Demystifying PHP’s array_rand() Function
PHP is a versatile and widely used programming language that offers a plethora of built-in functions to make developers’ lives easier. One such function is array_rand(), which allows you to randomly select elements from an array. While it may seem straightforward, there are some intricacies to consider when using this function effectively. In this blog post, we will demystify PHP’s array_rand() function, exploring its syntax, use cases, and best practices.
Table of Contents
1. Understanding array_rand()
Before diving into the details, let’s get a basic understanding of what the array_rand() function does. Essentially, it allows you to pick one or more random elements from an array. This can be particularly useful in scenarios where you need to shuffle elements, select a random winner, or create randomized content.
1.1. Syntax
The syntax of array_rand() is quite simple:
php mixed array_rand ( array $array [, int $num = 1 ] )
- $array: The input array from which you want to select random elements.
- $num: An optional parameter specifying the number of random elements to select. By default, it’s set to 1.
Now, let’s explore various use cases and scenarios where array_rand() can come in handy.
2. Selecting a Single Random Element
The most common use case for array_rand() is selecting a single random element from an array. Let’s look at a practical example:
php $fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']; $randomFruit = $fruits[array_rand($fruits)]; echo "Today's random fruit is: $randomFruit";
In this example, array_rand($fruits) returns a random index from the $fruits array, and we use that index to retrieve the corresponding fruit. This way, each time you run the script, you’ll get a different random fruit.
3. Selecting Multiple Random Elements
You can also use array_rand() to select multiple random elements from an array. Simply provide the desired number of elements as the second argument:
php $colors = ['red', 'blue', 'green', 'yellow', 'purple']; $randomColors = []; $numColors = 3; $randomKeys = array_rand($colors, $numColors); foreach ($randomKeys as $key) { $randomColors[] = $colors[$key]; } echo "Random colors: " . implode(', ', $randomColors);
In this example, we specify that we want three random colors from the $colors array. The array_rand() function returns an array of random keys, which we then use to fetch the corresponding colors from the original array.
4. Handling Edge Cases
When working with array_rand(), it’s essential to consider edge cases. For instance, what happens when you pass an empty array or a non-array variable to the function?
4.1. Handling Empty Arrays
If you attempt to use array_rand() on an empty array, it will result in an error. To avoid this, you should check if the array is empty before calling the function:
php $emptyArray = []; if (!empty($emptyArray)) { $randomElement = $emptyArray[array_rand($emptyArray)]; } else { echo "The array is empty!"; }
By adding this conditional check, you prevent the function from being called on an empty array, which would lead to an undefined index error.
4.2. Handling Non-Arrays
Another important consideration is ensuring that the input is an array. If you attempt to use array_rand() on a non-array variable, you will encounter a warning. To address this issue, use the is_array() function to validate the input:
php $notAnArray = 'This is not an array'; if (is_array($notAnArray)) { $randomElement = $notAnArray[array_rand($notAnArray)]; } else { echo "The input is not an array!"; }
By checking whether the input is an array, you can prevent unexpected errors in your code.
5. Advanced Usage: Shuffling an Array
array_rand() can also be used to shuffle the elements of an array randomly. While there are dedicated functions like shuffle() for this purpose, you can achieve the same result using array_rand():
php $numbers = [1, 2, 3, 4, 5]; $shuffledNumbers = []; while (!empty($numbers)) { $key = array_rand($numbers); $shuffledNumbers[] = $numbers[$key]; unset($numbers[$key]); } print_r($shuffledNumbers);
In this example, we repeatedly select a random element from the $numbers array and add it to the $shuffledNumbers array while removing it from the original array using unset(). This process continues until the original array is empty, resulting in a shuffled array.
While this approach works, keep in mind that it’s less efficient than using shuffle() for larger arrays, as shuffle() is optimized for this specific task.
6. Performance Considerations
When using array_rand(), it’s important to be mindful of performance, especially when dealing with large arrays. The function involves generating random numbers, which can become computationally expensive if used excessively.
If you need to select multiple random elements from an array, consider an alternative approach like shuffling the array using shuffle() and then selecting the desired number of elements from the shuffled array. This approach can be more efficient, as shuffling is generally faster than generating multiple random numbers.
7. Use Cases in Web Development
array_rand() can be a valuable tool in web development when you need to introduce randomness into your applications. Here are some practical use cases:
7.1. Randomizing Content
You can use array_rand() to randomize content on your website. For example, you could create a rotating banner that displays different images or messages each time a user visits the page.
php $bannerMessages = ['Welcome to our website!', 'Check out our latest products!', 'Join our newsletter for updates!']; $randomMessage = $bannerMessages[array_rand($bannerMessages)]; echo "<div class='banner'>$randomMessage</div>";
This code snippet selects a random message from an array and displays it in a website banner. Users will see a different message each time they visit the page, adding variety to their experience.
7.2. Random User Recommendations
In e-commerce or social networking platforms, you can use array_rand() to provide users with random product recommendations or suggest new connections or friends.
php $products = ['Product A', 'Product B', 'Product C', 'Product D', 'Product E']; $recommendedProducts = []; $numRecommendations = 3; $randomIndices = array_rand($products, $numRecommendations); foreach ($randomIndices as $index) { $recommendedProducts[] = $products[$index]; } echo "<h2>Recommended Products:</h2>"; echo "<ul>"; foreach ($recommendedProducts as $product) { echo "<li>$product</li>"; } echo "</ul>";
This code snippet selects three random products from an array and displays them as recommendations. Users get a personalized shopping experience with different product suggestions each time.
7.3. Gamification
If you’re developing a game or gamifying your website, array_rand() can help create randomness in game outcomes. For instance, you can use it to determine the outcome of a dice roll or to select a random winner in a contest.
php $diceValues = [1, 2, 3, 4, 5, 6]; $rollResult = $diceValues[array_rand($diceValues)]; echo "You rolled a $rollResult!";
In this example, the array_rand() function simulates a dice roll, providing a random value between 1 and 6.
Conclusion
PHP’s array_rand() function is a versatile tool for introducing randomness into your applications. Whether you need to select random elements from an array, shuffle content, or implement randomization in web development, array_rand() can simplify the process. However, it’s crucial to use it judiciously, considering performance implications and handling edge cases to ensure the reliability of your code. By demystifying array_rand(), you can harness its power to create dynamic and engaging experiences for your users.
Table of Contents
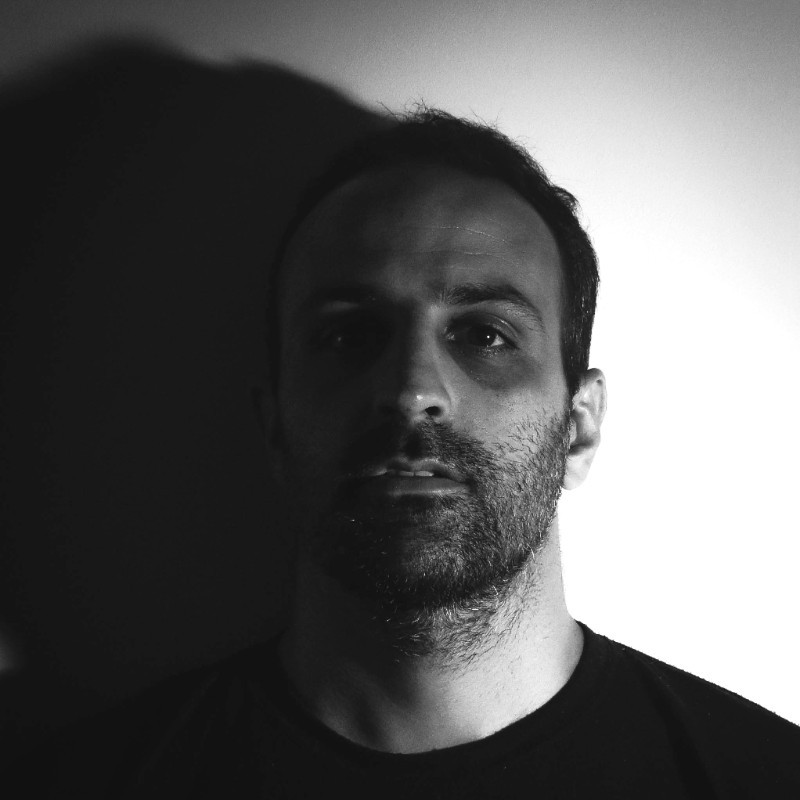
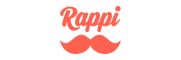