Mastering PHP’s array_merge() Function
Arrays are fundamental data structures in PHP, allowing developers to store and manipulate collections of data. One of the most versatile functions for working with arrays is array_merge(). In this tutorial, we’ll dive deep into mastering the array_merge() function, exploring its various use cases, nuances, and best practices.
1. Understanding the Basics
At its core, the array_merge() function is used to combine two or more arrays into a single array. This can be incredibly handy when you want to consolidate data from multiple sources or build more complex data structures. Let’s start by understanding the basic syntax of the array_merge() function:
php $result = array_merge($array1, $array2, ...);
Here, $array1, $array2, and so on are the arrays you want to merge. The function takes these arrays as arguments and returns a new array containing the merged elements. It’s important to note that the original arrays remain unchanged.
2. Merging Indexed Arrays
Indexed arrays, where elements are identified by numerical indices, are the simplest form of arrays. Let’s say you have two indexed arrays, and you want to combine them into one array. Here’s how you can do it using array_merge():
php $fruits = array("apple", "banana", "orange"); $moreFruits = array("grape", "kiwi"); $combinedFruits = array_merge($fruits, $moreFruits); print_r($combinedFruits);
In this example, the output will be:
csharp Array ( [0] => apple [1] => banana [2] => orange [3] => grape [4] => kiwi )
As you can see, the elements from both arrays have been successfully merged into a single array.
3. Merging Associative Arrays
Associative arrays use named keys to identify elements, allowing for more descriptive data structures. When merging associative arrays, it’s important to understand how array_merge() handles duplicate keys. Let’s take a look at an example:
php $userInfo = array( "name" => "John Doe", "age" => 30, "email" => "john@example.com" ); $additionalInfo = array( "age" => 31, "city" => "New York" ); $mergedInfo = array_merge($userInfo, $additionalInfo); print_r($mergedInfo);
The output will be:
less Array ( [name] => John Doe [age] => 31 [email] => john@example.com [city] => New York )
Here, notice that the age key appears in both arrays. The array_merge() function prioritizes the values from the second array when there are duplicate keys.
4. Preserving Numeric Keys
In some scenarios, you might want to preserve numeric keys when merging arrays. To achieve this, you can use the + operator or the array_merge() function in combination with the + operator. Let’s see how this works:
php $array1 = array(0 => "apple", 1 => "banana"); $array2 = array(1 => "orange", 2 => "kiwi"); $combined = $array1 + $array2; print_r($combined); $merged = array_merge($array1, $array2); print_r($merged);
The output will be:
csharp Array ( [0] => apple [1] => banana ) Array ( [0] => apple [1] => banana [2] => orange [3] => kiwi )
As shown in the example, the + operator preserves numeric keys, while the array_merge() function reindexes numeric keys to maintain a continuous sequence.
5. Merging Multidimensional Arrays
PHP also supports multidimensional arrays, which are arrays containing other arrays as elements. Merging multidimensional arrays can be a bit more complex, but the array_merge() function handles it gracefully. Consider the following example:
php $array1 = array( "fruits" => array("apple", "banana"), "colors" => array("red", "yellow") ); $array2 = array( "fruits" => array("orange", "kiwi"), "numbers" => array(1, 2, 3) ); $merged = array_merge_recursive($array1, $array2); print_r($merged);
The output will be:
csharp Array ( [fruits] => Array ( [0] => apple [1] => banana [2] => orange [3] => kiwi ) [colors] => Array ( [0] => red [1] => yellow ) [numbers] => Array ( [0] => 1 [1] => 2 [2] => 3 ) )
In this example, the array_merge_recursive() function is used to merge the multidimensional arrays. It preserves the nested arrays and their elements while combining them.
6. Best Practices and Considerations
While array_merge() is a powerful tool, it’s essential to be aware of a few considerations to ensure you use it effectively:
- Duplicate Keys: As mentioned earlier, if the same key exists in multiple arrays, the value from the last array takes precedence. Keep this in mind to avoid unintentional data overwriting.
- Reindexed Keys: When merging indexed arrays, the resulting array has continuous numeric keys. Be cautious if you rely on specific keys for any reason.
- Multidimensional Arrays: Use array_merge_recursive() when merging multidimensional arrays. It ensures that nested arrays are properly preserved during the merge.
- Performance: While array_merge() is efficient for small arrays, it might not be the best choice for large arrays. For larger datasets, consider using alternative approaches like iterating through arrays or using specialized merging functions.
Conclusion
Mastering the array_merge() function opens up a world of possibilities when working with arrays in PHP. Whether you’re dealing with indexed arrays, associative arrays, or even multidimensional arrays, understanding how to effectively merge them is crucial for building robust applications. By following the examples and best practices outlined in this tutorial, you’ll be well-equipped to harness the power of array_merge() and create complex data structures with ease. Remember to keep an eye on duplicate keys, reindexed keys, and performance considerations to make the most out of this versatile array manipulation tool. Happy coding!
Table of Contents
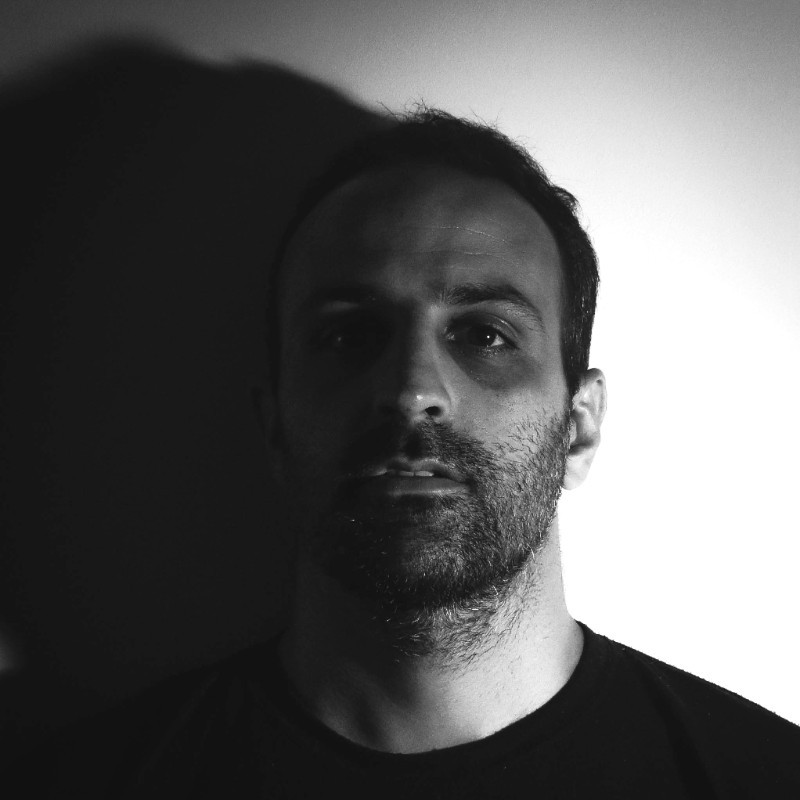
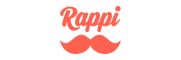