Testing Node.js Applications with Mocha and Chai
In the ever-evolving landscape of software development, ensuring the reliability and stability of your Node.js applications is paramount. One of the most effective ways to achieve this is through comprehensive testing. Enter Mocha and Chai, a dynamic duo that empowers developers to write elegant, expressive, and effective tests for their Node.js applications.
Understanding Mocha and Chai
Mocha: The Test Framework
Mocha is a feature-rich JavaScript test framework that runs on Node.js and in the browser, making it highly versatile. With its intuitive interface, Mocha provides a flexible and powerful testing environment, allowing developers to write asynchronous tests with ease. Its suite structure enables organized testing, while its robust reporting capabilities provide clear insights into test results.
Chai: The Assertion Library
Complementing Mocha is Chai, a BDD/TDD assertion library for Node.js and browsers. Chai’s expressive syntax enables developers to write human-readable assertions, enhancing the clarity and maintainability of their tests. Whether you prefer the should, expect, or assert style, Chai accommodates diverse testing styles, empowering developers to craft tests that align with their preferences and project requirements.
Getting Started with Mocha and Chai
Installation
Getting started with Mocha and Chai is straightforward. Begin by installing them via npm:
npm install --save-dev mocha chai
Writing Tests
With Mocha and Chai installed, you’re ready to start writing tests. Create a directory for your tests and begin by defining your test suite using Mocha’s describe function. Within each suite, use it to specify individual test cases. Then, leverage Chai’s assertion methods within your tests to validate expected outcomes.
const chai = require('chai'); const { expect } = chai; describe('Array', () => { describe('#indexOf()', () => { it('should return -1 when the value is not present', () => { expect([1, 2, 3].indexOf(4)).to.equal(-1); }); }); });
Running Tests
Executing your tests is as simple as invoking the mocha command in your terminal, specifying the directory where your tests reside. Mocha will then run your tests and provide detailed output, including the status of each test case and any failures encountered.
mocha test
Examples of Mocha and Chai in Action
Example 1: Testing a Basic Math Utility
Consider a simple utility function for adding two numbers. Let’s write tests for this function using Mocha and Chai:
const { expect } = require('chai'); const { add } = require('../math'); describe('Math Utility', () => { describe('add', () => { it('should return the sum of two numbers', () => { expect(add(2, 3)).to.equal(5); }); it('should handle negative numbers correctly', () => { expect(add(-1, 1)).to.equal(0); }); }); });
Example 2: Testing an API Endpoint
Imagine you have an API endpoint for retrieving user data. Let’s write tests for this endpoint using Mocha and Chai alongside a testing library like supertest:
const request = require('supertest'); const app = require('../app'); describe('User API', () => { describe('GET /users/:id', () => { it('should return user data for a valid ID', async () => { const res = await request(app).get('/users/1'); expect(res.status).to.equal(200); expect(res.body).to.have.property('name', 'John Doe'); }); it('should return 404 for an invalid ID', async () => { const res = await request(app).get('/users/999'); expect(res.status).to.equal(404); }); }); });
Conclusion
Mocha and Chai offer a potent combination for testing Node.js applications, providing developers with the tools needed to ensure the reliability and correctness of their code. By following best practices and leveraging the expressive syntax of Mocha and Chai, developers can streamline their testing workflow and deliver robust, high-quality software.
Now that you’ve delved into the world of testing with Mocha and Chai, why not explore further? Dive deeper into their documentation, experiment with different testing scenarios, and witness firsthand the transformative impact they can have on your Node.js projects.
External Resources:
Happy testing!
Table of Contents
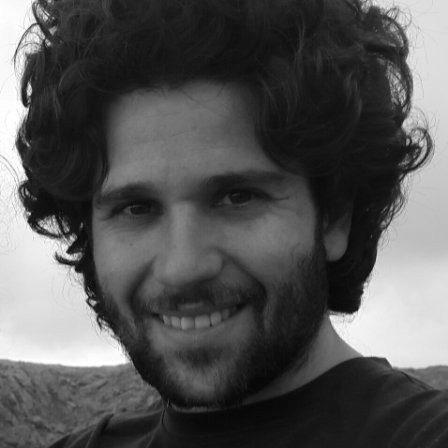
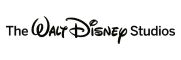