How do you handle form validation in Node.js?
WebSockets in Node.js are a communication protocol that enables bidirectional, full-duplex communication between a client and a server over a single, long-lived connection. Unlike traditional HTTP requests, which are stateless and one-directional (client to server), WebSockets allow real-time, interactive communication between clients and servers, making them ideal for applications that require low-latency, high-frequency data exchange, such as chat applications, online gaming, collaborative editing, and real-time analytics.
The key features and benefits of WebSockets in Node.js include:
- Full-Duplex Communication: WebSockets allow both the client and server to send and receive messages simultaneously over a single connection, enabling real-time, bidirectional communication without the overhead of HTTP request-response cycles.
- Low Latency: By maintaining a persistent connection between the client and server, WebSockets eliminate the need for repeated connection establishment and teardown, reducing latency and improving responsiveness, especially for applications that require frequent data updates.
- Efficient Resource Utilization: WebSockets are lightweight and efficient, requiring minimal overhead compared to traditional HTTP connections. This makes them suitable for handling large numbers of concurrent connections and scaling horizontally to meet increasing demand.
- Cross-Platform Support: WebSockets are supported by most modern web browsers and can be used with various programming languages and frameworks, making them a versatile choice for building real-time web applications.
In Node.js, you can implement WebSockets using libraries like Socket.IO, ws (WebSocket implementation for Node.js), or uWebSockets.js. These libraries provide APIs for creating WebSocket servers, handling WebSocket connections, sending and receiving messages, and managing WebSocket events.
Here’s a basic example of creating a WebSocket server in Node.js using the Socket.IO library:
javascript Copy code const http = require('http'); const socketIo = require('socket.io'); const server = http.createServer(); const io = socketIo(server); io.on('connection', (socket) => { console.log('A client connected'); socket.on('message', (data) => { console.log('Received message:', data); // Broadcast the message to all connected clients io.emit('message', data); }); socket.on('disconnect', () => { console.log('A client disconnected'); }); }); server.listen(3000, () => { console.log('WebSocket server is running on port 3000'); });
In this example, the server creates a WebSocket server and listens for incoming WebSocket connections. When a client connects (`connection` event), the server logs the connection and sets up event listeners for receiving messages (`message` event) and handling disconnections (`disconnect` event). The server then broadcasts any received messages to all connected clients using the `io.emit()` method.
On the client side, you can establish a WebSocket connection using JavaScript in the browser:
```javascript const socket = io('http://localhost:3000'); socket.on('connect', () => { console.log('Connected to server'); }); socket.on('message', (data) => { console.log('Received message:', data); }); socket.on('disconnect', () => { console.log('Disconnected from server'); }); // Send a message to the server socket.emit('message', 'Hello, server!');
This client code establishes a connection to the WebSocket server running on localhost:3000, listens for the connect event to confirm the connection, and defines event handlers for receiving messages (message event) and handling disconnections (disconnect event). It also sends a message to the server using the emit() method.
By using WebSockets in Node.js, developers can build real-time, interactive web applications that deliver seamless user experiences with low latency and high responsiveness.
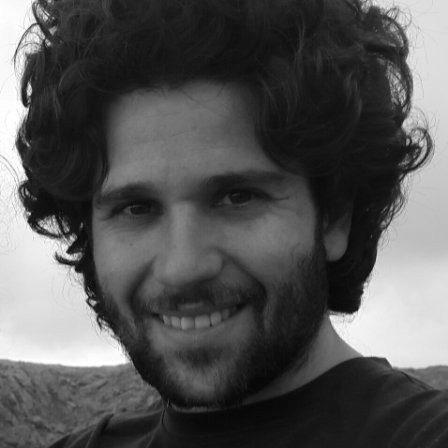
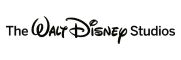