PHP’s array_pop() Function: A Comprehensive Look
PHP is a versatile and widely-used programming language that provides a rich set of functions for working with arrays. Arrays are fundamental data structures used to store collections of data, and PHP offers various functions to manipulate and interact with arrays efficiently. One such function is array_pop(), which allows you to remove and retrieve the last element of an array.
Table of Contents
In this comprehensive guide, we will dive deep into PHP’s array_pop() function. You will learn how to use it effectively, explore practical examples, and understand best practices for incorporating it into your PHP projects.
1. Understanding array_pop()
Before we delve into the details, let’s grasp the basic concept of array_pop(). This function is designed to perform two primary tasks:
- Remove the last element from an array.
- Return the removed element.
1.1. Syntax
The syntax for array_pop() is straightforward:
php array_pop(array &$array): mixed
Here’s what the parameters mean:
- array (required): The input array from which you want to remove the last element. It is passed by reference, which means the original array is modified.
- Return Value: array_pop() returns the last element of the array, or null if the array is empty.
2. Using array_pop()
Now that we have a clear understanding of the syntax, let’s explore how to use array_pop() effectively in various scenarios.
2.1. Removing the Last Element
php $fruits = ["apple", "banana", "cherry", "date"]; $lastFruit = array_pop($fruits); // $fruits now contains ["apple", "banana", "cherry"] // $lastFruit contains "date"
In this example, we first define an array $fruits containing four elements. We then use array_pop($fruits) to remove the last element, which is “date,” from the array. The removed element is stored in the variable $lastFruit.
2.2. Handling Empty Arrays
It’s essential to consider edge cases when working with arrays. If you attempt to use array_pop() on an empty array, it will return null. To avoid potential issues, you can check if the array is empty before using array_pop().
php $emptyArray = []; $lastElement = array_pop($emptyArray); if ($lastElement === null) { echo "The array is empty."; }
In this example, we start with an empty array $emptyArray. We then call array_pop($emptyArray) and check if the result is null. If it is, we display a message indicating that the array is empty.
2.3. Practical Applications
array_pop() is particularly useful in scenarios where you need to process elements in reverse order or when you want to manipulate the last element before removing it. Let’s explore a few practical use cases:
2.3.1. Processing a Stack
A common use case for array_pop() is implementing a stack data structure. In a stack, elements are added and removed from one end, known as the “top” of the stack. Using array_pop(), you can easily implement a stack in PHP.
php $stack = []; // Push elements onto the stack array_push($stack, "item1"); array_push($stack, "item2"); array_push($stack, "item3"); // Pop elements from the stack $topItem = array_pop($stack); // "item3" $secondItem = array_pop($stack); // "item2"
In this example, we start with an empty array $stack. We push elements onto the stack using array_push(), and then we pop elements from the stack using array_pop(). This simulates the behavior of a stack data structure.
2.3.2. Rotating Elements
You can use array_pop() in combination with other array manipulation functions to implement rotation of elements in an array.
php $numbers = [1, 2, 3, 4, 5]; // Rotate the array by removing and reinserting the last element $lastNumber = array_pop($numbers); // Remove the last element array_unshift($numbers, $lastNumber); // Reinsert it at the beginning // The array is now [5, 1, 2, 3, 4]
In this example, we start with an array of numbers and use array_pop() to remove the last element. We then use array_unshift() to insert the removed element at the beginning of the array, effectively rotating the elements.
2.3.3. Implementing a Queue
While PHP has a built-in array_shift() function for working with queues, you can also use array_pop() in certain scenarios to implement a queue data structure.
php $queue = []; // Enqueue elements array_push($queue, "item1"); array_push($queue, "item2"); array_push($queue, "item3"); // Dequeue elements $nextItem = array_pop($queue); // "item3" $secondItem = array_pop($queue); // "item2"
In this example, we use array_push() to enqueue elements onto the queue and array_pop() to dequeue elements from the queue, mimicking the behavior of a queue data structure.
3. Tips and Best Practices
To make the most of array_pop() and ensure efficient and error-free code, consider the following tips and best practices:
3.1. Check for an Empty Array
Always check if the array is empty before using array_pop() to prevent errors. You can use the empty() function or check the array’s length using count().
php if (!empty($myArray)) { $lastElement = array_pop($myArray); } else { // Handle the case when the array is empty }
3.2. Store the Result
Store the result of array_pop() in a variable to avoid unintentionally modifying the original array.
php $lastElement = array_pop($myArray);
3.3. Use in Combination with Other Functions
array_pop() can be powerful when used in combination with other array manipulation functions like array_push(), array_unshift(), and array_shift(). Explore these functions to implement complex data structures and algorithms.
3.4. Document Your Code
If you are working on a project with multiple team members or sharing your code with others, it’s essential to document your usage of array_pop() and its purpose within your codebase. This will make your code more maintainable and understandable.
Conclusion
PHP’s array_pop() function is a valuable tool for manipulating arrays in various ways. Whether you need to remove the last element, implement a stack or queue, or perform other array-related operations, array_pop() can simplify your code and make it more efficient.
By understanding the syntax, usage, and best practices of array_pop(), you can leverage its capabilities to enhance your PHP projects. Remember to check for empty arrays, store results appropriately, and explore combinations with other array functions to unleash the full potential of array_pop() in your PHP applications.
Incorporate these tips and techniques into your coding practices, and you’ll find that array_pop() becomes an indispensable part of your PHP development toolkit. Happy coding!
Table of Contents
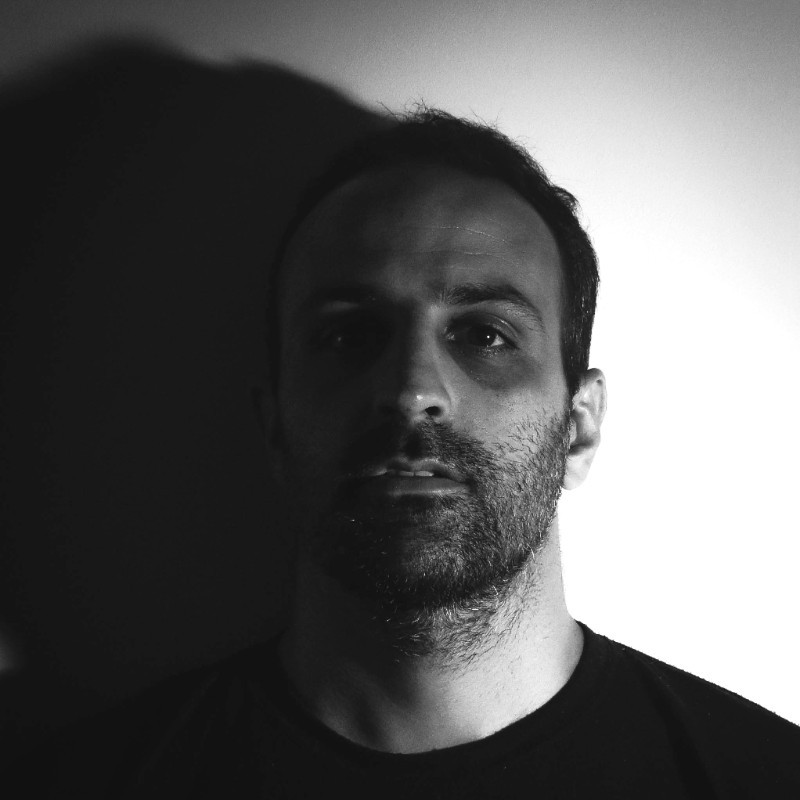
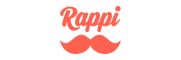