Demystifying PHP’s array_push() Function
Arrays are an essential part of programming, allowing us to store and manage collections of data efficiently. In PHP, one of the most versatile and commonly used programming languages for web development, arrays play a crucial role in managing data. Among the many functions available to manipulate arrays, array_push() stands out as a convenient tool for adding elements to the end of an array. In this blog post, we will demystify the array_push() function, exploring its usage, benefits, and providing code samples to illustrate its capabilities.
Table of Contents
1. Understanding the Basics of array_push()
When working with arrays, the need to add new elements dynamically is a common scenario. PHP’s array_push() function simplifies this process by allowing you to effortlessly append one or more elements to the end of an array. Its syntax is straightforward:
php array_push(array $array, mixed ...$values): int
- $array: The input array to which you want to add elements.
- $values: One or more values you want to append to the array.
The function returns the new number of elements in the array after the addition.
2. Advantages of Using array_push()
2.1. Simplicity and Readability
One of the main advantages of array_push() is its simplicity. The function’s name itself clearly conveys its purpose, making your code more readable and understandable. This is particularly useful when collaborating with other developers, as it reduces the time required to comprehend the codebase.
2.2. Adding Multiple Elements at Once
Unlike other methods that require multiple lines of code to add multiple elements, array_push() allows you to add several elements in a single line. This not only simplifies the code but also improves its efficiency, as fewer lines generally lead to better performance.
2.3. Integration with Other Functions
array_push() works seamlessly with other array manipulation functions, enabling you to create more complex operations without sacrificing code elegance. This integration enhances your ability to manipulate arrays and create sophisticated data structures.
3. Examples of array_push() in Action
Let’s dive into some real-world examples to showcase how array_push() can be used effectively.
Example 1: Adding a Single Element
php $fruits = ['apple', 'banana', 'orange']; $newFruit = 'grape'; array_push($fruits, $newFruit); // Resulting $fruits array: ['apple', 'banana', 'orange', 'grape']
In this example, the array_push() function appends the value ‘grape’ to the end of the $fruits array.
Example 2: Adding Multiple Elements
php $colors = ['red', 'green']; $newColors = ['blue', 'yellow']; array_push($colors, ...$newColors); // Resulting $colors array: ['red', 'green', 'blue', 'yellow']
By using the spread operator (…$newColors), you can add multiple elements from the $newColors array to the end of the $colors array in a single function call.
Example 3: Building a Dynamic List
php $tasks = []; array_push($tasks, 'Task 1', 'Task 2', 'Task 3'); // Resulting $tasks array: ['Task 1', 'Task 2', 'Task 3']
Here, the array_push() function is used to create a dynamic list of tasks. As new tasks arise, you can easily add them to the $tasks array without repetitive code.
4. Considerations and Alternatives
While array_push() offers a convenient way to add elements to the end of an array, there are a few considerations to keep in mind:
4.1. Performance Implications
For adding a single element, using the assignment operator (=) to directly append an element to an array is generally more performant than using array_push(). However, the difference is negligible for small arrays and doesn’t significantly impact overall application performance.
4.2. Alternative Syntax: $array[] = $value
A common alternative to array_push() for adding a single element is the array append syntax, where you directly assign a value to the next index of the array:
php $animals = ['cat', 'dog']; $animals[] = 'elephant'; // Resulting $animals array: ['cat', 'dog', 'elephant']
This syntax can be more concise and equally readable in certain scenarios.
4.3. array_push() vs. array_unshift()
While array_push() adds elements to the end of an array, array_unshift() is used to prepend elements to the beginning. Depending on your specific use case, you might find one more suitable than the other.
Conclusion
The array_push() function in PHP is a powerful tool for manipulating arrays, offering a simple and effective way to add elements to the end of an array. Its ease of use, compatibility with other array functions, and ability to handle multiple elements at once make it an invaluable asset in your array manipulation toolbox. By understanding the basics and exploring real-world examples, you can confidently incorporate array_push() into your projects and enhance your PHP programming skills.
Incorporating dynamic elements into arrays is a fundamental aspect of programming, and array_push() streamlines this process, contributing to cleaner and more efficient code. Whether you’re building a dynamic list, managing user inputs, or processing data, mastering the array_push() function unlocks new possibilities for your PHP applications. So go ahead, experiment with this versatile function, and elevate your array manipulation prowess to new heights. Happy coding!
Table of Contents
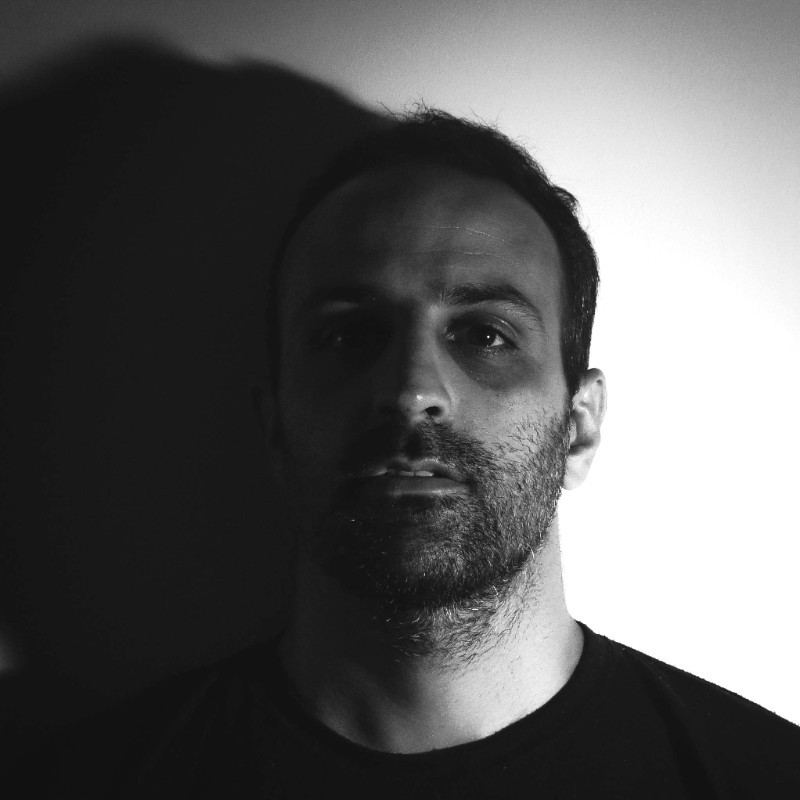
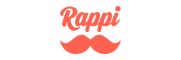