PHP and AJAX: Creating Interactive Web Applications
In today’s digital landscape, users expect web applications to be not only visually appealing but also highly interactive and responsive. To meet these demands, developers need robust technologies that enable real-time data updates without page reloads. This is where PHP and AJAX come into play.
PHP is a server-side scripting language widely used for web development, while AJAX (Asynchronous JavaScript and XML) is a set of techniques that allows web pages to communicate with a server asynchronously, updating content without reloading the entire page. By combining these two powerful technologies, developers can create interactive web applications that deliver dynamic and seamless user experiences.
In this blog post, we will explore the fundamentals of PHP and AJAX, how they work together, and demonstrate how to create interactive web applications using these technologies. Let’s dive in!
Understanding AJAX
AJAX revolutionized web development by introducing a way to update parts of a web page without requiring a full page reload. It accomplishes this by leveraging JavaScript to send asynchronous requests to the server, retrieve data, and update the page content dynamically.
To illustrate the concept, let’s consider a simple example. Assume we have a webpage that displays the weather for a specific location. Traditionally, without AJAX, the page would need to reload every time the user requested the weather for a different location. However, by using AJAX, we can fetch the weather data from the server in the background and update only the relevant portion of the page, resulting in a more fluid and seamless user experience.
AJAX operates by leveraging JavaScript’s XMLHttpRequest object or more modern approaches such as the Fetch API or jQuery’s AJAX functions. These methods enable the client-side code to send requests to the server, receive responses, and update the web page dynamically without disrupting the user’s workflow.
PHP: A Powerful Server-Side Language
PHP is a versatile scripting language primarily used for server-side web development. Its popularity stems from its simplicity, flexibility, and extensive support for interacting with databases and handling HTTP requests.
When it comes to building interactive web applications, PHP’s server-side capabilities play a crucial role. It can process user input, interact with databases to fetch or store data, and generate dynamic content that can be seamlessly integrated with AJAX on the client side.
To demonstrate the power of PHP in conjunction with AJAX, let’s look at a practical example. Suppose we have a web application that allows users to post comments on an article. Using AJAX, we can send the comment data to the server asynchronously, where PHP can process it, validate and sanitize the input, and store the comment in a database. Once the server completes the operation, it can send a response back to the client, which AJAX can then handle to update the user interface with the new comment, all without refreshing the page.
Example Code: AJAX Request with PHP Processing
To better understand the interplay between PHP and AJAX, let’s examine a code snippet that demonstrates a basic AJAX request to a PHP script.
javascript // AJAX request var xhr = new XMLHttpRequest(); xhr.open("POST", "process_comment.php", true); xhr.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var response = JSON.parse(xhr.responseText); // Handle the response from the server // Update the page dynamically } }; xhr.send("comment=" + encodeURIComponent(userComment));
In this example, we create an XMLHttpRequest object and configure it to send a POST request to the “process_comment.php” script. We also set the appropriate content type and define a callback function to handle the server’s response. Once the request is ready, we send it along with the user’s comment data.
On the server side, the “process_comment.php” script receives the AJAX request, extracts the comment data, performs necessary processing (such as validation and sanitization), and stores the comment in the database. Finally, it sends back a response to the client-side code, which can then handle the response and update the web page accordingly.
Conclusion
PHP and AJAX are powerful technologies that, when combined, allow developers to create interactive web applications with dynamic content and responsive user experiences. Leveraging AJAX’s ability to send asynchronous requests and PHP’s server-side processing capabilities, developers can build web applications that update specific sections of a page without requiring full page reloads.
Throughout this blog post, we’ve explored the fundamentals of PHP and AJAX, their symbiotic relationship, and provided an example code snippet illustrating how they can work together. Armed with this knowledge, you’re now equipped to harness the power of PHP and AJAX to create immersive and engaging web applications.
Remember, the key to mastering these technologies lies in practice and experimentation. So, get hands-on, explore the vast capabilities they offer, and unlock the true potential of interactive web application development.
Table of Contents
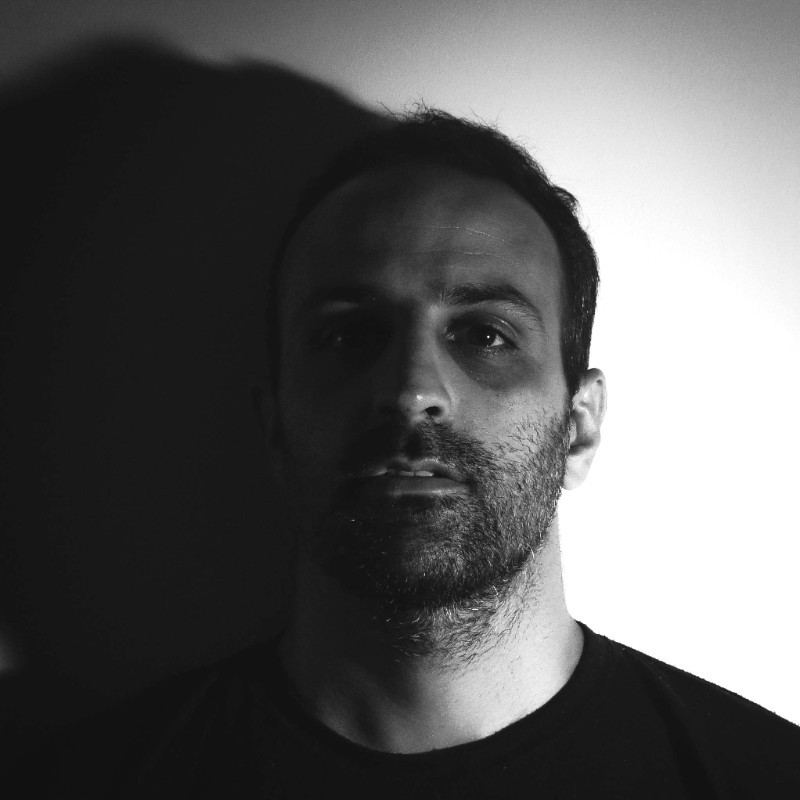
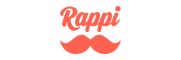