PHP’s array_shift() Function: A Complete Guide
PHP is a versatile and powerful scripting language widely used for web development. One of its core features is its ability to work with arrays efficiently. If you’re a PHP developer, you’re likely familiar with array manipulation. In this guide, we’ll explore one of the essential array functions: array_shift(). This function allows you to remove the first element from an array, making it a valuable tool in your PHP arsenal.
Table of Contents
1. Understanding array_shift()
The array_shift() function in PHP serves a straightforward purpose: it removes and returns the first element from an array. This operation modifies the original array, reducing its length by one. This function takes a single argument, which is the array you want to manipulate. Here’s a basic syntax:
php array_shift($array)
$array: The array from which you want to remove and return the first element.
Let’s dive into the details and explore how you can effectively use array_shift() in your PHP projects.
2. Practical Use Cases
2.1. Removing Unwanted Elements
Suppose you have an array of user data, and you want to remove the first element, which contains information about the administrator. You can achieve this with array_shift():
php $userData = [ ['name' => 'Admin', 'role' => 'Administrator'], ['name' => 'John', 'role' => 'User'], ['name' => 'Jane', 'role' => 'User'], ]; $adminInfo = array_shift($userData);
After executing this code, the $adminInfo variable will contain the information of the administrator, and the $userData array will no longer have the admin’s data.
2.2. Creating Queues
array_shift() is often used to implement simple queue systems. You can add elements to the end of the array and remove them from the beginning, effectively mimicking a first-in, first-out (FIFO) behavior. Here’s a basic example:
php $queue = []; // Enqueue (add to the end) array_push($queue, 'Task 1'); array_push($queue, 'Task 2'); // Dequeue (remove from the beginning) $nextTask = array_shift($queue);
In this scenario, $nextTask will contain ‘Task 1’, and the $queue array will only contain ‘Task 2’.
2.3. Parsing CSV Data
When working with CSV data, you can use array_shift() to extract the header row, which typically contains column names. This allows you to work with the data more efficiently. Here’s an example:
php $csvData = [ ['id', 'name', 'email'], [1, 'John', 'john@example.com'], [2, 'Jane', 'jane@example.com'], ]; $header = array_shift($csvData); // $header now contains ['id', 'name', 'email']
Now, you can easily associate the data rows with their corresponding columns using the extracted header.
3. Handling Edge Cases
3.1. Empty Arrays
It’s important to handle edge cases when working with array_shift(). When you try to shift an element from an empty array, PHP will return null. To avoid unexpected behavior, make sure to check if the array is empty before using array_shift():
php if (!empty($array)) { $firstElement = array_shift($array); } else { // Handle empty array case }
This check prevents errors and ensures your code behaves as expected.
3.2. Associative Arrays
array_shift() primarily works with indexed arrays. If you attempt to use it with an associative array, it will return null and not modify the original array. If you need to remove and retrieve an element from an associative array, consider using other array functions like array_shift_assoc():
php function array_shift_assoc(&$array) { $element = reset($array); unset($array[key($array)]); return $element; }
This custom function resets the array’s internal pointer, retrieves the first element, unsets it by key, and returns the element.
4. Modifying the Original Array
Keep in mind that array_shift() directly modifies the original array. If you want to preserve the original array and work with a copy, make sure to create a copy of the array before using array_shift(). You can do this using the array_slice() function:
php $originalArray = [1, 2, 3, 4, 5]; $copyArray = $originalArray; // Create a copy $firstElement = array_shift($copyArray); // $firstElement contains 1 // $copyArray contains [2, 3, 4, 5] // $originalArray remains unchanged [1, 2, 3, 4, 5]
This ensures that your original data remains intact while you manipulate the copy.
5. Returning false on Failure
In addition to returning null for empty arrays, array_shift() returns false on failure. This can happen if you attempt to shift an element from a non-array variable. It’s a good practice to check the return value to ensure the operation was successful:
php $result = array_shift($nonArrayVariable); if ($result === false) { // Handle the failure }
By checking the return value, you can gracefully handle unexpected situations.
6. Performance Considerations
While array_shift() is a convenient function for removing elements from the beginning of an array, it may not be the most efficient choice for large arrays. This is because shifting elements in a PHP array requires reindexing all the remaining elements. The larger the array, the more time-consuming this operation becomes.
If you need to perform frequent removals at the beginning of an array with large datasets, consider using other data structures such as a doubly linked list or a queue implemented with SplQueue. These structures provide more efficient ways to handle this type of operation.
Conclusion
In this guide, we’ve explored PHP’s array_shift() function and learned how to use it effectively. This function is a valuable tool for removing and retrieving the first element of an array, making it useful in various scenarios, from managing queues to parsing CSV data. Remember to handle edge cases, create copies of arrays if needed, and be mindful of performance considerations when working with large datasets. By mastering array_shift(), you’ll have a powerful tool at your disposal for array manipulation in PHP.
Array manipulation is just one aspect of PHP development. To become a proficient PHP developer, consider exploring other array functions and PHP’s vast ecosystem. With practice and experience, you’ll be well-equipped to tackle a wide range of web development challenges using PHP. Happy coding!
Table of Contents
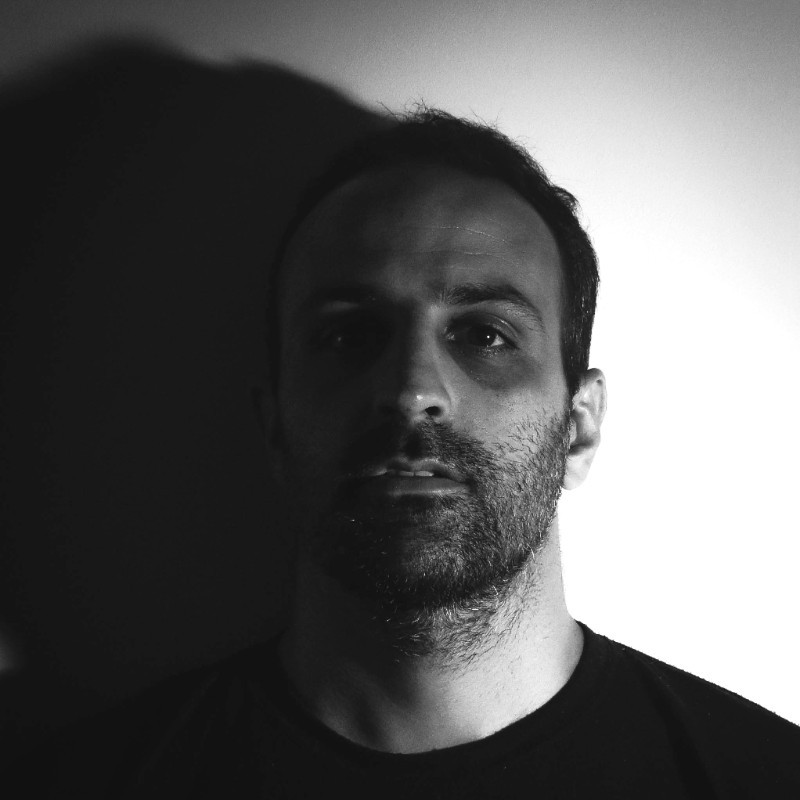
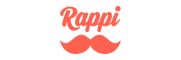