Unveiling the Power of PHP’s array_slice() Function
When it comes to working with arrays in PHP, developers often find themselves in situations where they need to extract a portion of an array or perform various array manipulations. This is where the array_slice() function comes to the rescue. This powerful function allows you to slice and dice arrays with ease, making your code more efficient and readable.
Table of Contents
In this blog post, we will delve deep into the world of PHP’s array_slice() function. We’ll explore its syntax, parameters, and usage, and provide you with real-world examples of how it can simplify your code and improve your PHP programming skills.
1. Understanding the Basics
Before we dive into the intricacies of array_slice(), let’s start with the basics. The array_slice() function is used to extract a slice of an array. A slice, in this context, refers to a portion of the array, typically determined by specifying a start and end point. It can be an essential tool for tasks like pagination, data filtering, and more.
1.1. Syntax
The syntax of array_slice() is quite straightforward:
php array_slice(array $input, int $offset, ?int $length = null, bool $preserve_keys = false)
Let’s break down each parameter:
- array $input: This is the input array from which you want to extract a slice.
- int $offset: The starting index of the slice. If the offset is non-negative, the slice will start at that index. If it’s negative, the slice will start that many elements from the end of the array.
- ?int $length: (Optional) The length of the slice. If specified and positive, the slice will contain that many elements. If negative, the slice will stop that many elements from the end of the array. If omitted or set to null, the slice will continue to the end of the array.
- bool $preserve_keys: (Optional) If set to true, the keys of the original array will be preserved in the resulting slice. If set to false (the default), the resulting slice will have re-indexed keys.
Now that we have a good grasp of the function’s syntax, let’s explore some practical use cases and examples.
2. Extracting a Slice from an Array
2.1. Getting a Range of Elements
One common use case for array_slice() is to extract a range of elements from an array. Let’s say you have an array of numbers, and you want to get the numbers from index 2 to 5. You can achieve this with array_slice():
php $numbers = [1, 2, 3, 4, 5, 6, 7, 8]; $slice = array_slice($numbers, 2, 4); print_r($slice);
In this example, $slice will contain the elements [3, 4, 5, 6]. The function starts at index 2 (inclusive) and includes 4 elements from that point.
2.2. Using Negative Offset
You can also use negative offsets to count elements from the end of the array. Let’s modify the previous example to get the last three elements of the array:
php $numbers = [1, 2, 3, 4, 5, 6, 7, 8]; $slice = array_slice($numbers, -3); print_r($slice);
In this case, $slice will contain the elements [6, 7, 8]. The negative offset -3 indicates that we want to start three elements from the end of the array.
3. Preserving Keys
By default, array_slice() re-indexes the keys of the resulting slice. However, there are situations where you may want to preserve the keys from the original array. You can achieve this by setting the $preserve_keys parameter to true.
Let’s look at an example where preserving keys is essential. Suppose you have an associative array representing user data, and you want to extract the first two users based on their IDs:
php $users = [ 'user1' => ['id' => 1, 'name' => 'Alice'], 'user2' => ['id' => 2, 'name' => 'Bob'], 'user3' => ['id' => 3, 'name' => 'Charlie'], // ... ]; $firstTwoUsers = array_slice($users, 0, 2, true); print_r($firstTwoUsers);
Without preserving keys ($preserve_keys set to false), the resulting slice would re-index the array keys starting from 0. However, by setting $preserve_keys to true, the keys are retained, and $firstTwoUsers will contain:
php Array ( [user1] => Array ( [id] => 1 [name] => Alice ) [user2] => Array ( [id] => 2 [name] => Bob ) )
4. Practical Use Cases
Now that we’ve covered the basics, let’s explore some practical use cases where array_slice() can be incredibly useful in PHP development.
4.1. Pagination
Pagination is a common requirement for web applications when displaying large sets of data. You need to display a subset of records on each page while allowing users to navigate through the pages. array_slice() makes it easy to implement pagination for arrays.
Here’s a simplified example of paginating an array of articles:
php $articles = [ // An array of articles ]; $page = isset($_GET['page']) ? (int)$_GET['page'] : 1; $perPage = 10; $start = ($page - 1) * $perPage; $paginatedArticles = array_slice($articles, $start, $perPage); foreach ($paginatedArticles as $article) { // Display the article }
With this code, you can easily control which portion of the $articles array is displayed on each page by adjusting the $_GET[‘page’] parameter. The array_slice() function extracts the appropriate slice for each page, simplifying your pagination logic.
4.2. Filtering Data
Another common task is filtering data based on specific criteria. Suppose you have an array of products, and you want to filter out products that are out of stock:
php $products = [ ['name' => 'Product A', 'stock' => 5], ['name' => 'Product B', 'stock' => 0], ['name' => 'Product C', 'stock' => 3], // ... ]; $filteredProducts = array_filter($products, function ($product) { return $product['stock'] > 0; }); print_r($filteredProducts);
In this case, array_filter() is used to remove products with zero stock. However, what if you want to limit the number of products returned, perhaps for display on a page? You can combine array_filter() and array_slice() to achieve this:
php $filteredProducts = array_filter($products, function ($product) { return $product['stock'] > 0; }); $page = isset($_GET['page']) ? (int)$_GET['page'] : 1; $perPage = 10; $start = ($page - 1) * $perPage; $paginatedProducts = array_slice($filteredProducts, $start, $perPage); foreach ($paginatedProducts as $product) { // Display the product }
This code first filters the products to exclude those with zero stock and then uses array_slice() to paginate the results. It’s a powerful combination for managing and displaying filtered data efficiently.
5. Implementing Custom Pagination Logic
While the previous pagination example used a straightforward approach, you might encounter scenarios where you need to implement custom pagination logic. For instance, you may want to display five items per page, but your URL structure or design requires a different pattern.
Let’s say you want to display items in a grid with three items per row, and you want to paginate by rows instead of individual items. You can use array_slice() to create custom pagination logic:
php $items = [ 'Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5', 'Item 6', 'Item 7', 'Item 8', 'Item 9', // ... ]; $page = isset($_GET['page']) ? (int)$_GET['page'] : 1; $itemsPerRow = 3; $rowsPerPage = 2; $startRow = ($page - 1) * $rowsPerPage; $startItem = $startRow * $itemsPerRow; $paginatedItems = array_slice($items, $startItem, $itemsPerRow * $rowsPerPage); foreach ($paginatedItems as $item) { // Display the item }
In this example, we use $itemsPerRow and $rowsPerPage to determine the number of items to display on each page. The custom logic in the foreach loop ensures that items are displayed in rows, accommodating the unique design requirement.
Conclusion
The array_slice() function in PHP is a versatile tool for array manipulation and data extraction. Whether you need to implement pagination, filter data, or create custom pagination logic, array_slice() simplifies the process and makes your code more maintainable.
In this blog post, we explored the syntax and parameters of array_slice(), along with various practical use cases and examples. By incorporating this function into your PHP projects, you can enhance their efficiency and readability, ultimately becoming a more proficient PHP developer.
Next time you find yourself working with arrays in PHP, remember the power of array_slice() and how it can streamline your code and improve your programming experience. Happy coding!
Now that you’ve unveiled the power of array_slice(), dive into array manipulation in PHP with confidence. Whether you’re implementing pagination, filtering data, or creating custom logic, this function has got you covered. Happy coding!
Table of Contents
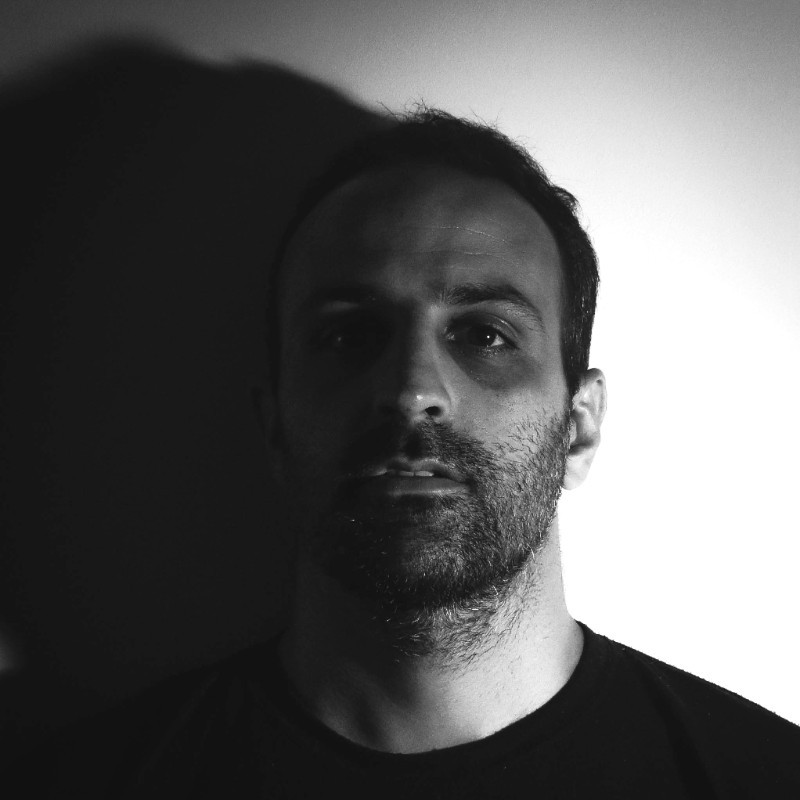
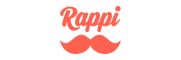