A Deep Dive into PHP’s array_fill() Function
PHP is a versatile and widely-used scripting language for web development. Its extensive standard library provides developers with a plethora of built-in functions to simplify common tasks. One such function is array_fill(), a powerful tool for creating arrays with repeated values. In this blog post, we will take a deep dive into PHP’s array_fill() function, exploring its syntax, use cases, and practical examples to demonstrate its versatility.
Table of Contents
1. Understanding the Basics
1.1. Syntax of array_fill()
Before we dive into the practical aspects of array_fill(), let’s first understand its syntax:
php array_fill(start_index, num, value)
- start_index: The starting index for filling the array.
- num: The number of elements to fill.
- value: The value to be filled in each element.
1.2. Return Value
The array_fill() function returns a new array filled with the specified value. The keys of the array will start from start_index and continue up to start_index + num – 1. If num is zero or negative, the function will return an empty array.
2. Practical Use Cases
Now that we have a grasp of the basic syntax, let’s explore some practical use cases for array_fill().
2.1. Creating an Array of Default Values
One common use case for array_fill() is to create an array with default values. This can be especially useful when you need to initialize an array with a predefined set of values. For example, suppose you want to create an array of 10 elements, all initialized to the value 0:
php $defaultValues = array_fill(0, 10, 0);
In this example, we specify a start_index of 0, num as 10, and the value as 0. The resulting $defaultValues array will contain 10 elements, all set to 0.
2.2. Generating an Array of Sequential Numbers
Another practical use case is generating an array of sequential numbers. This can be handy in scenarios where you need to create an array of numbers for pagination or looping purposes. For example, to generate an array of numbers from 1 to 10, you can use the following code:
php $sequentialNumbers = array_fill(1, 10, null);
In this case, we specify a start_index of 1, num as 10, and null as the value. This will result in an array containing the numbers from 1 to 10, with keys ranging from 1 to 10.
2.3. Filling an Array with Default Values at Specific Indices
Sometimes, you may want to fill specific indices of an array with default values while leaving other elements unchanged. array_fill() allows you to achieve this easily. Consider the following example:
php $myArray = [10, 20, 30, 40, 50]; $filledArray = $myArray; // Fill indices 1 to 3 with the value 0 $filledArray = array_replace($filledArray, array_fill(1, 3, 0));
In this example, we have an existing array $myArray, and we want to fill indices 1 to 3 with the value 0 without affecting the rest of the array. We create a new array $filledArray by combining the original array with the result of array_fill() for the specified indices.
2.4. Populating an Associative Array
array_fill() is not limited to indexed arrays; it can also be used to populate associative arrays. Suppose you want to create an associative array where all keys are initially set to a specific value, say null:
php $keys = ['name', 'email', 'phone', 'address']; $defaultValues = array_fill_keys($keys, null);
Here, we use array_fill_keys() in combination with array_fill() to populate the associative array. The resulting $defaultValues array will have keys ‘name’, ’email’, ‘phone’, and ‘address’, all set to null.
3. Practical Examples
Now that we’ve explored some use cases, let’s dive into practical examples to see array_fill() in action.
Example 1: Creating a Chessboard
Imagine you want to create a chessboard represented as a two-dimensional array in PHP. You can use array_fill() to initialize the chessboard with empty squares (‘E’) and then set the positions of the chess pieces accordingly. Here’s a simplified example:
php $chessboard = []; // Initialize the chessboard with empty squares for ($i = 0; $i < 8; $i++) { $chessboard[$i] = array_fill(0, 8, 'E'); } // Set the positions of the chess pieces $chessboard[0][0] = 'R'; $chessboard[0][7] = 'R'; $chessboard[7][0] = 'r'; $chessboard[7][7] = 'r'; // ... (other piece positions)
In this example, we create an 8×8 chessboard where all squares are initially set to ‘E’ (empty). We then set the positions of the rooks (‘R’ and ‘r’) and other pieces as needed.
Example 2: Creating a Simple Bar Chart
Suppose you want to create a simple bar chart where each bar’s height is determined by an array of values. You can use array_fill() to initialize an array with spaces (‘ ‘) and then fill in the bars at specific positions. Here’s an example:
php $values = [3, 6, 2, 8, 4]; // Initialize the bar chart with spaces $chart = array_fill(0, max($values), ' '); // Fill in the bars at appropriate positions foreach ($values as $position => $height) { $chart[$position] = str_repeat('*', $height); } // Print the bar chart foreach (array_reverse($chart) as $row) { echo $row . PHP_EOL; }
In this example, we initialize the $chart array with spaces and then use a loop to fill in the bars at positions corresponding to the values in the $values array. Finally, we print the bar chart, with taller bars represented by more asterisks (‘*’).
4. Tips and Best Practices
While array_fill() is a useful function, there are some tips and best practices to keep in mind when using it:
- Avoid Negative Values for num: Be cautious when using negative values for num, as it will result in an empty array. Always ensure that num is a positive integer or zero.
- Combine with Other Array Functions: array_fill() can be combined with other array functions like array_replace() and array_fill_keys() to perform more complex array manipulations efficiently.
- Consider Alternatives: In some cases, using a loop or other array-building techniques may be more efficient or offer greater flexibility than array_fill(). Assess your specific requirements before choosing this function.
- Error Handling: Handle any potential errors or edge cases that may arise when working with arrays created using array_fill(). Check for null or empty values if necessary.
- Performance: While array_fill() is convenient, it may not be the fastest option for creating large arrays. For performance-critical applications, consider alternative approaches like direct array construction.
Conclusion
In this deep dive into PHP’s array_fill() function, we’ve explored its syntax, practical use cases, and provided real-world examples to illustrate its versatility. Whether you need to initialize arrays with default values, create sequential arrays, or populate associative arrays, array_fill() can simplify your PHP coding tasks. However, it’s essential to use it judiciously and consider alternative approaches when necessary to ensure efficient and error-free code. With a solid understanding of array_fill(), you can enhance your PHP development skills and build more robust applications.
Now that you’ve learned about array_fill(), consider experimenting with it in your PHP projects to see how it can streamline your array manipulation tasks and make your code more efficient. Happy coding!
Table of Contents
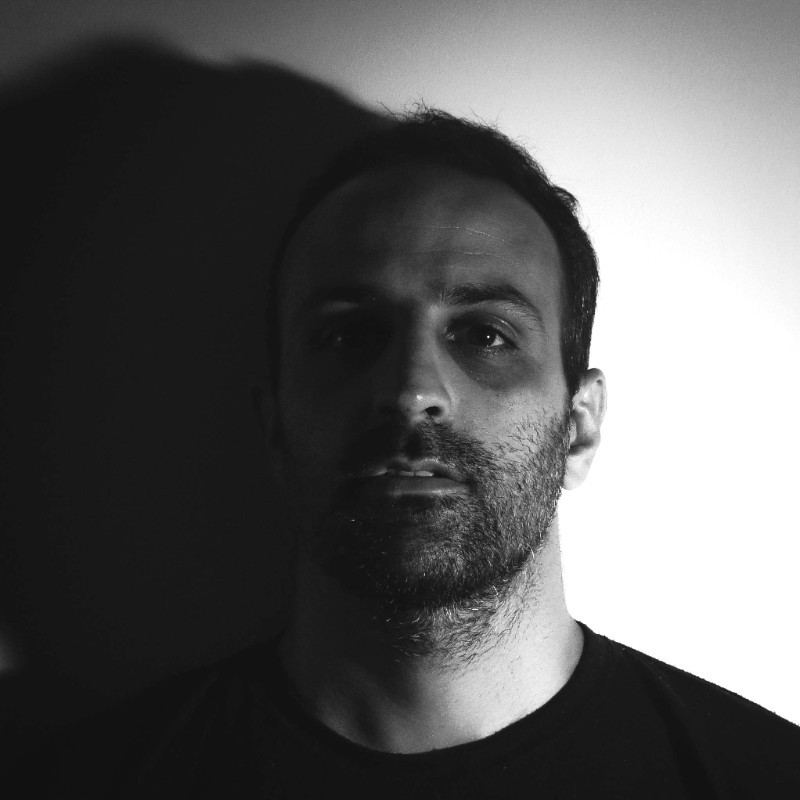
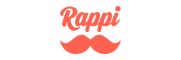