
const readStream = fs.createReadStream('file.txt', 'utf8');
readStream.on('data', (chunk) => {
console.log(chunk);
});
const fs = require('fs'); const readStream = fs.createReadStream('file.txt', 'utf8'); readStream.on('data', (chunk) => { console.log(chunk); });
The main differences between readFile and createReadStream are:
- Memory Usage:
-
-
- readFile loads the entire file into memory, which may cause memory issues for large files. In contrast, createReadStream reads data in chunks, consuming less memory overall.
-
- Performance:
-
-
- createReadStream is generally more efficient for large files because it avoids loading the entire file into memory at once. It allows for parallel processing of chunks, leading to better performance.
-
- Usage Pattern:
-
- readFile is suitable for scenarios where you need to read the entire contents of a file into memory for processing or manipulation.
- createReadStream is suitable for scenarios where you want to process data sequentially or stream it to another destination (e.g., HTTP response, network socket).
Choose between readFile and createReadStream based on the size of the file, memory constraints, performance considerations, and the desired usage pattern in your Node.js application.
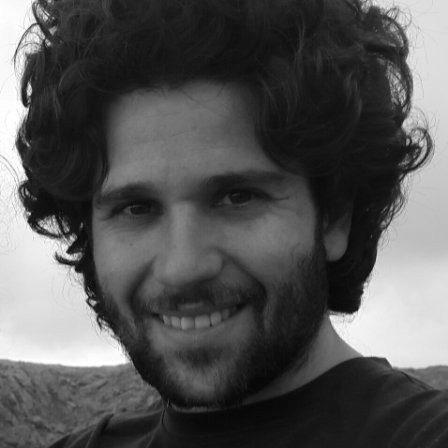
Previously at
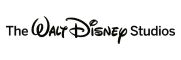
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.