PHP and Cookies: A Guide to Web Session Management
In today’s web development landscape, managing user sessions is a crucial aspect of building secure and user-friendly applications. PHP, being one of the most popular server-side scripting languages, offers robust features for handling cookies, which are a fundamental component of web session management. In this comprehensive guide, we will delve into the world of PHP and cookies, exploring their role in managing web sessions and uncovering best practices along the way. Whether you are a seasoned PHP developer or just starting out, this guide will equip you with the knowledge and tools to master web session management using PHP’s cookie capabilities.
Understanding Cookies and Web Sessions
Before diving into the details of PHP’s cookie handling, it is essential to grasp the concepts of cookies and web sessions. Cookies are small pieces of data that a web server sends to a user’s browser and are stored on the client-side. They serve as a means of persisting information between HTTP requests, enabling stateful interactions in stateless HTTP protocols. Web sessions, on the other hand, represent the logical connections or interactions between a user and a web application. They allow the server to maintain user-specific data across multiple requests, forming the foundation for user authentication, personalization, and more.
Setting and Retrieving Cookies in PHP
PHP provides a convenient and straightforward mechanism for setting and retrieving cookies. By using the setcookie() function, you can specify the cookie’s name, value, and other attributes. On subsequent requests, PHP automatically populates the $_COOKIE superglobal array, allowing you to access the cookie’s value. This section of the guide will walk you through the process of setting and retrieving cookies in PHP, providing code examples for clarity.
php // Setting a cookie setcookie("username", "JohnDoe", time() + 3600, "/"); // Retrieving a cookie if (isset($_COOKIE["username"])) { $username = $_COOKIE["username"]; echo "Welcome back, " . $username; } else { echo "Welcome, guest"; }
Cookie Attributes and Best Practices
To make the most of PHP’s cookie handling capabilities, it’s important to understand the various attributes that can be set when creating cookies. This section explores essential attributes such as expiration and persistence, domain and path restriction, and security flags like Secure and HttpOnly. By leveraging these attributes effectively, you can enhance the security and usability of your web sessions.
- Expiration and Persistent Cookies
Setting an appropriate expiration time for a cookie determines how long it remains valid. PHP’s setcookie() function allows you to specify the expiration time as a Unix timestamp or in a relative format using the time() function. Persistent cookies, which have expiration dates in the future, can be used to create “remember me” functionality or to maintain long-term user preferences.
php // Setting a persistent cookie for 30 days setcookie("remember_me", "1", time() + 30 * 24 * 60 * 60, "/");
- Domain and Path Restriction
Cookies can be restricted to specific domains and paths, ensuring they are only sent back to the server when requested from matching URLs. This attribute helps prevent unauthorized access to cookies by restricting their availability to specific parts of your website.
php // Setting a cookie with domain and path restrictions setcookie("mycookie", "value", time() + 3600, "/", "example.com");
- Secure and HttpOnly Flags
The Secure flag ensures that cookies are only transmitted over encrypted connections (HTTPS), reducing the risk of interception and eavesdropping. The HttpOnly flag restricts access to cookies through client-side scripts, mitigating cross-site scripting (XSS) attacks.
php // Setting a secure and HttpOnly cookie setcookie("sensitive_data", "value", time() + 3600, "/", "", true, true);
Managing Sessions with Cookies
PHP’s cookie handling capabilities play a crucial role in managing web sessions effectively. This section focuses on techniques such as creating and updating session IDs, storing session data in cookies, and managing session expiration and invalidation.
- Creating and Updating Session IDs
Session IDs serve as unique identifiers for each user session. PHP automatically generates a session ID and stores it in a cookie named “PHPSESSID” by default. However, you can customize the session ID’s name using the session_name() function.
php // Creating a custom session ID session_name("MYAPPSESSID"); session_start();
- Storing Session Data in Cookies
In some cases, it may be desirable to store session data directly in cookies instead of server-side storage mechanisms like file-based or database-based sessions. This approach eliminates the need for server-side session storage, reducing the load on the server and improving scalability.
php // Storing session data in cookies $_SESSION["username"] = "JohnDoe"; setcookie("session_data", base64_encode(json_encode($_SESSION)), time() + 3600, "/");
- Session Expiration and Invalidation
Session expiration is crucial for maintaining security and managing server resources efficiently. PHP provides options to control session expiration based on idle time, absolute time, or custom logic. Additionally, invalidating sessions on logout or user-specific actions is essential to prevent unauthorized access to session data.
php // Configuring session expiration ini_set("session.gc_maxlifetime", 3600); // 1 hour ini_set("session.cookie_lifetime", 0); // expire on browser close // Invalidating a session session_destroy(); setcookie("PHPSESSID", "", time() - 3600, "/");
Advanced Techniques for Web Session Security
To enhance web session security, additional measures beyond basic cookie handling may be required. This section explores advanced techniques such as cookie encryption and integrity checks, as well as protecting against cross-site request forgery (CSRF) attacks using cookies.
- Cookie Encryption and Integrity
Encrypting sensitive data stored in cookies prevents unauthorized access and tampering. Techniques like symmetric or asymmetric encryption, combined with hashing and digital signatures, can provide a robust security layer for cookie-based session management.
php // Encrypting and decrypting cookie data $encryptedData = encryptData($data); $decryptedData = decryptData($encryptedData);
- CSRF Protection with Cookies
Cookies can be leveraged to protect against CSRF attacks by including a token that is sent with each request. The server validates the token’s integrity to ensure the authenticity of the request, mitigating the risk of unauthorized actions.
php // Generating and validating CSRF tokens $token = generateCSRFToken(); if (validateCSRFToken($token)) { // Proceed with the request } else { // Handle the invalid request }
Common Pitfalls and Best Practices
While PHP’s cookie handling offers powerful capabilities, it’s essential to be aware of common pitfalls and adopt best practices to ensure efficient and secure web session management. This section covers aspects such as cookie size limitations, cookie-based authentication, and considerations for GDPR compliance and user privacy.
- Cookie Size Limitations
Cookies have size limitations imposed by browser restrictions. It’s crucial to be mindful of these limitations and avoid storing excessive or sensitive data in cookies. When faced with data size constraints, consider alternative approaches like storing data in server-side sessions or databases.
- Handling Cookie-Based Authentication
Using cookies for authentication requires careful implementation to prevent security vulnerabilities like session fixation or session hijacking. Adopting secure practices like regenerating session IDs on login, implementing session timeouts, and applying strict cookie attributes can help mitigate these risks.
- GDPR and Privacy Considerations
The General Data Protection Regulation (GDPR) places stringent requirements on data protection and user privacy. When working with cookies, ensure compliance with GDPR regulations by providing clear cookie consent mechanisms, allowing users to manage their cookie preferences, and respecting “Do Not Track” requests.
Future Trends and Alternatives
While PHP’s cookie handling remains a popular and effective approach for web session management, other techniques and technologies are emerging. This section briefly explores alternatives such as JSON Web Tokens (JWT) and session handling with databases, providing insights into potential future trends.
Conclusion
Web session management is a critical aspect of building secure and user-friendly applications. By mastering PHP’s cookie handling capabilities, you can effectively manage web sessions, enhance security, and provide a seamless user experience. In this guide, we explored the fundamentals of PHP and cookies, delved into advanced techniques for web session security, and discussed common pitfalls and best practices. Armed with this knowledge, you are now ready to embark on your journey to becoming a proficient web developer skilled in PHP and web session management.
Table of Contents
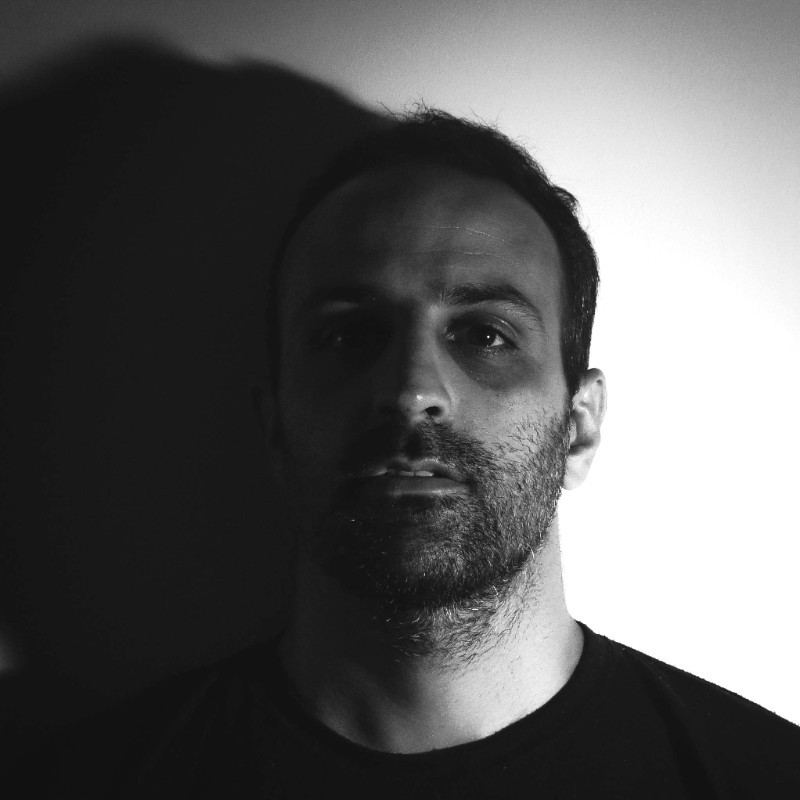
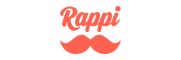