Building Web Scrapers with Puppeteer and Node.js
In today’s data-driven world, extracting information from websites has become an essential task for various applications, from market research to competitive analysis. Web scraping, the process of automating data extraction from websites, enables businesses and individuals to gather valuable insights. One of the most popular tools for web scraping is Puppeteer, a Node.js library developed by Google. In this guide, we will delve into the world of web scraping using Puppeteer and Node.js, exploring its capabilities, best practices, and providing practical examples to help you build effective web scrapers.
Table of Contents
1. Understanding Web Scraping and Puppeteer:
Web scraping is the process of automatically extracting data from websites. It involves fetching web pages, parsing their content, and extracting relevant information for further analysis. This technique has a wide range of applications, including data mining, price monitoring, content aggregation, and more.
1.1. Introducing Puppeteer:
Puppeteer is a powerful Node.js library developed by Google that provides a high-level API to control headless Chrome or Chromium browsers. Headless browsers are essentially web browsers without a graphical user interface, making them ideal for automated tasks like web scraping. Puppeteer enables you to interact with web pages, simulate user interactions, navigate through websites, and extract data efficiently.
2. Setting Up Your Environment:
Before you start building web scrapers with Puppeteer and Node.js, you need to set up your development environment.
2.1. Installing Node.js and NPM:
Node.js is a JavaScript runtime that allows you to execute JavaScript code on the server-side. NPM (Node Package Manager) is a package manager for Node.js that helps you install and manage libraries and dependencies.
To install Node.js and NPM, visit the official Node.js website (https://nodejs.org/) and follow the installation instructions for your operating system.
2.2. Initializing a Node.js Project:
Once Node.js and NPM are installed, you can create a new directory for your web scraping project and navigate to it using the terminal. Then, run the following command to initialize a new Node.js project:
bash npm init -y
This command creates a package.json file in your project directory, which will store information about your project and its dependencies.
2.3. Installing Puppeteer:
With your Node.js project set up, you can now install Puppeteer. Open the terminal and run the following command:
bash npm install puppeteer
Puppeteer will be downloaded and added as a dependency to your project.
3. Basic Web Scraping with Puppeteer:
Now that your environment is ready, let’s start with some basic web scraping using Puppeteer.
3.1. Navigating to a Web Page:
To begin, you’ll need to launch a new browser instance with Puppeteer and navigate to a web page. Here’s a code snippet that demonstrates how to do this:
javascript const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); // Continue with your scraping logic await browser.close(); })();
In this example, we use the puppeteer.launch() function to launch a new browser instance and browser.newPage() to create a new page within that instance. The page.goto() function navigates to the specified URL.
3.2. Selecting Elements:
Once you’re on a web page, you’ll likely want to extract specific elements such as headings, paragraphs, images, or tables. Puppeteer provides various methods to select elements using CSS selectors. Here’s an example of how to select and extract text from all the headings on a page:
javascript const headings = await page.$$eval('h1, h2, h3, h4, h5, h6', (elements) => { return elements.map(element => element.textContent); }); console.log(headings);
The $$eval() function allows you to evaluate a function in the context of the page and retrieve data from selected elements.
3.3. Extracting Text and Attributes:
To extract text or attributes from a single element, you can use the page.evaluate() function. Here’s an example of extracting the text from a specific element:
javascript const text = await page.evaluate(() => { const element = document.querySelector('#target-element'); return element.textContent; }); console.log(text);
For extracting attributes, you can modify the code as follows:
javascript const href = await page.evaluate(() => { const link = document.querySelector('#target-link'); return link.getAttribute('href'); }); console.log(href);
3.4. Taking Screenshots:
Puppeteer also allows you to capture screenshots of web pages. This can be useful for visual verification or creating previews of scraped content. Here’s how you can take a screenshot using Puppeteer:
javascript await page.screenshot({ path: 'screenshot.png' });
You can customize the screenshot by providing various options like fullPage, clip, and type.
4. Advanced Web Scraping Techniques:
As you become more comfortable with the basics, you can explore advanced techniques for handling complex scenarios during web scraping.
4.1. Handling Pagination:
Scraping paginated content involves navigating through multiple pages to extract data. This often requires iterating over pages and extracting data from each one. Here’s a simplified example of how you might handle pagination:
javascript const totalPages = 5; for (let pageIdx = 1; pageIdx <= totalPages; pageIdx++) { const pageUrl = `https://example.com/page/${pageIdx}`; await page.goto(pageUrl); // Extract and process data from the current page }
4.2. Interacting with Forms:
Some websites require user interaction, such as submitting search forms. Puppeteer enables you to fill out and submit forms programmatically:
javascript await page.type('#search-input', 'your search query'); await page.click('#search-button'); await page.waitForNavigation();
In this example, we use the page.type() function to input text into a form field, page.click() to click the search button, and page.waitForNavigation() to wait for the page to navigate to the search results.
4.3. Waiting for Asynchronous Content:
Modern websites often load content asynchronously through AJAX requests or other JavaScript techniques. Puppeteer provides functions to wait for specific events before proceeding:
javascript await page.waitForSelector('.dynamic-content');
The waitForSelector() function waits until an element matching the specified selector is present on the page.
4.4. Dealing with Captchas:
Some websites use captchas to prevent automated scraping. While solving captchas programmatically can be challenging, you can employ services like AntiCaptcha or reCAPTCHA solving services to overcome this hurdle.
5. Best Practices for Ethical Web Scraping:
When building web scrapers, it’s crucial to follow ethical practices to avoid disrupting websites or violating terms of use.
5.1. Respect Robots.txt:
The robots.txt file on a website specifies which pages are off-limits for web crawlers. It’s essential to review this file and ensure your scraper follows the guidelines.
5.2. Use Headless Browsing:
Puppeteer’s headless mode allows you to run browsers without a graphical user interface, making your scraping less resource-intensive and more discreet.
5.3. Implement Rate Limiting:
Sending too many requests in a short period can overload a server. Implement rate limiting in your scraper to avoid causing strain on the target website’s infrastructure.
5.4. Mimic Human Behavior:
Configure your scraper to behave like a human user by adding delays between requests, varying user-agent headers, and simulating mouse movements.
6. Building a Real-World Web Scraper:
Let’s walk through the process of building a real-world web scraper using Puppeteer and Node.js.
6.1. Defining the Scraping Target:
For our example, let’s create a scraper that extracts product information from an online shopping website. The target website has a list of products, each with a name, price, and description.
6.2. Designing the Scraper Workflow:
Before writing code, it’s essential to plan the scraper’s workflow. Here’s a high-level overview:
- Navigate to the product listing page.
- Extract URLs of individual product pages.
- For each product page URL:
- Navigate to the page.
- Extract product details (name, price, description).
- Store the data.
- Repeat the process for multiple pages (pagination).
6.3. Writing the Scraper Code:
Here’s a simplified version of the scraper code based on the workflow we designed:
javascript const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); const productListingUrl = 'https://example-shopping-site.com/products'; await page.goto(productListingUrl); const productPageUrls = await page.$$eval('.product-link', links => { return links.map(link => link.href); }); const scrapedData = []; for (const productUrl of productPageUrls) { await page.goto(productUrl); const productDetails = await page.evaluate(() => { const name = document.querySelector('.product-name').textContent; const price = document.querySelector('.product-price').textContent; const description = document.querySelector('.product-description').textContent; return { name, price, description }; }); scrapedData.push(productDetails); } console.log(scrapedData); await browser.close(); })();
In this example, we first navigate to the product listing page and extract the URLs of individual product pages. Then, for each product page URL, we navigate to the page and extract the product details using page.evaluate(). The scraped data is stored in the scrapedData array.
7. Handling Errors and Edge Cases:
During web scraping, you’ll encounter various errors and edge cases that need to be handled gracefully.
7.1. Dealing with Page Crashes:
Web pages might crash due to JavaScript errors or other issues. To handle this, wrap your scraping logic in a try-catch block and handle page crashes by reopening the page:
javascript for (const productUrl of productPageUrls) { try { await page.goto(productUrl); // Scraping logic } catch (error) { console.error(`Error scraping ${productUrl}: ${error.message}`); await page.goto(productUrl); // Reopen the page } }
7.2. Handling Dynamic Websites:
Some websites load content dynamically using JavaScript. You might need to use the page.waitForSelector() function to ensure the required elements are present before extracting data.
7.3. Debugging and Logging:
For effective debugging, use console.log() statements strategically throughout your code. Additionally, Puppeteer provides the page.screenshot() function to capture screenshots during different stages of scraping, aiding in identifying issues.
8. Storing and Using Scraped Data:
After scraping data, you’ll likely want to store it for further analysis or integration with other applications.
8.1. Data Storage Options:
You can store scraped data in various formats such as JSON, CSV, or databases like MongoDB or MySQL, depending on your requirements.
8.2. Data Transformation and Analysis:
Once data is scraped and stored, you can transform and analyze it using tools like Python’s pandas library or data visualization libraries like matplotlib or D3.js.
8.3. Integrating with Other Applications:
Scraped data can be integrated into business intelligence tools, dashboards, or APIs to provide valuable insights to stakeholders.
9. Deploying and Scaling Scraping Jobs:
When it comes to deploying and scaling your scrapers, there are a few considerations to keep in mind.
9.1. Running Scrapers on Servers:
For continuous scraping, consider deploying your scraper on a cloud server using platforms like AWS, Google Cloud, or DigitalOcean. Use tools like pm2 or Docker for process management and containerization.
9.2. Using Cloud Services:
Cloud-based scraping services like ScrapingHub or Apify offer managed infrastructure, handling the technical aspects of running scrapers at scale.
9.3. Scaling Considerations:
If you plan to scrape a large volume of data, ensure your scraper is designed to handle concurrency, retries, and distributed processing.
Conclusion
Web scraping using Puppeteer and Node.js opens up a world of possibilities for extracting valuable data from websites. By understanding the basics, mastering advanced techniques, and following ethical best practices, you can build powerful and efficient web scrapers tailored to your specific needs. Whether you’re gathering market insights, monitoring competitors, or conducting research, Puppeteer empowers you to automate data extraction with precision and reliability. Start your web scraping journey today and unlock a wealth of information at your fingertips.
Table of Contents
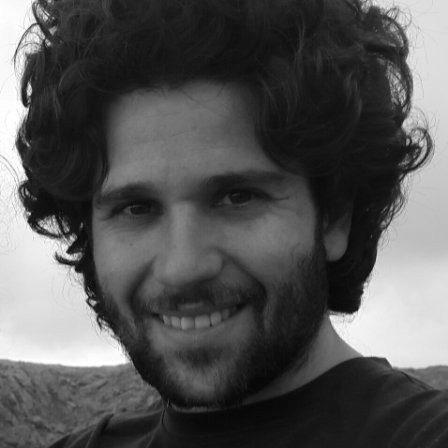
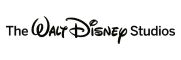