PHP’s array_push() Function: A Comprehensive Guide
Introduction to array_push()
PHP offers a wide range of functions to handle arrays, one of which is array_push(). This function is widely used to add elements to the end of an array, making it an essential tool for developers who frequently manipulate arrays in their applications. In this guide, we’ll explore the syntax, usage, and best practices of array_push() to help you master this function.
What is array_push()?
The array_push() function adds one or more elements to the end of an array. It accepts the array to be modified and the elements to be added as its arguments. The function returns the new number of elements in the array after the addition.
Syntax
```php int array_push(array &$array, mixed $value1, mixed $value2, ..., mixed $valueN) ```
- array: The array to which elements will be added. This parameter is passed by reference.
- value1, value2, …, valueN: The values to add to the array.
Basic Example
```php <?php $fruits = ['apple', 'banana']; array_push($fruits, 'cherry', 'date'); print_r($fruits); ?> ```
Output:
``` Array ( [0] => apple [1] => banana [2] => cherry [3] => date ) ```
In this example, the array_push() function adds ‘cherry’ and ‘date’ to the $fruits array.
Advanced Usage of array_push()
While the basic usage of array_push() is straightforward, it can also be used in more complex scenarios. For instance, it can handle adding elements from one array to another or be used in conditional logic.
Adding Elements from Another Array
```php <?php $array1 = ['apple', 'banana']; $array2 = ['cherry', 'date']; array_push($array1, ...$array2); print_r($array1); ?> ```
Output:
``` Array ( [0] => apple [1] => banana [2] => cherry [3] => date ) ```
In this example, the elements of $array2 are unpacked and added to $array1 using the spread operator (…).
Conditional Element Addition
```php <?php $numbers = [1, 2, 3]; if (count($numbers) < 5) { array_push($numbers, 4, 5); } print_r($numbers); ?> ```
Output:
``` Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 ) ```
Here, elements 4 and 5 are only added if the length of the $numbers array is less than 5.
Best Practices
Passing by Reference
One key aspect of array_push() is that it modifies the array passed to it by reference. This means the original array is altered, which is useful for avoiding the overhead of copying large arrays. However, it also means you should be cautious when using array_push() within functions or loops to avoid unintended side effects.
Use with Indexed Arrays
array_push() is primarily designed for indexed arrays. If you use it with associative arrays, the numeric indexes will continue to increment, potentially leading to unexpected behavior. It’s best to use other methods like $array[] = $value when dealing with associative arrays.
Efficiency Considerations
While array_push() is a convenient way to add elements, it is not the most efficient method if you’re only adding a single element. In such cases, using $array[] = $value is slightly faster as it doesn’t involve a function call overhead.
Conclusion
The array_push() function is a versatile and commonly used tool in PHP for adding elements to arrays. Whether you’re working with simple indexed arrays or handling more complex data structures, understanding how to use this function efficiently can significantly streamline your coding process.
Further Reading
Table of Contents
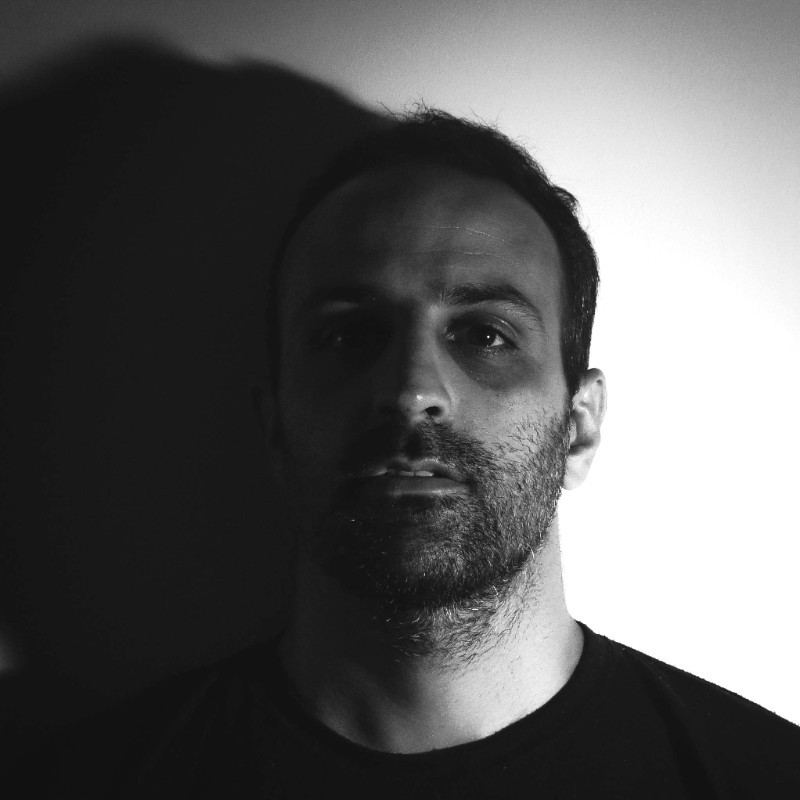
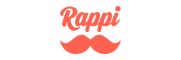