Building Real-Time Dashboards with Node.js and D3.js
In today’s fast-paced digital landscape, data is king. From tracking website traffic to monitoring social media engagement, businesses rely on real-time insights to make informed decisions. However, accessing and visualizing this data in a meaningful way can be challenging. That’s where real-time dashboards come in, providing a dynamic and interactive way to display data as it happens. In this guide, we’ll explore how to build real-time dashboards using Node.js and D3.js, two powerful tools in the developer’s toolkit.
Why Node.js and D3.js?
Node.js, known for its event-driven architecture and non-blocking I/O, is well-suited for building real-time applications. Its ability to handle concurrent connections makes it an ideal choice for streaming data to clients in real-time. On the other hand, D3.js, short for Data-Driven Documents, is a JavaScript library for manipulating documents based on data. With its powerful visualization capabilities, D3.js enables developers to create stunning and interactive charts, graphs, and maps.
Setting Up the Environment
Before diving into the code, let’s set up our development environment. Make sure you have Node.js and npm installed on your machine. You can download them from the official website or use a package manager like Homebrew (for macOS) or Chocolatey (for Windows).
Once you have Node.js installed, create a new directory for your project and navigate to it using the terminal or command prompt. Then, initialize a new Node.js project by running the following command:
npm init -y
Next, install the necessary dependencies:
npm install express socket.io d3
Creating the Server
Now that we have our dependencies installed, let’s create a basic Node.js server using Express.js. Express is a minimalist web framework for Node.js that simplifies the process of building web applications. Create a new file named server.js and add the following code:
const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIo(server); app.use(express.static('public')); io.on('connection', (socket) => { console.log('A client connected'); // Emit dummy data every second setInterval(() => { const data = Math.random() * 100; socket.emit('data', data); }, 1000); }); const PORT = process.env.PORT || 3000; server.listen(PORT, () => { console.log(`Server running on port ${PORT}`); });
In this code, we’re creating a basic Express server and using Socket.IO for real-time communication with clients. We’re emitting dummy data every second to simulate real-time updates.
Building the Dashboard
Now that our server is up and running, let’s create the client-side code for our dashboard. Create a new directory named public and inside it, create an HTML file named index.html:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Real-Time Dashboard</title> <script src="https://d3js.org/d3.v7.min.js"></script> </head> <body> <div id="chart"></div> <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); const svg = d3.select('#chart').append('svg'); const margin = { top: 20, right: 20, bottom: 20, left: 20 }; const width = 600 - margin.left - margin.right; const height = 400 - margin.top - margin.bottom; const x = d3.scaleLinear().domain([0, 10]).range([0, width]); const y = d3.scaleLinear().domain([0, 100]).range([height, 0]); const line = d3.line() .x((d, i) => x(i)) .y((d) => y(d)); svg.attr('width', width + margin.left + margin.right) .attr('height', height + margin.top + margin.bottom) .append('g') .attr('transform', `translate(${margin.left},${margin.top})`); const path = svg.append('path').attr('class', 'line'); socket.on('data', (data) => { // Update the data const dataset = d3.range(10).map(() => data); // Update the line path.datum(dataset) .attr('d', line) .attr('fill', 'none') .attr('stroke', 'steelblue') .attr('stroke-width', 2); }); </script> </body> </html>
In this HTML file, we’re using D3.js to create a simple line chart that updates in real-time as new data is received from the server via Socket.IO.
Running the Application
To run the application, start the server by running the following command in your terminal:
node server.js
Then, open your web browser and navigate to http://localhost:3000. You should see a real-time line chart updating with random data points every second.
Conclusion
In this tutorial, we’ve explored how to build real-time dashboards using Node.js and D3.js. By combining the power of server-side JavaScript with dynamic data visualization, you can create powerful tools for monitoring and analyzing data streams in real-time. Whether you’re tracking website analytics, monitoring IoT devices, or visualizing financial data, real-time dashboards offer a flexible and scalable solution for staying ahead of the curve.
External Links:
Now, armed with this knowledge, unleash the potential of real-time dashboards in your own projects and stay ahead of the competition!
Table of Contents
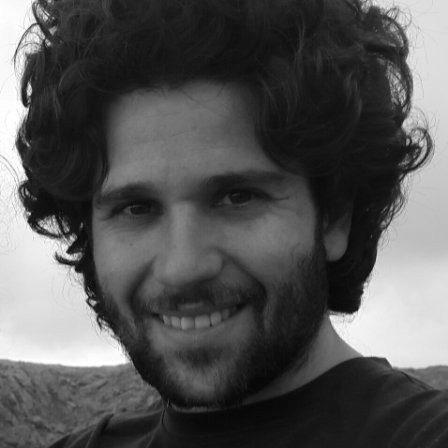
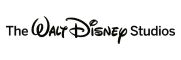