The Power of PHP’s array_filter() Function
When it comes to handling arrays in PHP, developers are often faced with the task of filtering out specific elements based on certain conditions. This is where the array_filter() function shines, providing an elegant and efficient solution. In this blog post, we’ll delve into the various aspects of array_filter() and explore how it can supercharge your array manipulation tasks.
1. Understanding array_filter()
At its core, the array_filter() function is designed to iterate through an array and retain only those elements that satisfy a given callback function’s conditions. This versatile function accepts two mandatory arguments:
- The input array that you want to filter.
- A callback function that defines the filtering conditions.
Let’s dive into some code to better understand how it works:
php // An array of numbers $numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; // Callback function to filter even numbers function filterEven($number) { return $number % 2 === 0; } // Applying array_filter() with the callback $evenNumbers = array_filter($numbers, 'filterEven'); // Result: [2, 4, 6, 8, 10]
In this example, the filterEven() function is the callback used by array_filter() to retain only the even numbers from the input array. This simple yet powerful approach saves developers from writing explicit loops and conditionals, resulting in cleaner and more readable code.
2. Leveraging Callback Functions
The real strength of array_filter() lies in the flexibility it offers through callback functions. These functions can be user-defined or predefined, granting you the freedom to apply a wide range of filtering criteria to your arrays.
2.1. User-Defined Callbacks
User-defined callback functions allow you to tailor the filtering logic to your specific requirements. Whether you’re working with complex data structures or need to implement custom filtering rules, the possibilities are virtually endless.
Let’s say you have an array of products and you want to filter out items with prices exceeding $50:
php $products = [ ['name' => 'Widget A', 'price' => 30], ['name' => 'Widget B', 'price' => 60], ['name' => 'Widget C', 'price' => 25], // ... ]; // User-defined callback to filter expensive products function filterExpensive($product) { return $product['price'] <= 50; } $affordableProducts = array_filter($products, 'filterExpensive'); // Result: Only products with prices up to $50
2.2. Predefined Callbacks
PHP provides a set of predefined functions that can serve as callbacks for common filtering scenarios. These functions are not only efficient but also save time and effort.
For instance, you can use the strlen() function to filter out strings with a specific length:
php $words = ['apple', 'banana', 'cherry', 'date', 'fig']; // Using strlen() as a callback to filter words with length > 5 $filteredWords = array_filter($words, 'strlen'); // Result: ['banana', 'cherry']
3. Handling Callback Parameters
When designing callback functions, it’s essential to understand how parameters are passed to them. The callback function can accept up to three parameters:
- The current array element’s value.
- The current array element’s key.
- Additional arguments (if provided) passed to array_filter().
This parameter-passing mechanism provides the necessary context for creating dynamic and context-aware filtering functions.
4. Using Anonymous Functions
Anonymous functions, also known as closures, are a powerful way to create callbacks on the fly. They allow you to define ad-hoc functions directly within the array_filter() call, avoiding the need to declare separate functions.
php $temperatures = [32, 45, 50, 28, 38, 55]; // Filtering temperatures above 40 using an anonymous function $hotDays = array_filter($temperatures, function($temperature) { return $temperature > 40; }); // Result: [45, 50, 55]
5. Handling Associative Arrays
array_filter() works seamlessly with associative arrays as well. When applied to an associative array, it retains the key-value associations of the filtered elements.
php $students = [ 'Alice' => ['age' => 22, 'grade' => 'A'], 'Bob' => ['age' => 19, 'grade' => 'B'], 'Carol' => ['age' => 25, 'grade' => 'A'], // ... ]; // Filtering students with grade 'A' $topStudents = array_filter($students, function($student) { return $student['grade'] === 'A'; }); // Result: ['Alice' => ['age' => 22, 'grade' => 'A'], 'Carol' => ['age' => 25, 'grade' => 'A']]
6. Combining with Other Array Functions
One of the true benefits of array_filter() is its compatibility with other array functions. You can seamlessly combine it with functions like array_map(), array_reduce(), and more to create sophisticated data transformations.
php $numbers = [1, 2, 3, 4, 5, 6]; // Squaring even numbers using array_filter() and array_map() $squaredEvenNumbers = array_map(function($number) { return $number * $number; }, array_filter($numbers, 'filterEven')); // Result: [4, 16, 36]
Conclusion
In the realm of PHP programming, the array_filter() function emerges as an indispensable tool for manipulating arrays. Its ability to streamline filtering tasks, coupled with the flexibility of callback functions, makes it an elegant solution for enhancing code efficiency and readability. By harnessing the power of array_filter(), developers can conquer complex array filtering challenges with ease, ultimately leading to cleaner and more maintainable codebases. So, whether you’re dealing with numeric arrays, associative arrays, or multidimensional data structures, remember that array_filter() has the potential to revolutionize your array manipulation workflow.
Table of Contents
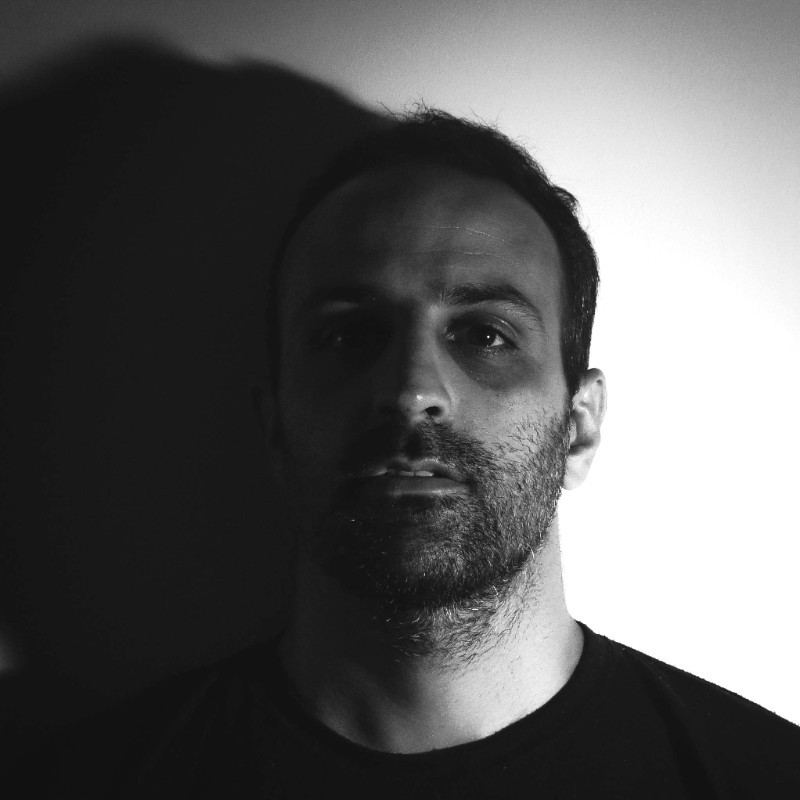
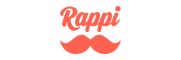