PhoneGap and Background Processing: Multitasking in Hybrid Apps
In the ever-evolving world of mobile app development, striking the right balance between functionality and performance is a constant challenge. Hybrid apps, which combine web technologies with native capabilities, have gained popularity for their ability to bridge this gap. One crucial aspect of enhancing hybrid app performance is background processing, allowing apps to perform tasks even when they are not in the foreground. In this guide, we will delve into PhoneGap and how it can be used for background processing in hybrid apps.
1. Understanding Hybrid Apps and PhoneGap
1.1. What are Hybrid Apps?
Hybrid apps are a blend of web applications and native mobile apps. They are built using web technologies such as HTML, CSS, and JavaScript, and then wrapped in a native container to make them accessible as standalone mobile applications. This approach offers the advantage of code reusability across different platforms, making development more efficient.
1.2. Introducing PhoneGap
PhoneGap, now known as Apache Cordova, is an open-source platform that simplifies the development of hybrid mobile apps. It provides a set of APIs (Application Programming Interfaces) that allow developers to access native device features like the camera, GPS, contacts, and more using web technologies.
2. The Need for Background Processing
Mobile apps often require background processing to provide a seamless user experience. Common use cases for background processing include:
- Notifications: Apps need to handle incoming notifications, whether they are push notifications, messages, or updates.
- Data Sync: Background processing is crucial for syncing app data with servers or other devices, ensuring that the latest information is available to users.
- Location Tracking: Apps that require real-time location tracking or geofencing functionality need background processing to run location-related tasks.
- Media Playback: Apps playing audio or video should continue to function even when the app is not in the foreground.
Now, let’s explore how PhoneGap allows you to implement background processing for these and other use cases.
3. Multitasking Techniques in PhoneGap
PhoneGap provides several ways to implement background processing and multitasking in hybrid apps. Here are some of the most common techniques:
3.1. Using Web Workers
Web Workers are JavaScript scripts that run in the background, separate from the main app thread. They are ideal for CPU-intensive tasks that could slow down the user interface if executed in the main thread. PhoneGap allows you to create and use Web Workers just like you would in a web application.
javascript // Creating a Web Worker const myWorker = new Worker('worker.js'); // Listening for messages from the worker myWorker.onmessage = function(event) { console.log(`Worker said: ${event.data}`); }; // Sending a message to the worker myWorker.postMessage('Hello, worker!');
3.2. Background Geolocation
For apps that require continuous location tracking, PhoneGap offers plugins like the “cordova-plugin-background-geolocation.” This plugin allows you to track the device’s location even when the app is in the background or the device is locked.
javascript // Configuring background geolocation const bgGeo = window.BackgroundGeolocation; bgGeo.configure({ desiredAccuracy: 10, stationaryRadius: 20, distanceFilter: 30, notificationTitle: 'Background location tracking', notificationText: 'ENABLED', debug: true, interval: 60000, }); // Starting background geolocation bgGeo.start();
3.3. Local Notifications
To send local notifications from your app, PhoneGap provides plugins like the “cordova-plugin-local-notification.” These notifications can be scheduled to appear at a specific time or triggered by specific events, even when the app is in the background.
javascript // Scheduling a local notification cordova.plugins.notification.local.schedule({ title: 'Reminder', text: 'Don't forget your meeting at 2 PM!', at: new Date(new Date().getTime() + 60 * 1000) // One minute from now });
3.4. Background Fetch
PhoneGap supports background fetching, which allows your app to periodically fetch data from the internet, even when it’s in the background. This is useful for keeping app content up-to-date.
javascript // Registering a background fetch task cordova.plugins.backgroundFetch.configure( function(taskId) { // Perform background fetch here console.log('Background fetch task started:', taskId); // Finish the task cordova.plugins.backgroundFetch.finish(taskId); }, function(error) { console.error('Background fetch error:', error); }, { minimumFetchInterval: 15 // Minimum interval in minutes } );
4. Best Practices for Background Processing
While implementing background processing in your PhoneGap app, it’s essential to follow best practices to ensure efficiency and maintain a positive user experience. Here are some tips:
4.1. Optimize Resource Usage
Background processing can consume significant resources like battery and memory. Be mindful of these resources and optimize your code to minimize their usage. Avoid unnecessary background tasks.
4.2. Handle Errors Gracefully
Ensure that your app gracefully handles errors that may occur during background processing. Logging errors and providing user-friendly error messages can help troubleshoot issues.
4.3. Respect User Privacy
Always obtain explicit user consent for background processing tasks that involve sensitive data or device features like location tracking. Respect user preferences and privacy settings.
4.4. Test Thoroughly
Thoroughly test your background processing functionality on various devices and operating systems to ensure compatibility and reliability.
Conclusion
Background processing is a crucial aspect of mobile app development, and PhoneGap provides the tools and plugins necessary to implement multitasking in hybrid apps effectively. Whether you need to track the device’s location, schedule notifications, or perform data synchronization, PhoneGap’s features empower you to create responsive and feature-rich apps.
As you explore background processing in PhoneGap, remember to prioritize resource optimization, error handling, and user privacy. With careful implementation and adherence to best practices, you can create hybrid apps that excel in multitasking scenarios, providing users with a seamless and enjoyable experience.
Start incorporating background processing into your PhoneGap projects today, and unlock a new level of functionality and responsiveness in your hybrid mobile apps. Happy coding!
With this comprehensive guide, you’ve learned how PhoneGap can be a powerful tool for implementing background processing and multitasking in your hybrid apps. From Web Workers to location tracking and local notifications, PhoneGap provides a range of options to cater to your app’s specific needs. Remember to follow best practices to ensure the efficiency and reliability of your background processing tasks. Now, you’re equipped to create responsive and feature-rich hybrid mobile apps that deliver an exceptional user experience.
Table of Contents
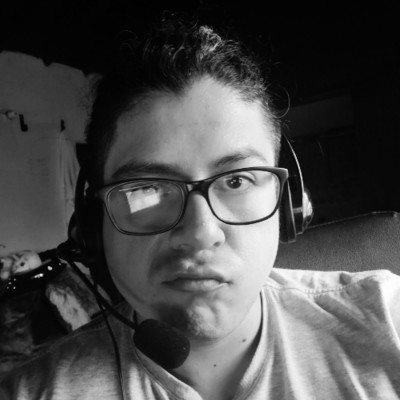
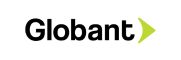