PHP and JavaScript: Making the Perfect Blend
In the realm of web development, PHP and JavaScript have long been the powerhouses that drive dynamic and interactive web applications. PHP, a server-side scripting language, provides robust back-end functionality, while JavaScript, a client-side scripting language, brings interactivity to the front end. Together, they form a perfect blend that enables developers to create seamless web experiences. In this blog post, we will delve into the world of PHP and JavaScript, explore their individual strengths, and uncover how they can be combined to build powerful web applications.
Understanding PHP and JavaScript
- PHP: The Backbone of Server-side Logic
PHP, which stands for Hypertext Preprocessor, is a versatile scripting language primarily used for server-side web development. It enables developers to generate dynamic web pages by embedding code within HTML. PHP offers a wide range of features, including database connectivity, session management, file manipulation, and more. With its extensive library of functions and frameworks like Laravel and Symfony, PHP provides a solid foundation for server-side logic.
Code Sample:
php <?php // Simple PHP code example $name = "John"; echo "Hello, $name!"; ?>
- JavaScript: Empowering Client-side Interactivity
JavaScript is a powerful scripting language that runs on the client-side, enabling dynamic and interactive web experiences. It allows developers to manipulate HTML elements, handle events, create animations, and communicate with servers asynchronously. JavaScript frameworks such as React, Angular, and Vue.js have further expanded its capabilities, making it a go-to choice for front-end development.
Code Sample:
javascript // Simple JavaScript code example let name = "John"; console.log(`Hello, ${name}!`);
The Benefits of Combining PHP and JavaScript
- Separation of Concerns: Back-end and Front-end Collaboration
By separating back-end and front-end concerns, the combination of PHP and JavaScript promotes a clean and maintainable codebase. PHP handles server-side logic, data processing, and database interactions, while JavaScript takes care of the user interface and interactivity. This division of responsibilities allows developers to work on their respective areas of expertise, leading to more efficient and scalable development processes.
- Enhanced User Experience: Real-time Updates and Interactivity
JavaScript’s ability to update web pages dynamically without requiring a full page reload complements PHP’s server-side processing. Through AJAX (Asynchronous JavaScript and XML) requests, developers can leverage PHP to retrieve data from the server and use JavaScript to update specific parts of the page in real-time. This approach enhances the user experience by providing instant feedback, reducing latency, and creating a smoother browsing experience.
Code Sample:
javascript // AJAX request using JavaScript and PHP let xhr = new XMLHttpRequest(); xhr.open("GET", "api/data.php", true); xhr.onreadystatechange = function () { if (xhr.readyState === 4 && xhr.status === 200) { let response = JSON.parse(xhr.responseText); // Update UI with the retrieved data document.getElementById("data-container").innerHTML = response.data; } }; xhr.send();
- Code Reusability: Shared Logic between Back-end and Front-end
PHP and JavaScript allow developers to share code and logic between the server and the client. By utilizing frameworks like Node.js, developers can write JavaScript code that runs on both the server and the browser. This enables seamless integration and reuse of validation logic, data manipulation functions, and other shared functionalities, reducing duplication and promoting consistency across the application.
Best Practices for PHP and JavaScript Integration
- Handling Data Exchange: JSON and API Endpoints
To establish seamless communication between PHP and JavaScript, JSON (JavaScript Object Notation) serves as the lingua franca. PHP’s native functions such as json_encode() and json_decode() facilitate data exchange between the server and the client. Additionally, defining well-structured API endpoints in PHP allows JavaScript to consume and manipulate data effectively.
Code Sample:
php <?php // PHP code to generate JSON response $data = array("name" => "John", "age" => 30); header('Content-Type: application/json'); echo json_encode($data); ?> javascript // JavaScript code to consume API endpoint fetch("api/data.php") .then(response => response.json()) .then(data => { // Work with the retrieved data console.log(data.name, data.age); });
- Security Considerations: Input Validation and Cross-Site Scripting (XSS) Prevention
When integrating PHP and JavaScript, it is crucial to ensure the security of your application. PHP provides various functions for input validation and sanitization to prevent common vulnerabilities like SQL injection and Cross-Site Scripting (XSS). By validating user input on the server-side using PHP and implementing client-side validation with JavaScript, you can establish a robust security framework for your web application.
- Optimizing Performance: Caching and Minification
To enhance the performance of PHP and JavaScript-driven web applications, it’s essential to implement caching and minification techniques. PHP offers caching mechanisms like APCu and Redis, which can improve response times by storing frequently accessed data in memory. JavaScript, on the other hand, can be minified and concatenated using tools like UglifyJS to reduce file sizes and minimize network requests.
Real-world Examples
- Single-Page Applications (SPAs): Combining PHP and JavaScript
Single-Page Applications (SPAs) have gained popularity for their seamless user experience. By combining PHP for server-side rendering and JavaScript frameworks like React or Vue.js for client-side interactivity, developers can create SPAs that dynamically update the UI without page reloads. PHP’s routing capabilities can also be utilized to handle API requests and serve the initial HTML page.
- Real-time Collaborative Applications: PHP, JavaScript, and WebSockets
Real-time collaborative applications, such as chat systems or collaborative editors, heavily rely on real-time communication. PHP can handle the server-side logic, while JavaScript, in combination with WebSockets, enables bidirectional communication between the server and the client. With this approach, users can experience instantaneous updates and interactions within the application.
Conclusion
The combination of PHP and JavaScript presents developers with a powerful toolkit for creating dynamic, interactive, and scalable web applications. By leveraging the strengths of each language, developers can harness PHP’s robust server-side capabilities and JavaScript’s front-end interactivity. Whether it’s building SPAs, real-time collaborative applications, or any other web project, understanding the synergy between PHP and JavaScript opens up a world of possibilities. Embrace this perfect blend, and embark on a journey to create exceptional web experiences.
Table of Contents
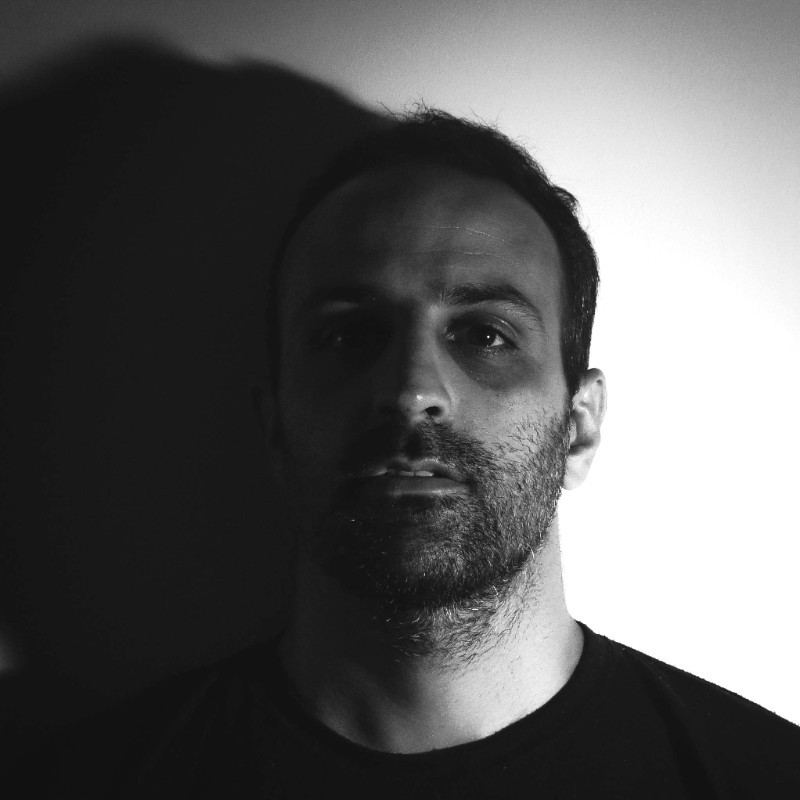
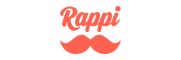