The Power of PHP’s array_diff() Function
In the realm of programming, arrays stand as fundamental data structures that store a collection of values. When working with arrays, developers often find themselves needing to compare arrays to identify differences between them. This is where PHP’s array_diff() function comes into play, offering a robust and efficient solution to this common challenge. In this blog post, we will explore the capabilities of the array_diff() function, its practical applications, and how it enhances the development process.
Table of Contents
1. Understanding array_diff()
1.1. What is array_diff()?
array_diff() is a built-in PHP function designed to compare arrays and return the differences between them. It accepts two or more arrays as arguments and returns an array containing the values that are present in the first array but not in the subsequent arrays.
1.2. Syntax
The syntax of the array_diff() function is as follows:
php array_diff(array $array1, array $array2 [, array $... ])
Where:
- $array1 is the initial array to compare against.
- $array2 is the array to compare with $array1.
- $… represents additional arrays that can be compared with $array1.
2. Working with array_diff()
2.1. Basic Usage
Let’s dive into some code examples to understand how array_diff() works. Consider two arrays, $array1 and $array2, as shown below:
php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7];
To find the values that exist in $array1 but not in $array2, you can use the array_diff() function:
php $diff = array_diff($array1, $array2); print_r($diff);
Output:
csharp Array ( [0] => 1 [1] => 2 )
In this example, the values 1 and 2 are present in $array1 but not in $array2. Therefore, they are returned in the $diff array.
2.2. Numeric and Associative Arrays
The array_diff() function works seamlessly with both numeric and associative arrays. Consider the following example:
php $numericArray1 = [1, 2, 3, 4, 5]; $numericArray2 = [3, 4, 5, 6, 7]; $assocArray1 = ['a' => 1, 'b' => 2, 'c' => 3]; $assocArray2 = ['c' => 3, 'd' => 4, 'e' => 5]; $numericDiff = array_diff($numericArray1, $numericArray2); $assocDiff = array_diff($assocArray1, $assocArray2); print_r($numericDiff); print_r($assocDiff);
Output:
csharp Array ( [0] => 1 [1] => 2 ) Array ( [a] => 1 [b] => 2 )
As shown in the example, array_diff() maintains the original keys when working with associative arrays, ensuring that the association between keys and values is preserved.
2.3. Handling Complex Data
array_diff() is not limited to simple arrays containing primitive data types. It can also handle complex data structures, including arrays of objects or arrays of arrays. The function performs a deep comparison to identify differences even within nested structures. Let’s consider a scenario where we have an array of objects:
php class Product { public $id; public $name; public function __construct($id, $name) { $this->id = $id; $this->name = $name; } } $product1 = new Product(1, 'Widget'); $product2 = new Product(2, 'Gadget'); $array1 = [$product1, $product2]; $array2 = [$product2]; $diff = array_diff($array1, $array2); print_r($diff);
Output:
css Array ( [0] => Product Object ( [id] => 1 [name] => Widget ) )
In this example, the array_diff() function compares the objects within the arrays and identifies that the object with ID 1 and name Widget is present in $array1 but not in $array2.
3. Practical Use Cases
3.1. Data Synchronization
One of the most common use cases for array_diff() is in data synchronization. Imagine you have two arrays representing datasets from different sources. By using array_diff(), you can quickly identify new or changed data points that need to be synchronized between the sources.
php $sourceData = [...]; // Data from a source $localData = [...]; // Locally stored data $missingData = array_diff($sourceData, $localData); $newData = array_diff($localData, $sourceData); // Perform synchronization operations using $missingData and $newData
3.2. User Permissions
When dealing with user roles and permissions, array_diff() can be invaluable. Consider a scenario where a user has a list of permissions stored in an array. By comparing this array with the permissions associated with a certain role, you can identify the permissions that the user lacks.
php $userPermissions = ['read', 'write', 'delete']; $rolePermissions = ['read', 'write', 'execute']; $missingPermissions = array_diff($rolePermissions, $userPermissions);
In this example, $missingPermissions will contain the permission ‘execute’, which the user does not possess based on their assigned permissions.
4. Benefits of Using array_diff()
4.1. Efficiency
The array_diff() function is optimized for performance, making it an efficient solution for array comparison tasks. Its underlying algorithm minimizes the number of comparisons required, resulting in faster execution times even for larger arrays.
4.2. Simplicity
Using array_diff() simplifies the code required for array comparisons. It eliminates the need for manual loops and conditional checks, reducing the chances of introducing errors in the comparison process.
4.3. Preserved Keys
As demonstrated earlier, array_diff() maintains the original keys of elements in associative arrays. This behavior ensures that the relationships between keys and values remain intact throughout the comparison process.
4.4. Versatility
The function’s ability to handle complex data structures and deep comparisons adds to its versatility. It can be applied to various scenarios, from comparing primitive values to intricate objects.
Conclusion
PHP’s array_diff() function is a powerful tool that streamlines the process of comparing arrays and identifying differences. Its simplicity, efficiency, and ability to handle diverse data types make it an essential asset in a developer’s toolkit. Whether you’re synchronizing data, managing user permissions, or performing other array-related tasks, array_diff() simplifies the coding process and enhances the efficiency of your applications. By mastering this function, you unlock the potential to create more robust and reliable software systems.
Table of Contents
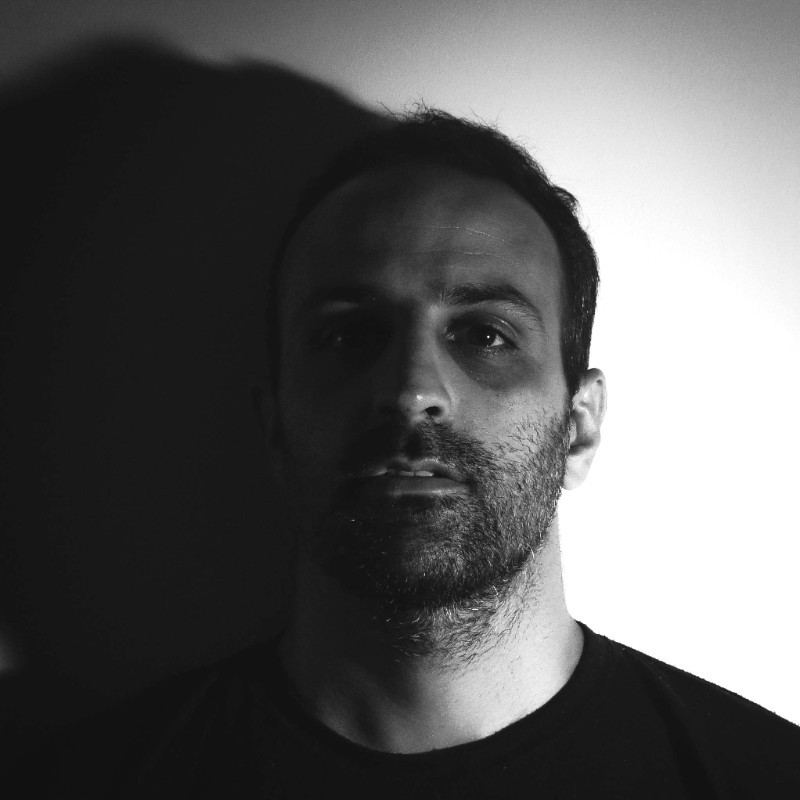
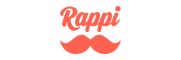