PhoneGap and Firebase Integration: Real-Time Data for Hybrid Apps
In the ever-evolving world of mobile app development, creating applications that provide real-time data and engagement is crucial. Hybrid apps, which combine the best of both native and web apps, have gained immense popularity. One of the key technologies that can supercharge hybrid app development is Firebase, a robust cloud-based platform offered by Google. In this comprehensive guide, we will explore how to integrate PhoneGap with Firebase to enable real-time data capabilities in your hybrid apps.
1. Understanding PhoneGap and Firebase
1.1 PhoneGap
PhoneGap, now known as Apache Cordova, is an open-source mobile development framework that allows developers to build cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. PhoneGap bridges the gap between web development and native mobile app development by providing a set of APIs that give web apps access to native device features.
Key features of PhoneGap include:
- Cross-platform compatibility: Develop once, deploy on multiple platforms (iOS, Android, Windows, etc.).
- Access to native device capabilities: Camera, GPS, accelerometer, and more.
A large community and plugin ecosystem for extended functionality.
Support for popular web development frameworks like Angular, React, and Vue.js.
1.2 Firebase
Firebase is a comprehensive platform for mobile and web app development that offers a wide range of tools and services to help developers build high-quality applications. Some of the core features of Firebase include:
- Real-time Database: A NoSQL cloud database that enables real-time data synchronization across devices.
- Authentication: Secure user authentication with email/password, social identity providers, and more.
- Cloud Firestore: A scalable and flexible NoSQL cloud database with real-time data syncing.
- Cloud Storage: Store and serve user-generated content, such as images and videos.
- Hosting: Web hosting with SSL support and continuous integration.
- Cloud Functions: Execute serverless functions in response to events.
By integrating Firebase with PhoneGap, you can harness the power of these Firebase features to create hybrid apps that provide real-time updates, secure user authentication, and cloud-based data storage.
2. Setting Up Your Development Environment
Before diving into PhoneGap and Firebase integration, make sure you have the necessary tools and environments set up on your development machine. Here’s what you’ll need:
- Node.js and npm: PhoneGap and Firebase both rely on Node.js and npm (Node Package Manager). Install them if you haven’t already.
To check if Node.js is installed, open your terminal and run:
bash node -v
To check if npm is installed, run:
bash npm -v
If not installed, download and install Node.js from the official website: Node.js.
- PhoneGap CLI: Install the PhoneGap Command-Line Interface globally by running:
bash npm install -g phonegap
- Firebase Account: You’ll need a Google account to access Firebase. If you don’t have one, sign up for free at Firebase.
With your development environment set up, you’re ready to create a PhoneGap project and integrate Firebase.
3. Creating a PhoneGap Project
Let’s start by creating a new PhoneGap project. Open your terminal and navigate to the directory where you want to create your project. Run the following commands to create a PhoneGap project and add a platform (e.g., Android):
bash phonegap create myApp cd myApp phonegap platform add android
Replace myApp with your desired project name. This will create a basic PhoneGap project structure.
4. Setting Up Firebase
Now that you have a PhoneGap project in place, let’s set up Firebase for your application. Follow these steps:
4.1 Create a Firebase Project
- Go to the Firebase Console.
- Click on “Add project” to create a new Firebase project.
- Follow the on-screen instructions to configure your project.
4.2 Add Your App to Firebase
Once your Firebase project is created, click on “Add app” and select the appropriate platform (iOS, Android, or web).
Follow the setup instructions to add Firebase to your PhoneGap project. This will include downloading a configuration file (usually google-services.json for Android) and adding it to your project.
4.3 Install Firebase JavaScript SDK
To access Firebase services from your PhoneGap app, you’ll need to include the Firebase JavaScript SDK. Install it using npm:
bash npm install --save firebase
Now, you’re all set to integrate Firebase services into your PhoneGap app.
5. Authenticating Users with Firebase
User authentication is a crucial aspect of many mobile apps. Firebase provides an easy way to authenticate users using various methods, including email and password, social identity providers, and more.
5.1 Setting Up Firebase Authentication
In your PhoneGap project, include the Firebase Authentication module by adding the following code to your HTML file (e.g., index.html):
html <!-- Add this in your HTML file --> <script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-auth.js"></script>
Replace 9.0.0 with the version of Firebase you are using.
5.2 Initialize Firebase Authentication
Initialize Firebase in your JavaScript code by providing your Firebase project configuration. Replace the configuration values with those from your Firebase project:
javascript // Initialize Firebase var firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; firebase.initializeApp(firebaseConfig);
5.3 User Registration and Login
Now, you can implement user registration and login functionality using Firebase Authentication. Here’s a basic example using email and password authentication:
javascript // User Registration function registerUser(email, password) { firebase.auth().createUserWithEmailAndPassword(email, password) .then((userCredential) => { // User registered successfully var user = userCredential.user; console.log("User registered:", user); }) .catch((error) => { // Handle registration errors var errorCode = error.code; var errorMessage = error.message; console.error("Registration error:", errorMessage); }); } // User Login function loginUser(email, password) { firebase.auth().signInWithEmailAndPassword(email, password) .then((userCredential) => { // User logged in successfully var user = userCredential.user; console.log("User logged in:", user); }) .catch((error) => { // Handle login errors var errorCode = error.code; var errorMessage = error.message; console.error("Login error:", errorMessage); }); }
You can create registration and login forms in your app’s HTML and trigger these functions accordingly. Firebase Authentication takes care of user management, including password reset and email verification.
6. Real-Time Database Integration
Firebase offers two primary real-time database options: the Realtime Database and Cloud Firestore. Let’s explore how to integrate each of them into your PhoneGap app.
6.1 Realtime Database
The Realtime Database is a NoSQL cloud database that stores data as JSON and provides real-time synchronization across devices.
6.1.1 Setting Up the Realtime Database
In your Firebase Console, navigate to the “Realtime Database” section, and click on “Create Database.” Choose the location for your database and set the security rules based on your app’s requirements.
To interact with the Realtime Database from your PhoneGap app, include the Firebase Realtime Database module in your HTML:
html <!-- Add this in your HTML file --> <script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-database.js"></script>
Replace 9.0.0 with your Firebase version.
6.1.2 Initialize the Realtime Database
Initialize the Realtime Database in your JavaScript code:
javascript // Initialize the Realtime Database var database = firebase.database();
6.1.3 Writing Data
You can write data to the Realtime Database using the set() method. For example:
javascript // Write data to the Realtime Database function writeData(data) { var ref = database.ref("your-data-path"); ref.set(data); }
Replace “your-data-path” with the path where you want to store your data.
6.1.4 Reading Data
To read data from the Realtime Database and listen for real-time updates, you can use the on() method. Here’s an example:
javascript // Read data from the Realtime Database function readData() { var ref = database.ref("your-data-path"); ref.on("value", (snapshot) => { var data = snapshot.val(); console.log("Data:", data); }); }
This code will retrieve data from the specified path and log it whenever there’s a change.
6.2 Cloud Firestore
Cloud Firestore is a scalable and flexible NoSQL cloud database by Firebase. It provides real-time data syncing and is a great choice for applications that require complex querying and hierarchical data structures.
6.2.1 Setting Up Cloud Firestore
In your Firebase Console, navigate to the “Firestore Database” section, and click on “Create Database.” Choose the location for your database and set the security rules based on your app’s requirements.
To interact with Cloud Firestore from your PhoneGap app, include the Firebase Firestore module in your HTML:
html <!-- Add this in your HTML file --> <script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-firestore.js"></script>
Replace 9.0.0 with your Firebase version.
6.2.2 Initialize Cloud Firestore
Initialize Cloud Firestore in your JavaScript code:
javascript // Initialize Cloud Firestore var db = firebase.firestore();
6.2.3 Writing Data
You can write data to Cloud Firestore using the set() method. For example:
javascript // Write data to Cloud Firestore function writeData(data) { db.collection("your-collection").doc("your-document").set(data) .then(() => { console.log("Data written successfully!"); }) .catch((error) => { console.error("Error writing data:", error); }); }
Replace “your-collection” and “your-document” with your desired collection and document names.
6.2.4 Reading Data
To read data from Cloud Firestore and listen for real-time updates, you can use the onSnapshot() method. Here’s an example:
javascript // Read data from Cloud Firestore function readData() { db.collection("your-collection").doc("your-document") .onSnapshot((doc) => { var data = doc.data(); console.log("Data:", data); }); }
This code will retrieve data from the specified collection and document and log it whenever there’s a change.
7. Implementing Real-Time Features
Now that you have integrated Firebase into your PhoneGap app and learned how to work with both the Realtime Database and Cloud Firestore, you can start implementing real-time features. Here are some ideas:
- Real-Time Chat: Create a chat application where users can send and receive messages in real time.
- Live Notifications: Implement push notifications to notify users of new updates or messages.
- Collaborative Editing: Build a collaborative document editing tool where multiple users can work on the same document simultaneously.
- Live Updates: Display real-time updates of data, such as sports scores or stock prices.
- Location Sharing: Allow users to share their location with friends in real time.
Remember to follow best practices for real-time features, such as handling data synchronization, optimizing database queries, and securing your app’s data.
Conclusion
In this blog post, we’ve explored the integration of PhoneGap and Firebase to create hybrid apps with real-time data capabilities. We started by setting up our development environment, creating a PhoneGap project, and configuring Firebase. Then, we delved into Firebase Authentication for user management and saw how to integrate both the Realtime Database and Cloud Firestore for real-time data synchronization.
By harnessing the power of Firebase, you can create dynamic and engaging hybrid apps that keep your users informed and connected in real time. Whether you’re building a social networking app, a collaborative tool, or any other type of application, Firebase integration can elevate your app to the next level of interactivity and user engagement.
Now that you have the knowledge and tools, it’s time to start building your own real-time hybrid app with PhoneGap and Firebase. Happy coding!
Table of Contents
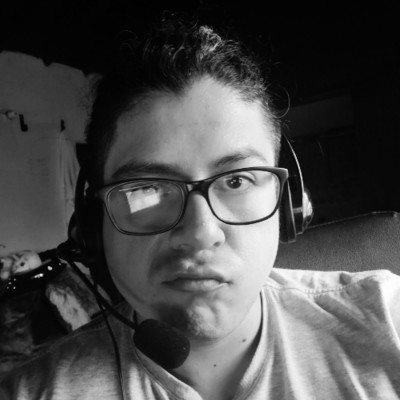
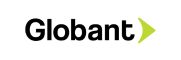