Harnessing the Power of PHP’s array_reduce() Function
In the world of PHP programming, array manipulation is a common task. Whether you’re working with data retrieved from a database, parsing JSON, or dealing with any other type of structured data, you’ll often find yourself needing to iterate through arrays and perform various operations on their elements. PHP provides a wide array of array functions to help you with this, and one of the most powerful and versatile among them is array_reduce().
Table of Contents
1. What is array_reduce()?
array_reduce() is a built-in PHP function that allows you to reduce an array to a single value. It iterates over an array and performs a callback function on each element, accumulating a result along the way. This function can be used for a wide range of tasks, such as summing the elements of an array, finding the maximum or minimum value, or even constructing entirely new data structures based on the original array.
1.1. Syntax
Here’s the basic syntax of array_reduce():
php array_reduce(array $array, callable $callback, mixed $initial = null): mixed
- $array: The input array.
- $callback: A callback function to apply to the elements of the array. This function takes two arguments: the current result and the current array element, and returns a new result.
- $initial (optional): An initial value to start the reduction. If not provided, the first element of the array is used as the initial value.
2. Why Use array_reduce()?
You might be wondering why you should use array_reduce() when PHP offers other array functions like array_map(), array_filter(), and array_walk(). While these functions are useful for specific tasks, array_reduce() stands out for a few reasons:
2.1. Versatility
array_reduce() is incredibly versatile. It can be used to solve a wide variety of problems, from basic arithmetic operations to complex data transformations. You can tailor the callback function to suit your specific needs, making it a powerful tool in your PHP programming toolbox.
2.2. Reduced Memory Consumption
Compared to functions like array_map(), which create a new array as output, array_reduce() can significantly reduce memory consumption because it doesn’t generate an intermediate array. Instead, it accumulates the result step by step, which can be especially beneficial when dealing with large datasets.
2.3. Performance
In some cases, array_reduce() can outperform other array manipulation functions due to its reduced memory usage. This can lead to better overall performance in your PHP applications, particularly when working with extensive datasets.
2.4. Code Clarity
Using array_reduce() can lead to cleaner and more readable code, especially when dealing with complex array transformations or aggregations. It encapsulates the reduction logic in a single function, making your code more self-explanatory.
Now that you understand the advantages of using array_reduce(), let’s explore some practical examples to see how it can simplify your code and solve real-world problems.
3. Practical Examples
Example 1: Summing an Array of Numbers
One of the most common use cases for array_reduce() is calculating the sum of an array of numbers. Let’s say you have an array of integers, and you want to find their sum. Here’s how you can do it using array_reduce():
php $numbers = [1, 2, 3, 4, 5]; $sum = array_reduce($numbers, function ($carry, $number) { return $carry + $number; }); echo $sum; // Output: 15
In this example, the callback function takes two arguments: $carry (the current sum) and $number (the current array element). It adds the current number to the sum and returns the updated sum. The result is the sum of all the numbers in the array.
Example 2: Finding the Maximum Value
You can also use array_reduce() to find the maximum value in an array of numbers:
php $numbers = [42, 19, 67, 35, 88, 10]; $max = array_reduce($numbers, function ($carry, $number) { return $number > $carry ? $number : $carry; }); echo $max; // Output: 88
In this example, the callback function compares each number with the current maximum ($carry) and updates the maximum value when a larger number is encountered. The result is the maximum value in the array.
Example 3: Grouping Data by Category
Let’s explore a more advanced example where array_reduce() is used to group data by category. Suppose you have an array of products, and each product has a category. You want to organize these products into an associative array, where each category is a key, and the products within that category are stored in an array. Here’s how you can achieve this:
php $products = [ ['name' => 'Laptop', 'category' => 'Electronics'], ['name' => 'Headphones', 'category' => 'Electronics'], ['name' => 'Sneakers', 'category' => 'Fashion'], ['name' => 'T-shirt', 'category' => 'Fashion'], ['name' => 'Guitar', 'category' => 'Music'], ]; $grouped = array_reduce($products, function ($carry, $product) { $category = $product['category']; if (!isset($carry[$category])) { $carry[$category] = []; } $carry[$category][] = $product; return $carry; }, []); print_r($grouped);
In this example, the callback function initializes an empty array for each category encountered in the input data. It then appends each product to the corresponding category’s array. The result is an associative array that groups products by their categories.
These practical examples demonstrate the flexibility and power of array_reduce(). Whether you’re performing basic arithmetic operations or more complex data transformations, this function can handle a wide range of scenarios.
4. Tips and Best Practices
To effectively harness the power of array_reduce(), consider the following tips and best practices:
4.1. Define a Clear Callback Function
Your callback function should have a clear and well-defined purpose. It should take in the current result and the current array element and return an updated result. Naming your variables descriptively can make your code more readable.
4.2. Initialize the Accumulator
In most cases, it’s a good practice to initialize the accumulator (the initial value) explicitly. This ensures that your code behaves predictably, especially when working with empty arrays.
4.3. Handle Edge Cases
Be aware of edge cases, such as empty arrays or unexpected input data. Your callback function should handle these cases gracefully to avoid errors or unexpected behavior.
4.4. Keep Performance in Mind
While array_reduce() can be efficient, it may not always be the fastest option for every scenario. Benchmark your code and consider alternative approaches if performance is a critical concern.
4.5. Document Your Code
Clearly document the purpose of your array_reduce() usage, especially when dealing with complex transformations. This makes it easier for other developers (or your future self) to understand the code’s intent.
Conclusion
PHP’s array_reduce() function is a versatile tool that can simplify your code, improve performance, and help you solve complex array manipulation problems. Whether you’re aggregating data, finding the maximum value, or performing more advanced transformations, array_reduce() is a valuable addition to your PHP programming toolkit. By following best practices and understanding its capabilities, you can harness the full power of this function and write more efficient and readable code.
In summary, embrace the power of array_reduce() and let it streamline your array manipulation tasks, making your PHP code more elegant and efficient.
Table of Contents
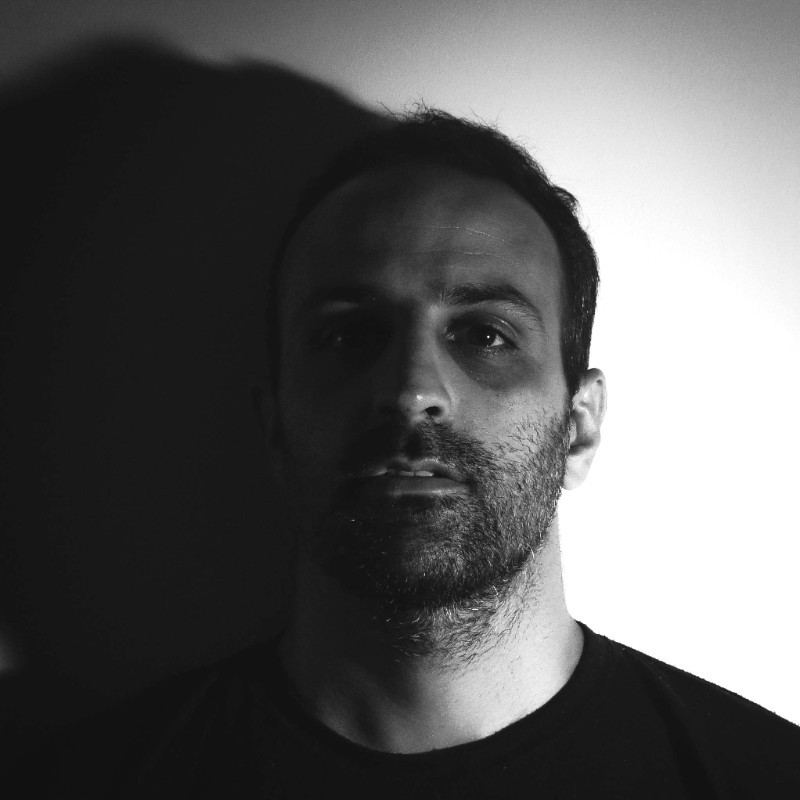
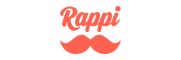