Working with PHP’s array_search() Function: A Practical Guide
PHP is a versatile programming language that provides developers with a wide array of functions and tools to work with arrays effectively. One such function is array_search(), which allows you to search for a specific value within an array and retrieve its corresponding key. This function can be incredibly useful in various scenarios, from finding a user in a database to checking if a particular item exists in a shopping cart. In this practical guide, we will explore how to work with array_search() and provide you with real-world examples and code samples to help you master this essential PHP function.
Table of Contents
1. Understanding the Basics of array_search()
Before we dive into more complex scenarios, let’s start by understanding the basics of the array_search() function. At its core, this function searches for a value within an array and returns the corresponding key if the value is found. If the value is not found, it returns false.
Here’s the basic syntax of array_search():
php array_search($needle, $haystack, $strict);
- $needle: The value you want to search for.
- $haystack: The array in which you want to search.
- $strict (optional): A boolean parameter that specifies whether the search should be strict (both type and value must match). Default is false.
Now, let’s look at a simple example:
php $fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']; $position = array_search('cherry', $fruits); if ($position !== false) { echo 'The key of "cherry" is ' . $position; } else { echo 'Value not found in the array.'; }
In this example, we have an indexed array called $fruits, and we’re using array_search() to find the key of the value ‘cherry’. Since ‘cherry’ is present in the array, it will return the key ‘2’, and our code will output “The key of ‘cherry’ is 2.”
2. Searching for Values in Indexed Arrays
Searching for values in indexed arrays is a common use case for array_search(). Indexed arrays are arrays where keys are numeric and automatically assigned starting from 0. Let’s explore this further with an example:
php $numbers = [10, 20, 30, 40, 50]; $position = array_search(30, $numbers); if ($position !== false) { echo 'The key of 30 is ' . $position; } else { echo 'Value not found in the array.'; }
In this example, we have an indexed array of numbers, and we’re searching for the value ’30’. Since ’30’ is present in the array, the array_search() function will return the key ‘2’, and our code will output “The key of 30 is 2.”
3. Searching for Values in Associative Arrays
Associative arrays are arrays where keys are defined explicitly. Searching for values in associative arrays is slightly different from indexed arrays, as you need to account for the keys as well. Let’s see how it works:
php $person = [ 'first_name' => 'John', 'last_name' => 'Doe', 'age' => 30, 'email' => 'john@example.com', ]; $key = array_search('Doe', $person); if ($key !== false) { echo 'The key of "Doe" is "' . $key . '"'; } else { echo 'Value not found in the array.'; }
In this example, we have an associative array $person containing information about an individual. We’re using array_search() to find the key associated with the value ‘Doe’. Since ‘Doe’ is associated with the key ‘last_name’, our code will output “The key of ‘Doe’ is ‘last_name’.”
4. Handling Non-Strict and Strict Searches
The array_search() function allows you to perform both non-strict and strict searches. In a non-strict search, the function checks for the presence of a value without considering its type. In a strict search, both the type and the value must match for a successful search. You can achieve this by passing a true value as the third parameter to array_search() for strict searching.
Let’s compare non-strict and strict searches with an example:
php $fruits = ['1', '2', '3', 4, 5]; $non_strict = array_search(3, $fruits); $strict = array_search(3, $fruits, true); echo 'Non-Strict Search: ' . ($non_strict !== false ? 'Found at key ' . $non_strict : 'Value not found') . PHP_EOL; echo 'Strict Search: ' . ($strict !== false ? 'Found at key ' . $strict : 'Value not found') . PHP_EOL;
In this example, we have an array $fruits containing a mix of string and integer values. We perform both non-strict and strict searches for the value ‘3’. Here’s the output:
vbnet Non-Strict Search: Found at key 2 Strict Search: Value not found
As you can see, the non-strict search successfully finds the value ‘3’ at key ‘2’ because it doesn’t consider the type. In contrast, the strict search fails because it requires an exact type and value match.
5. Working with Multidimensional Arrays
Multidimensional arrays are arrays that contain other arrays as elements. When dealing with multidimensional arrays, using array_search() becomes slightly more complex because you need to navigate through multiple levels of the array to find your desired value. Let’s explore how to handle this scenario:
php $employees = [ ['id' => 1, 'name' => 'John'], ['id' => 2, 'name' => 'Jane'], ['id' => 3, 'name' => 'Bob'], ]; $searchName = 'Jane'; foreach ($employees as $key => $employee) { $position = array_search($searchName, $employee); if ($position !== false) { echo $searchName . ' found in the sub-array with key ' . $key; break; } } if ($position === false) { echo $searchName . ' not found in the array.'; }
In this example, we have a multidimensional array $employees, where each element is an associative array representing an employee. We want to find the key of the employee with the name ‘Jane’. To do this, we loop through the outer array and use array_search() to search within each sub-array. Once we find a match, we break out of the loop and display the result.
6. Handling Edge Cases
While array_search() is a powerful tool for searching arrays, there are some edge cases to be aware of. One important consideration is that array_search() returns false both when a value is not found and when the value is found at key 0. This can lead to unexpected results, especially in indexed arrays. To avoid this, you should use the !== operator to check for both value and type.
Here’s an example illustrating this edge case:
php $colors = ['red', 'green', 'blue']; $position = array_search('red', $colors); if ($position !== false) { echo 'The key of "red" is ' . $position; } else { echo 'Value not found in the array.'; }
In this example, we’re searching for the value ‘red’ in the $colors array. Since ‘red’ is found at key 0, the array_search() function will return 0, which may be interpreted as false in a loose comparison. To handle this correctly, we use the !== operator to check for both value and type, ensuring that the key 0 is considered a valid result.
Conclusion
PHP’s array_search() function is a valuable tool for searching arrays in a variety of scenarios. Whether you’re working with indexed arrays, associative arrays, or even multidimensional arrays, array_search() can help you find values efficiently. By understanding its basics, handling strict and non-strict searches, and considering edge cases, you can leverage this function to enhance your PHP projects and make your code more robust.
In this guide, we’ve covered the fundamental concepts of array_search() and provided practical examples to help you get started. As you continue to explore PHP and work with arrays, you’ll find this function to be a valuable asset in your development toolkit.
Table of Contents
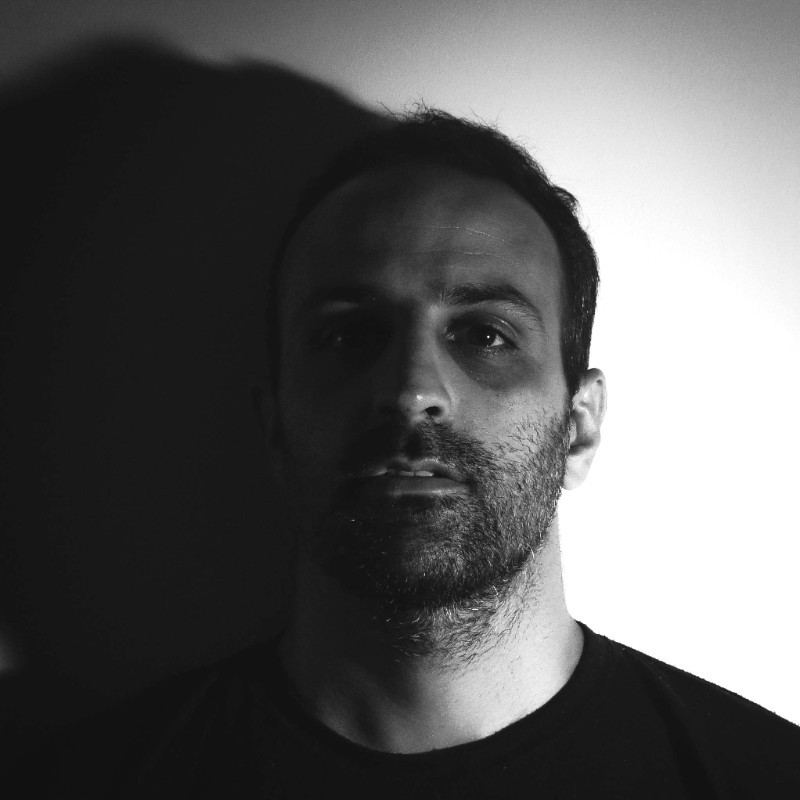
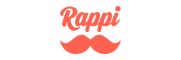