Unraveling PHP’s array_intersect() Function: A Detailed Guide
PHP is a versatile and widely-used scripting language that’s essential for web development. It provides a plethora of functions and features to simplify various tasks, including working with arrays. One such function that comes in handy when dealing with arrays is array_intersect(). This powerful function allows you to find common elements between two or more arrays effortlessly. In this detailed guide, we will dive deep into the array_intersect() function, exploring its syntax, use cases, and practical examples to help you harness its full potential.
Table of Contents
1. Introduction to array_intersect()
1.1 What is array_intersect()?
In PHP, array_intersect() is a built-in function that allows you to compare two or more arrays and return the values that exist in all of them. It’s an incredibly useful tool for various scenarios, such as filtering data, ensuring unique values, and performing intersection operations.
1.2 Syntax of array_intersect()
Before we dive into practical examples, let’s take a look at the syntax of the array_intersect() function:
php array_intersect(array $array1, array $array2 [, array $... ]): array
$array1, $array2, and $… (optional) are the arrays you want to compare.
The function returns an array containing the values that exist in all the input arrays.
2. Basic Usage
2.1 Comparing Two Arrays
Let’s start with a straightforward example of comparing two arrays using array_intersect():
php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $result = array_intersect($array1, $array2); print_r($result);
Output:
php Array ( [2] => 3 [3] => 4 [4] => 5 )
In this example, $result contains the values that are common between $array1 and $array2. As you can see, it returned the values 3, 4, and 5.
2.2 Finding Common Elements
array_intersect() is particularly handy when you need to find common elements between arrays of different sizes. Consider the following example:
php $array1 = [10, 20, 30, 40, 50]; $array2 = [30, 40]; $result = array_intersect($array1, $array2); print_r($result);
Output:
php Array ( [2] => 30 [3] => 40 )
Here, $result contains the values that exist in both $array1 and $array2, which are 30 and 40. The function automatically identifies the common elements for you.
3. Advanced Usage
3.1 Comparing Multiple Arrays
While comparing two arrays is common, you can also use array_intersect() with more than two arrays. Simply add additional arrays as arguments to the function:
php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $array3 = [5, 6, 7, 8, 9]; $result = array_intersect($array1, $array2, $array3); print_r($result);
Output:
php Array ( [2] => 5 )
In this example, $result contains the value 5, which is the only value present in all three arrays.
3.2 Associative Arrays and array_intersect_assoc()
So far, we’ve been working with indexed arrays. However, array_intersect() can also be used with associative arrays. If you want to preserve the key-value associations, you can use array_intersect_assoc():
php $array1 = ['a' => 1, 'b' => 2, 'c' => 3]; $array2 = ['b' => 2, 'c' => 3, 'd' => 4]; $result = array_intersect_assoc($array1, $array2); print_r($result);
Output:
php Array ( [b] => 2 [c] => 3 )
Here, $result contains the key-value pairs that are common between $array1 and $array2. The function checks both the keys and values for equality.
3.3 array_intersect_key(): Intersection by Keys
If you’re interested in finding the common keys between arrays without considering their values, you can use array_intersect_key():
php $array1 = ['a' => 1, 'b' => 2, 'c' => 3]; $array2 = ['b' => 2, 'c' => 3, 'd' => 4]; $result = array_intersect_key($array1, $array2); print_r($result);
Output:
php Array ( [b] => 2 [c] => 3 )
In this case, $result contains the keys that are common between $array1 and $array2, which are ‘b’ and ‘c’.
4. Practical Examples
Now that we’ve covered the basics and some advanced usage, let’s explore some practical examples of how you can use array_intersect() in real-world scenarios.
4.1 Filtering Data
Suppose you have a list of products and you want to filter out the products that are available in a user’s cart. You can easily achieve this using array_intersect():
php $products = ['product1', 'product2', 'product3', 'product4']; $cart = ['product2', 'product4', 'product5']; $availableProducts = array_intersect($products, $cart); print_r($availableProducts);
Output:
php Array ( [1] => product2 [3] => product4 )
Here, $availableProducts contains the products that are both in the $products list and the user’s $cart.
4.2 User Authentication
In a user authentication system, you might have a list of valid usernames and a list of usernames that users provide during login. You can use array_intersect() to check if the provided username exists in the list of valid usernames:
php $validUsernames = ['user1', 'user2', 'user3']; $providedUsername = 'user2'; if (in_array($providedUsername, $validUsernames)) { echo "Authentication successful!"; } else { echo "Authentication failed."; }
In this example, in_array() is used to check if the provided username exists in the list of valid usernames. While not directly using array_intersect(), this demonstrates how you can use array comparison techniques in real-world scenarios.
5. Tips and Best Practices
When using array_intersect(), keep the following tips and best practices in mind:
- Ensure that all input arrays are of the same type (indexed or associative) for consistent results.
- If you need to compare arrays with different types, consider using array_values() to normalize them first.
- Be aware of the performance implications when comparing large arrays. The function iterates through all elements, so it may be less efficient for extensive datasets.
- Experiment and explore other related functions like array_diff() and array_merge() to solve more complex array manipulation problems.
Conclusion
In this detailed guide, we’ve uncovered the power of PHP’s array_intersect() function. You’ve learned how to compare arrays, find common elements, and use this versatile tool in various real-world scenarios. Armed with this knowledge, you can optimize your PHP applications by efficiently working with arrays and simplifying complex data manipulation tasks. Whether you’re building e-commerce websites, authentication systems, or any other PHP-based application, array_intersect() is a valuable addition to your toolkit, making array comparison a breeze.
Start using array_intersect() today and unlock its potential in your PHP projects!
Table of Contents
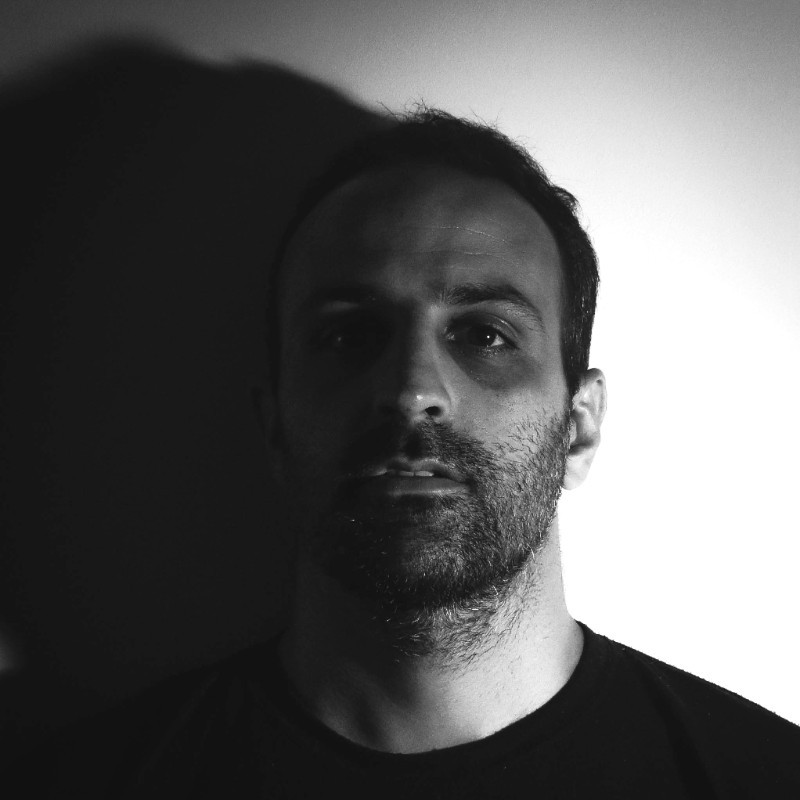
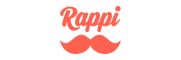