Making Sense of PHP’s array_keys() Function
In the world of web development, PHP (Hypertext Preprocessor) stands as one of the most popular scripting languages. It’s known for its versatility and capability to handle various tasks, from simple webpage interactions to complex data manipulations. One of the key aspects of PHP is its array handling functions, which enable developers to manage data efficiently. In this guide, we’ll delve into one such function – array_keys() – an essential tool for extracting keys from arrays.
Table of Contents
1. Understanding the Basics
Before we dive into the specifics, let’s grasp the fundamental concept of arrays in PHP. An array is a data structure that stores a collection of values, each identified by a unique key. Think of it as a container that holds related information in an organized manner. The array_keys() function allows us to extract these keys, providing a helpful way to access, manipulate, and analyze array contents.
1.1. The Syntax
Here’s the basic syntax of the array_keys() function:
php array array_keys ( array $array [, mixed $search_value = null [, bool $strict = false ]] )
- $array: The input array from which keys will be extracted.
- $search_value (optional): If provided, only keys associated with this value will be returned.
- $strict (optional): If set to true, the function will perform a strict type comparison during the search.
2. Extracting All Keys
At its core, array_keys() can be used to simply extract all keys from an array. This is particularly useful when you want to work with keys independently of their corresponding values. Consider the following example:
php $fruits = array("apple" => "red", "banana" => "yellow", "orange" => "orange"); $keys = array_keys($fruits); // Output: Array ( [0] => apple [1] => banana [2] => orange )
In this example, the $fruits array contains color information for different fruits. By applying array_keys(), we obtain an array of the keys: “apple”, “banana”, and “orange”.
3. Filtering Keys by Value
Often, you might need to retrieve keys based on the values they are associated with. This is where the optional $search_value parameter comes into play. By providing a specific value, you instruct PHP to only return the keys that have that value associated with them.
php $grades = array("Alice" => "A", "Bob" => "B", "Charlie" => "A", "David" => "C"); $excellent_students = array_keys($grades, "A"); // Output: Array ( [0] => Alice [1] => Charlie )
In this case, the $excellent_students array holds the keys “Alice” and “Charlie” because they are associated with the value “A” in the $grades array.
4. Utilizing Strict Comparison
The $strict parameter is another dimension that affects the behavior of array_keys(). When set to true, it enforces a strict type comparison during the search. This means that not only the values but also the types must match for the keys to be included in the result.
php $data = array("42", 42); $strict_comparison = array_keys($data, 42, true); // Output: Array ( )
In this example, since the second element of the $data array is a string “42”, and the strict comparison is set, the function doesn’t consider the integer 42 as a match.
5. Practical Applications
Understanding the array_keys() function is crucial for efficient array manipulation in various scenarios. Here are some practical applications where this function shines:
5.1. Array Key Modification
Let’s say you have an array of settings for a website, and you want to update a specific setting. By extracting the keys with array_keys(), you can easily locate the key you need to modify, even if the array structure evolves over time.
php $site_settings = array("theme" => "blue", "language" => "en", "logo" => "logo.png"); $key_to_modify = "theme"; if (in_array($key_to_modify, array_keys($site_settings))) { $site_settings[$key_to_modify] = "green"; }
5.2. Checking Array Consistency
When working with larger datasets, you might encounter arrays where keys should follow a specific pattern or format. array_keys() can help you ensure that the keys conform to your expectations.
php $invoice = array("INV-2023-001" => 100.00, "INV-2023-002" => 150.00, "INV-2023-003" => 200.00); $expected_keys = array_keys($invoice); $actual_keys = array_keys($invoice); if ($expected_keys === $actual_keys) { echo "Invoice keys are consistent."; } else { echo "Invoice keys are not consistent."; }
5.3. Data Extraction from Multidimensional Arrays
Working with multidimensional arrays is common in PHP projects. The array_keys() function can help you navigate these complex structures and extract specific keys based on your needs.
php $students = array( "Alice" => array("math" => 85, "science" => 90), "Bob" => array("math" => 75, "science" => 85), "Charlie" => array("math" => 95, "science" => 80) ); $math_scores = array(); foreach ($students as $student => $scores) { if (in_array("math", array_keys($scores))) { $math_scores[$student] = $scores["math"]; } }
Conclusion
Mastering PHP’s array_keys() function opens up a world of possibilities for efficient array manipulation. By understanding its syntax, optional parameters, and practical applications, you gain the ability to extract, filter, and manipulate array keys effortlessly. From modifying settings to ensuring data consistency and handling multidimensional arrays, array_keys() is an indispensable tool in every PHP developer’s toolkit. So, next time you find yourself needing to work with array keys, remember the power and flexibility that array_keys() brings to your code.
Table of Contents
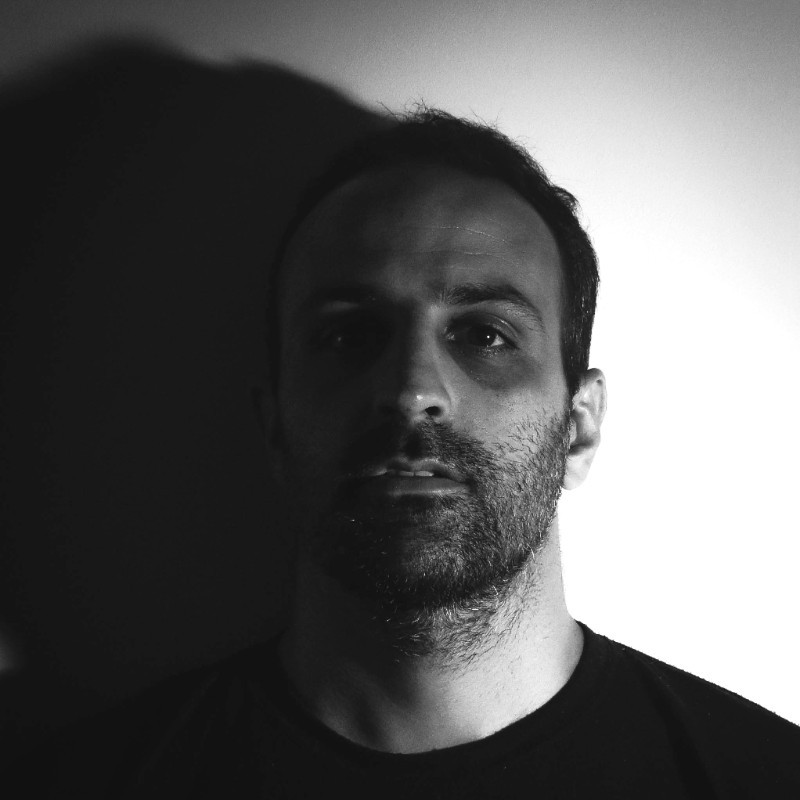
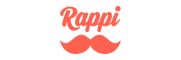