Accelerate Your Development with PHP Frameworks
In the world of web development, time is of the essence. The faster you can build and deploy robust applications, the more competitive you become in the market. This is where PHP frameworks come into play. If you’re a developer looking to streamline your development process and create efficient, maintainable, and scalable web applications, PHP frameworks are your secret weapon. In this article, we’ll explore how PHP frameworks can accelerate your development journey, the benefits they offer, some of the popular frameworks available, and even dive into a few code samples to illustrate their power.
1. Understanding the Power of PHP Frameworks
PHP, one of the most widely used server-side scripting languages, has a rich ecosystem of frameworks designed to simplify and expedite the web development process. These frameworks provide a structured foundation for building applications, ensuring code reusability, maintainability, and adherence to best practices. By utilizing a PHP framework, developers can save significant time and effort by avoiding the need to reinvent the wheel with every new project.
2. Benefits of PHP Frameworks
2.1. Rapid Development
PHP frameworks come with a plethora of built-in tools, libraries, and modules that help developers accelerate the coding process. These tools handle common tasks such as database interactions, form validation, and routing, allowing developers to focus on building unique features rather than the repetitive plumbing.
php // Example: Routing in Laravel Framework Route::get('/products/{id}', 'ProductController@show');
2.2. Code Organization
PHP frameworks enforce a structured approach to code organization, following the Model-View-Controller (MVC) pattern. This separation of concerns enhances code readability, simplifies maintenance, and allows for better collaboration among team members.
php // Example: MVC Structure in Yii Framework class ProductController extends Controller { public function actionShow($id) { $product = Product::findOne($id); return $this->render('show', ['product' => $product]); } }
2.3. Security
Security vulnerabilities can be a nightmare for web developers. PHP frameworks often include security features such as input validation, protection against cross-site scripting (XSS) attacks, and secure authentication mechanisms, reducing the risk of common security pitfalls.
php // Example: Input Validation in Symfony Framework use Symfony\Component\HttpFoundation\Request; $request = Request::createFromGlobals(); $username = $request->request->get('username'); $password = $request->request->get('password'); // Validate and process the input
2.4. Community and Documentation
Popular PHP frameworks have vibrant communities and extensive documentation. This means developers can easily find solutions to common problems, access tutorials, and seek help from experienced developers in online forums.
3. Popular PHP Frameworks to Consider
There are several PHP frameworks available, each with its own strengths and features. Let’s take a look at a few of the most popular ones:
3.1. Laravel
Laravel is often praised for its elegant syntax and developer-friendly features. It comes with a powerful ORM (Object-Relational Mapping) system, a built-in template engine, and a robust ecosystem of packages. Laravel’s intuitive syntax allows developers to write expressive and clean code.
php // Example: Creating a new record in Laravel's Eloquent ORM $product = new Product; $product->name = 'New Product'; $product->price = 99.99; $product->save();
3.2. Symfony
Symfony is known for its flexibility and modularity. It provides a range of reusable components that can be used in various projects. Symfony’s emphasis on decoupling components allows developers to pick and choose the pieces they need for their specific application.
php // Example: Using Symfony's Form Component use Symfony\Component\Form\Extension\Core\Type\TextType; $form = $this->createFormBuilder() ->add('username', TextType::class) ->add('password', TextType::class) ->getForm();
3.3. CodeIgniter
CodeIgniter is renowned for its lightweight nature and minimal footprint. It’s a great choice for small to medium-sized projects where simplicity is key. CodeIgniter’s documentation is comprehensive, making it easy for newcomers to get started.
php // Example: Querying the database in CodeIgniter $this->db->where('id', $id); $query = $this->db->get('products'); $product = $query->row();
4. Diving into Code: Building a Simple Web Application
To illustrate the power of PHP frameworks, let’s build a simple web application using the Laravel framework. In this example, we’ll create a basic task management application with the ability to add, view, and delete tasks.
Step 1: Installation and Setup
Start by installing Laravel using Composer:
bash composer global require laravel/installer
Create a new Laravel project:
bash laravel new TaskManager
Step 2: Create Routes and Views
Define the routes in the routes/web.php file:
php Route::get('/', 'TaskController@index'); Route::post('/task', 'TaskController@store'); Route::delete('/task/{id}', 'TaskController@destroy');
Create the corresponding controller and views:
bash php artisan make:controller TaskController php artisan make:view tasks.index php artisan make:view tasks.form
Step 3: Implement Controller and Database Logic
In the TaskController.php file, implement the methods to handle tasks:
php public function index() { $tasks = Task::all(); return view('tasks.index', ['tasks' => $tasks]); } public function store(Request $request) { Task::create(['name' => $request->name]); return redirect('/'); } public function destroy(Task $task) { $task->delete(); return redirect('/'); }
Step 4: Create Views
Design the task creation form in tasks/form.blade.php:
html <form action="/task" method="POST"> @csrf <input type="text" name="name"> <button type="submit">Add Task</button> </form>
Display tasks in tasks/index.blade.php:
html <ul> @foreach ($tasks as $task) <li> {{ $task->name }} <form action="/task/{{ $task->id }}" method="POST"> @csrf @method('DELETE') <button>Delete</button> </form> </li> @endforeach </ul>
Conclusion
PHP frameworks are essential tools for modern web development, offering developers the means to accelerate their coding process while maintaining code quality and security. With benefits like rapid development, code organization, security features, and a supportive community, PHP frameworks enable developers to focus on building innovative features rather than getting bogged down by repetitive tasks. As we explored through building a simple task management application using the Laravel framework, the power of PHP frameworks becomes evident in their ability to streamline and simplify complex development tasks. Whether you’re a beginner or an experienced developer, incorporating a PHP framework into your workflow can significantly enhance your productivity and the overall quality of your web applications.
Table of Contents
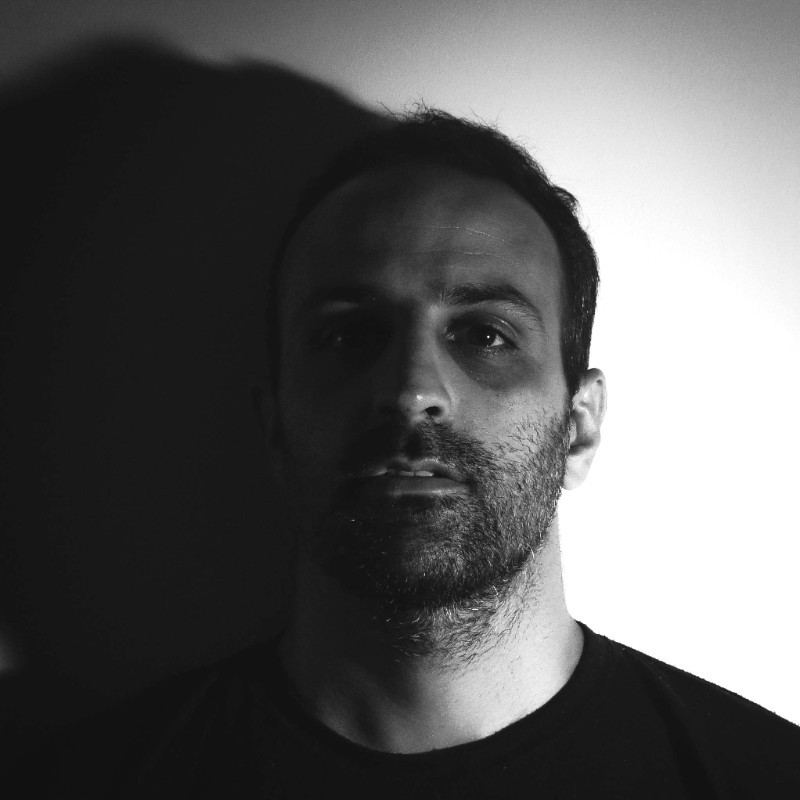
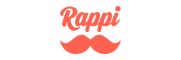