Node.js Q & A
How do you schedule tasks in Node.js?
In Node.js, scheduling tasks involves executing code at specific times, intervals, or according to predefined schedules. There are several ways to schedule tasks in Node.js:
- setTimeout and setInterval:
-
- The setTimeout function executes a callback function after a specified delay, measured in milliseconds.
- The setInterval function executes a callback function repeatedly at a specified interval.
Example:
javascript
Copy code
setTimeout(() => { console.log('Task executed after 2 seconds'); }, 2000); setInterval(() => { console.log('Task executed every 5 seconds'); }, 5000);
- Node.js Timers Module:
-
- Node.js provides additional timer functions like setImmediate, clearImmediate, clearInterval, and clearTimeout for more control over scheduled tasks.
- Cron Jobs:
-
- The node-cron library allows developers to schedule tasks using cron expressions. Cron expressions define the schedule for when a task should execute, such as specific times, intervals, or recurrence patterns.
Example:
javascript
Copy code
const cron = require('node-cron'); cron.schedule('*/5 * * * *', () => { console.log('Task executed every 5 minutes'); });
- Task Queues:
-
- Task queue libraries like bull, bee-queue, or agenda enable developers to create and manage job queues for executing tasks asynchronously or in the background.
- External Services:
-
- Developers can use external scheduling services like AWS Lambda, Google Cloud Functions, or Azure Functions to schedule and execute tasks in a serverless environment.
The choice of scheduling mechanism depends on the specific requirements of the application, such as the frequency of task execution, the complexity of scheduling patterns, and the need for scalability and reliability.
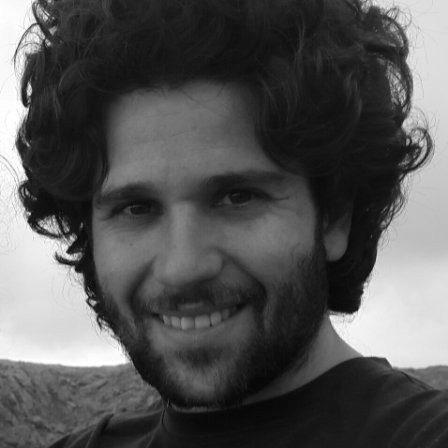
Previously at
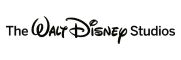
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.