Server-Side Showdown: Analyzing Node.js in Comparison to PHP, Java, Ruby, and Python
When it comes to server-side technologies, the modern developer has an extensive palette from which to choose. They can opt for PHP, a stalwart of web development, or maybe Java, a language renowned for its versatility. Perhaps they might consider Ruby, with its emphasis on clean syntax, or Python, known for its simplicity and readability. But among these, Node.js, a relative newcomer, has been rapidly gaining popularity. It’s widely appreciated for its speed, scalability, and proficiency with real-time applications, which has led to a surge in demand to hire Node.js developers.
In this post, we will delve into a comparative analysis of Node.js with other popular server-side technologies, including PHP, Java, Ruby, and Python. Our aim is not to establish an outright winner, as each of these technologies has its strengths and weaknesses, but rather to help you make informed decisions about the best fit for your particular project needs. This could very well mean determining when to hire Node.js developers for their specific skill set and understanding of this powerful runtime environment.
Node.js
Node.js is a runtime environment that allows JavaScript, a traditionally client-side language, to be used on the server side. It leverages Google’s V8 JavaScript engine and a non-blocking, event-driven architecture, making it highly efficient for handling concurrent requests and I/O operations.
Example: Real-time Application
```JavaScript const io = require('socket.io')(80); io.on('connection', function (socket) { socket.on('ferret', function (name, fn) { fn('woot'); }); }); ```
This is a simple Node.js server using Socket.IO for real-time communication. When a client connects and sends a “ferret” event, the server immediately responds with “woot”.
PHP
PHP is a server-side scripting language designed specifically for web development. It’s been around since 1994, making it one of the oldest languages in the web development space. Its popularity is fueled by its ease of integration with HTML and support for a variety of web servers and databases.
Example: Database Query
```PHP $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } $sql = "SELECT id, firstname, lastname FROM MyGuests"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "id: " . $row["id"]. " - Name: " . $row["firstname"]. " " . $row["lastname"]. "<br>"; } } else { echo "0 results"; } $conn->close(); ```
The PHP code above connects to a database, runs a SELECT SQL query to retrieve data, and displays the results. PHP’s procedural nature makes this a straightforward process.
Java
Java is an object-oriented language widely used in enterprise environments. With the use of frameworks like Spring, Java has robust capabilities for creating complex server-side applications.
Example: Spring MVC Application
```Java @Controller @RequestMapping("/hello") public class HelloController { @RequestMapping(method = RequestMethod.GET) public String printHello(ModelMap model) { model.addAttribute("message", "Hello Spring MVC Framework!"); return "hello"; } } ```
This Java code sets up a simple web application using the Spring MVC framework. When a GET request is made to “/hello”, it responds with a view named “hello” and adds a message to the model.
Ruby
Ruby, coupled with the Rails framework, offers a clean, elegant syntax that many developers find enjoyable to work with. Ruby on Rails follows the MVC (Model, View, Controller) architecture, promoting the organization of code and reuse of principles.
Example: Rails Application
```Ruby class ApplicationController < ActionController::Base def hello render html: "hello, world!" end end ```
This is a basic Ruby on Rails controller. It’s set up to respond to a request with the text “hello, world!”.
Python
Python is a high-level, interpreted language loved for its clean syntax and readability. The Django and Flask frameworks are popular choices for building web applications in Python.
Example: Flask Application
```Python from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' ```
This simple Flask web application responds to a request at the root URL (“/”) with the text “Hello, World!”.
Comparative Analysis
Each of these technologies has specific strengths that make them suitable for different kinds of projects.
- Performance: Node.js, with its non-blocking architecture, can handle more concurrent requests and is generally faster than PHP, Ruby, and Python for I/O intensive operations. Java, with its mature JVM and just-in-time compilation, can outperform Node.js in CPU-intensive tasks.
- Real-time Applications: Node.js, with its event-driven architecture and support for WebSockets, is a strong choice for real-time applications like chat apps and gaming servers.
- Ease of Learning: Python and Ruby are often recommended for beginners due to their clean, readable syntax. Node.js is a good choice for those already familiar with JavaScript. PHP, though somewhat inconsistent, has a low barrier to entry. Java, while powerful, can be more complex to learn.
- Web Development: PHP, with its deep integration with HTML and built-in web development capabilities, remains a solid choice for web development. However, Node.js and Python (with Django or Flask), and Ruby (with Rails), provide powerful, modern alternatives for web development. Java, with the Spring framework, is a robust choice for enterprise-level applications.
- Community and Libraries: All these technologies have strong, active communities and extensive libraries. Node.js, with its package manager npm, boasts the largest number of open-source libraries.
- Scalability: Node.js is often touted for its high scalability due to its event-driven, non-blocking I/O model. It can efficiently handle numerous concurrent connections making it a perfect fit for microservices architectures. Java also offers excellent scalability options, particularly in large and complex enterprise applications. PHP, Python, and Ruby may require additional tools and configurations for similar levels of scalability.
- Concurrency Model: Node.js uses an event-driven, non-blocking I/O model which makes it lightweight and efficient. Java uses a multi-threading model which can be more complex to work with but is excellent for CPU-intensive operations. PHP, Python, and Ruby are fundamentally synchronous, but they offer asynchronous features through various tools and libraries.
- Database Support: PHP has robust support for MySQL, although it can work with other databases as well. Python, with Django and Flask, and Ruby, with Rails, have ORM (Object Relational Mapping) support, which simplifies working with databases. Java, with technologies like JDBC and Hibernate, offers powerful database integration and transaction management features. Node.js supports a wide range of databases and has excellent support for NoSQL databases like MongoDB.
- Integration with Front-End Technologies: Given that Node.js uses JavaScript, it integrates seamlessly with front-end technologies and is a natural fit for full-stack JavaScript development. Java offers JSP and Thymeleaf for front-end development but often gets combined with JavaScript frameworks like Angular and React. PHP can be embedded directly into HTML, providing simple integration. Python and Ruby can integrate with front-end technologies but often require separate templates or front-end frameworks.
- Long-term Viability: Java and PHP, given their long history and widespread usage in the industry, have proven their long-term viability. Python, with its rising popularity in machine learning and data science, and its simplicity, has a strong future. Ruby, though it experienced a slight dip, maintains a dedicated community and continues to be used in new projects. Node.js, despite being relatively new, has gained substantial traction, is used by many large companies, and shows no sign of slowing down.
While comparing these technologies, it’s crucial to understand that there’s no absolute “best” choice that fits all use-cases. The optimal technology will largely depend on your specific project requirements, team expertise, and the problem you are trying to solve. Consider these factors carefully to make an informed decision.
Conclusion
As we conclude this comparative analysis, remember that all these technologies are evolving, and the landscape may change in the future. It’s beneficial to always do a fresh round of research when starting a new project to ensure that you’re using the best tools for your specific needs. In fact, many businesses opt to hire Node.js developers because of their expertise in handling the flexible and efficient framework. Their specialized knowledge can make a significant difference in the performance and success of your project.
Table of Contents
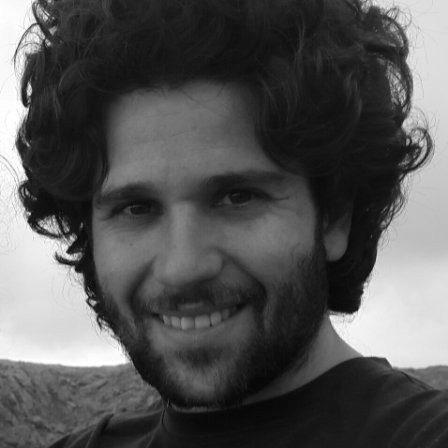
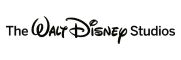