PhoneGap and Background Sync: Updating Data in the Background
In today’s fast-paced digital world, mobile applications need to provide seamless user experiences, even when the device is offline or has limited connectivity. Imagine you’re using a mobile app to manage tasks, and you suddenly lose your internet connection. You should still be able to create, edit, and delete tasks without any hiccups. This is where background sync comes into play.
In this blog post, we will explore how PhoneGap, a popular open-source framework for building cross-platform mobile apps using web technologies, enables developers to implement background sync for updating data. We’ll dive into the concepts, best practices, and provide code samples to help you get started.
1. Understanding Background Sync
Before we delve into PhoneGap and its Background Sync capabilities, it’s crucial to grasp the concept of background synchronization.
Background synchronization allows a mobile application to update data even when it’s not actively running in the foreground. This feature is essential for apps that rely on real-time data or need to sync user-generated content. Examples include messaging apps, task management apps, and collaborative document editors.
Imagine you’re using a messaging app, and someone sends you a message while you’re offline. With background sync, that message can be downloaded and displayed in the app as soon as your device regains connectivity, without requiring you to manually refresh the app.
2. Why Background Sync Matters
Improved User Experience: Background sync ensures that users can interact with your app seamlessly, regardless of their network connection. This leads to higher user satisfaction and retention.
- Data Consistency: It helps maintain data consistency across devices and ensures that users always have access to the latest information.
- Reduced Data Loss: By periodically syncing data in the background, you can minimize data loss in case of app crashes or device failures.
Now that we understand the importance of background sync, let’s see how PhoneGap can help us implement this feature in our mobile applications.
3. PhoneGap: A Quick Overview
PhoneGap, now known as Apache Cordova, is an open-source mobile development framework that allows you to build cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. It provides a bridge between web and native code, enabling you to access device features and APIs.
Here are some key benefits of using PhoneGap for mobile app development:
3.1. Cross-Platform Compatibility
PhoneGap allows you to write your app’s code once and run it on multiple platforms, including iOS, Android, and Windows. This significantly reduces development time and effort.
3.2. Access to Native Features
You can access native device features like the camera, GPS, and sensors using JavaScript, thanks to PhoneGap’s plugin system.
3.3. Large Community and Plugin Ecosystem
PhoneGap has a thriving community and a vast ecosystem of plugins, which means you can easily extend your app’s functionality by adding pre-built plugins.
3.4. Web-Based Development
Developers familiar with web technologies can leverage their existing skills to create mobile apps with PhoneGap.
Now that we have a basic understanding of PhoneGap, let’s explore how it can help us implement background sync.
4. Implementing Background Sync with PhoneGap
PhoneGap provides several plugins and tools that simplify the implementation of background sync in your mobile applications. One of the most popular plugins for this purpose is the Background Sync plugin.
4.1. Setting Up PhoneGap and the Background Sync Plugin
To get started, make sure you have PhoneGap installed on your development machine. You can install it using npm:
bash npm install -g phonegap
Next, create a new PhoneGap project:
bash phonegap create my-app cd my-app
Now, add the Background Sync plugin to your project:
bash phonegap plugin add cordova-plugin-background-sync
With the plugin installed, you can start implementing background sync in your app.
4.2. Creating a Background Sync Task
The first step in implementing background sync is to define a task that specifies what data needs to be synchronized and how often the synchronization should occur. You can do this by adding the following code to your JavaScript:
javascript const syncTask = { id: 'my-sync-task', options: { delay: 60000, // Delay in milliseconds before the sync task runs (60 seconds in this example). periodic: true, // Set to true for periodic sync. headsUpDisplay: true, // Show a notification when sync is in progress. networkTypes: [Connection.NONE] // Specify the network types on which sync should occur. }, callbackFn: function() { // Your synchronization logic goes here. } }; cordova.plugins.backgroundSync.register(syncTask);
In this code snippet:
- id is a unique identifier for your sync task.
- delay specifies the delay in milliseconds before the sync task runs. In this example, the sync task will run every 60 seconds.
- periodic is set to true to indicate that this is a periodic sync task.
- headsUpDisplay is set to true to display a notification when sync is in progress.
- networkTypes specifies the types of network connections on which the sync should occur. In this case, it’s set to Connection.NONE, which means the sync will occur when the device has no network connectivity.
5. Handling Synchronization Logic
Inside the callbackFn function, you can implement the logic to synchronize your data. This may involve making API requests, updating local databases, or any other data-related tasks.
Here’s an example of how you might synchronize data from a remote server:
javascript callbackFn: function() { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Update your app's data with the fetched data. }) .catch(error => { console.error('Sync failed:', error); }); }
In this example, we use the fetch API to retrieve data from a remote server and update the app’s data with the fetched information. If the synchronization fails, we log an error message.
6. Starting and Stopping Background Sync
Once you’ve defined your sync task, you can start and stop background sync as needed. Here’s how you can start the sync task:
javascript cordova.plugins.backgroundSync.start('my-sync-task');
And here’s how you can stop it:
javascript cordova.plugins.backgroundSync.stop('my-sync-task');
You can trigger these actions based on user interactions, app events, or a predefined schedule.
7. Handling Sync Errors and Retries
Background sync is not always guaranteed to succeed due to network issues or other factors. PhoneGap’s Background Sync plugin provides a mechanism for handling errors and retries.
You can specify the number of retries and the delay between retries in your sync task options:
javascript const syncTask = { // ... other options options: { retries: 3, // Number of retries before giving up. retryInterval: 30000 // Delay between retries in milliseconds (30 seconds in this example). // ... other options }, // ... callback function };
With these options set, the sync task will automatically retry a specified number of times if it encounters an error.
8. Testing Background Sync
Testing background sync can be a bit tricky, as it requires you to simulate different network conditions and test the behavior of your app when it’s running in the background. Here are some tips for testing background sync in your PhoneGap app:
- Use Emulators: Emulators or simulators allow you to simulate different network conditions and test your app’s behavior in the background. Both Android and iOS emulators provide tools for adjusting network settings.
- Debugging: Use debugging tools to monitor the behavior of your app when it’s running in the background. You can use the Chrome DevTools for Android or Xcode for iOS.
- Test on Real Devices: Ultimately, you should test your app on real devices to ensure that background sync works as expected in real-world scenarios.
9. Best Practices for Background Sync with PhoneGap
Implementing background sync effectively requires attention to detail and adherence to best practices. Here are some tips to ensure a smooth experience for your app users:
9.1. Minimize Data Transfer
Sync only the data that is essential for the app’s functionality. Minimizing data transfer reduces the load on the network and conserves the device’s battery.
9.2. Handle Network Failures Gracefully
Your app should gracefully handle network failures and retries. Provide clear error messages to the user and implement retry mechanisms as needed.
9.3. Optimize Battery Usage
Background sync can consume significant battery power if not optimized. Ensure that your sync tasks run efficiently and avoid unnecessary background processing.
9.4. Respect User Preferences
Allow users to control background sync settings. Provide options to enable or disable background sync, specify sync intervals, and manage data usage.
9.5. Test Thoroughly
Test your app under various network conditions, including poor connectivity and airplane mode. Ensure that background sync works reliably and doesn’t negatively impact the user experience.
Conclusion
Background sync is a crucial feature for modern mobile applications, ensuring that users can access up-to-date data even when their devices are offline. With PhoneGap and its Background Sync plugin, developers have a powerful toolset to implement this functionality seamlessly.
In this blog post, we’ve explored the concept of background sync, introduced PhoneGap as a versatile mobile development framework, and provided a step-by-step guide to implementing background sync using PhoneGap’s Background Sync plugin. We’ve also discussed best practices to follow when implementing background sync in your mobile app.
By leveraging the capabilities of PhoneGap and following best practices, you can create mobile applications that offer a superior user experience, even in challenging network conditions. So, go ahead, explore background sync, and enhance your app’s functionality for a world where connectivity is always on the move. Happy coding!
Table of Contents
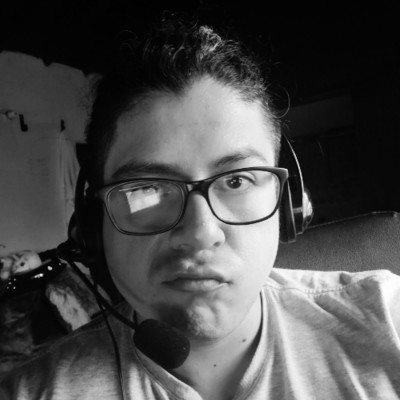
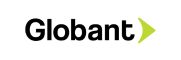