The Power of PHP’s array_pad() Function
If you’re a PHP developer, you’re likely no stranger to arrays. They are the workhorses of many PHP applications, used for storing and manipulating data efficiently. PHP provides an array of functions to work with arrays, and one such function that often goes unnoticed but is incredibly useful is array_pad().
Table of Contents
In this blog, we’ll delve into the depths of PHP’s array_pad() function, exploring its capabilities, use cases, and why it’s an essential tool in your PHP toolkit.
1. Understanding array_pad()
1.1. What is array_pad()?
PHP’s array_pad() function allows you to pad an array to a specified length with a certain value. This means you can expand or shrink an array as needed, ensuring it contains a specified number of elements.
The basic syntax of array_pad() is as follows:
php array_pad(array $array, int $size, mixed $value)
- $array: The input array that you want to pad.
- $size: The new size of the array after padding.
- $value: The value to use for padding.
array_pad() returns a new array with the specified size and filled with the specified value.
2. Examples of array_pad()
Let’s dive straight into some examples to understand how array_pad() works:
Example 1: Padding an Array with Zeros
php $originalArray = [1, 2, 3]; $paddedArray = array_pad($originalArray, 5, 0); print_r($paddedArray);
Output:
csharp Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 0 [4] => 0 )
In this example, we pad the $originalArray with zeros to make it a length of 5. As a result, the function adds two zeros to the end of the array.
Example 2: Truncating an Array
php $originalArray = [1, 2, 3, 4, 5, 6]; $truncatedArray = array_pad($originalArray, 3, null); print_r($truncatedArray);
Output:
csharp Array ( [0] => 1 [1] => 2 [2] => 3 )
In this example, we use array_pad() to reduce the length of $originalArray to 3 by filling it with null values. As a result, the array is truncated to the specified size.
3. Use Cases for array_pad()
3.1. Ensuring Consistent Array Lengths
In many scenarios, you might be working with arrays of data where you need to ensure that all arrays have the same length. This is crucial for operations like matrix manipulations or when preparing data for tabular display.
php $students = ["Alice", "Bob", "Charlie"]; $grades = [90, 85, 88, 92]; // Ensure both arrays have the same length $students = array_pad($students, count($grades), "");
Now, both $students and $grades arrays have the same length, and you can process them together reliably.
3.2. Creating Default Configurations
Imagine you are building a configuration management system, and you want to ensure that all configuration arrays have the same set of keys. array_pad() can help you achieve this by adding default values for missing keys.
php $config = [ "database_host" => "localhost", "database_username" => "root", ]; $defaultConfig = [ "database_host" => "localhost", "database_port" => 3306, "database_username" => "root", "database_password" => "", ]; // Ensure $config has all keys from $defaultConfig $config = array_pad($config, count($defaultConfig), "");
Now, $config will contain all the keys from $defaultConfig, even if they were missing initially.
3.3. Pagination with Fixed Results
When implementing pagination in your PHP application, you may need to ensure that each page displays the same number of items. array_pad() can help you achieve this by filling the last page with empty elements if needed.
php $page = 2; // Current page number $itemsPerPage = 10; $totalItems = 28; $startIndex = ($page - 1) * $itemsPerPage; $endIndex = min($startIndex + $itemsPerPage, $totalItems); $itemsOnPage = array_slice($items, $startIndex, $endIndex - $startIndex); $itemsOnPage = array_pad($itemsOnPage, $itemsPerPage, null);
This code ensures that each page displays exactly $itemsPerPage items, even if the last page has fewer items.
4. Advanced Usage of array_pad()
4.1. Dynamic Padding Values
The value you use for padding doesn’t have to be static. It can be dynamically generated based on certain conditions. For example, let’s say you want to pad an array with values that increment sequentially from a starting value.
php $startValue = 100; $originalArray = [1, 2, 3]; $newSize = 5; $paddingArray = array_pad([], $newSize - count($originalArray), function ($index) use ($startValue) { return $startValue + $index; }); $resultArray = array_merge($originalArray, $paddingArray); print_r($resultArray);
Output:
csharp Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 100 [4] => 101 )
In this example, we dynamically generate the padding values using a closure, ensuring that they increment sequentially from $startValue.
4.2. Combining array_pad() with Other Array Functions
array_pad() can be combined with other array functions to achieve more complex operations. For instance, you can use it to create a function that inserts elements at specific positions within an array.
php function insertElementAtPosition(array $array, $element, $position) { $length = count($array); if ($position >= 0 && $position <= $length) { $array = array_pad($array, $length + 1, null); for ($i = $length; $i > $position; $i--) { $array[$i] = $array[$i - 1]; } $array[$position] = $element; } return $array; } $originalArray = [1, 2, 4, 5]; $elementToInsert = 3; $positionToInsert = 2; $newArray = insertElementAtPosition($originalArray, $elementToInsert, $positionToInsert); print_r($newArray);
Output:
csharp Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
Here, we use array_pad() in combination with a custom function to insert an element at a specific position within an array.
Conclusion
PHP’s array_pad() function might not be as glamorous as some of its counterparts, but it’s a valuable tool in your PHP arsenal. Whether you need to ensure consistent array lengths, create default configurations, or implement advanced array operations, array_pad() can help you simplify your code and improve its readability.
Next time you find yourself working with arrays in PHP and facing issues related to array length or structure, remember the power of array_pad(). It’s a versatile function that can make your coding tasks more efficient and maintainable. So, go ahead and leverage its capabilities to enhance your PHP projects and streamline your development process.
Table of Contents
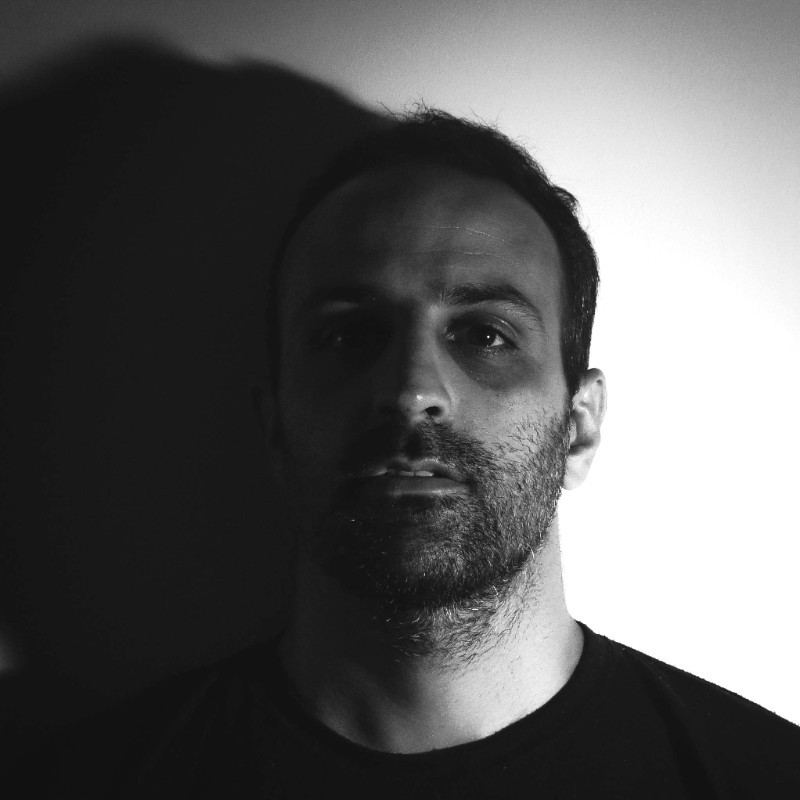
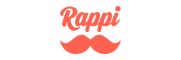