Getting Started with PHP’s array_reverse() Function
PHP, one of the most popular server-side scripting languages, offers a plethora of built-in functions that make it a developer’s favorite for web application development. Among these functions, array_reverse() stands out as a versatile tool for reversing the elements of an array. Whether you’re a seasoned PHP developer or just starting your journey, understanding how to use array_reverse() effectively can simplify array manipulation tasks. In this guide, we’ll explore the ins and outs of the array_reverse() function, including its syntax, parameters, use cases, and some practical examples.
Table of Contents
1. Understanding the Basics
1.1. What is array_reverse()?
The array_reverse() function is a built-in PHP function designed to reverse the order of elements in an array. It takes an array as input and returns a new array with the elements in reverse order. This function is particularly handy when you need to display or work with data in a different order than it originally appears.
1.2. Syntax
The basic syntax for using array_reverse() is as follows:
php array array_reverse ( array $array [, bool $preserve_keys = FALSE ] )
- $array: The input array that you want to reverse.
- $preserve_keys (optional): A boolean parameter that specifies whether to preserve the keys in the resulting array. By default, it’s set to FALSE, meaning that keys will be renumbered sequentially. If set to TRUE, keys will be preserved.
2. Practical Examples
Let’s dive into some practical examples to better understand how to use array_reverse() in different scenarios.
2.1. Reversing a Numeric Array
Suppose you have an array of numbers and you want to reverse their order. Here’s how you can achieve this using array_reverse():
php $numbers = [1, 2, 3, 4, 5]; $reversedNumbers = array_reverse($numbers); print_r($reversedNumbers);
Output:
csharp Array ( [0] => 5 [1] => 4 [2] => 3 [3] => 2 [4] => 1 )
In this example, the original array $numbers is reversed, and the keys are renumbered sequentially by default.
2.2. Preserving Keys
If you want to preserve the keys in the reversed array, you can do so by setting the $preserve_keys parameter to TRUE:
php $fruits = ['apple' => 'red', 'banana' => 'yellow', 'cherry' => 'red']; $reversedFruits = array_reverse($fruits, true); print_r($reversedFruits);
Output:
csharp Array ( [cherry] => red [banana] => yellow [apple] => red )
As you can see, the keys in the $fruits array are preserved in the reversed array.
2.3. Reversing an Associative Array
To reverse an associative array while maintaining the keys, simply pass the $preserve_keys parameter as TRUE:
php $colors = ['red' => 'apple', 'yellow' => 'banana', 'green' => 'kiwi']; $reversedColors = array_reverse($colors, true); print_r($reversedColors);
Output:
csharp Array ( [green] => kiwi [yellow] => banana [red] => apple )
2.4. Reversing a Multidimensional Array
You can also use array_reverse() to reverse multidimensional arrays. Let’s say you have the following multidimensional array:
php $matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ];
To reverse the order of rows in this matrix, you can use a combination of array_reverse() and a loop:
php $reversedMatrix = array_map('array_reverse', $matrix); print_r($reversedMatrix);
Output:
csharp Array ( [0] => Array ( [0] => 7 [1] => 8 [2] => 9 ) [1] => Array ( [0] => 4 [1] => 5 [2] => 6 ) [2] => Array ( [0] => 1 [1] => 2 [2] => 3 ) )
In this example, array_map() is used to apply array_reverse() to each sub-array within the multidimensional array, resulting in a reversed matrix.
3. Common Use Cases
The array_reverse() function can be a valuable tool in various scenarios:
3.1. Pagination
When working with paginated data, you may need to display items in reverse order, such as showing the most recent content first. array_reverse() makes it easy to achieve this by reversing the array of items before displaying them.
php $items = get_paginated_items(); $reversedItems = array_reverse($items); foreach ($reversedItems as $item) { // Display the item }
3.2. Building a Stack
A stack data structure follows the Last-In-First-Out (LIFO) principle. You can use array_reverse() to implement a stack by adding elements to the end of an array and then popping them off in reverse order.
php $stack = []; array_push($stack, 'item1'); array_push($stack, 'item2'); array_push($stack, 'item3'); $reversedStack = array_reverse($stack); while (!empty($reversedStack)) { $item = array_pop($reversedStack); // Process the item }
3.3. Sorting in Descending Order
If you have an array of data that you want to sort in descending order based on certain criteria, you can use array_reverse() after sorting it in ascending order using functions like sort() or usort().
php $numbers = [5, 2, 8, 1, 9]; sort($numbers); // Sort in ascending order $sortedDescending = array_reverse($numbers); print_r($sortedDescending);
Output:
csharp Array ( [0] => 9 [1] => 8 [2] => 5 [3] => 2 [4] => 1 )
3.4. Implementing a Queue
A queue data structure follows the First-In-First-Out (FIFO) principle. You can use array_reverse() to implement a queue by adding elements to the end of an array and dequeuing them from the beginning.
php $queue = []; array_push($queue, 'item1'); array_push($queue, 'item2'); array_push($queue, 'item3'); $dequeuedItem = array_pop(array_reverse($queue)); // Dequeue the first item // Process the dequeued item
4. Tips and Tricks
To make the most of the array_reverse() function, consider these tips and tricks:
4.1. Performance Considerations
While array_reverse() is a convenient function, keep in mind that it creates a new array with reversed elements. If you’re working with large arrays, this can consume significant memory. In such cases, consider alternative approaches, like reversing the array using a loop.
4.2. Reversing Strings
You can also use array_reverse() to reverse a string by converting it to an array of characters, reversing the array, and then converting it back to a string.
php $string = "Hello, World!"; $reversedString = implode('', array_reverse(str_split($string))); echo $reversedString;
Output:
diff !dlroW ,olleH
4.3. Using array_reverse() with Objects
array_reverse() can be used with objects, but it treats them as arrays. If you want to reverse an object’s properties, consider converting it to an associative array first.
5. Check for Valid Arrays
Before using array_reverse(), it’s a good practice to check if the input is a valid array using the is_array() function. This helps prevent unexpected errors.
php if (is_array($data)) { $reversedData = array_reverse($data); } else { // Handle the case when $data is not an array }
Conclusion
PHP’s array_reverse() function is a versatile tool for reversing the elements of arrays, whether they are numeric, associative, or multidimensional. By understanding its syntax, parameters, and use cases, you can simplify array manipulation tasks and make your code more efficient and readable. Whether you’re building a stack, implementing a queue, or just need to reverse the order of data, array_reverse() is a valuable addition to your PHP toolkit. So, go ahead and start harnessing the power of array_reverse() in your PHP projects to take your array manipulation skills to the next level.
Table of Contents
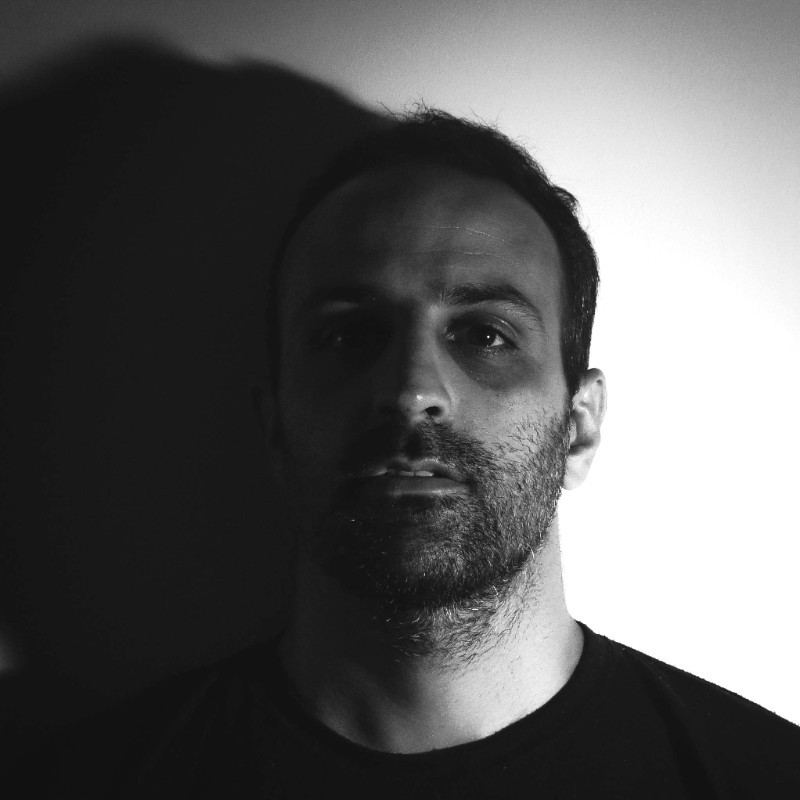
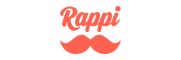