Making Sense of PHP’s array_unique() Function
PHP is a versatile scripting language widely used for web development. When working with arrays in PHP, you often encounter situations where you need to eliminate duplicate values. The array_unique() function comes to the rescue, simplifying this task. In this blog post, we’ll explore the ins and outs of PHP’s array_unique() function, covering its usage, nuances, and best practices.
Table of Contents
1. Understanding PHP Arrays
Before we dive into array_unique(), let’s have a quick review of PHP arrays. An array in PHP is a versatile data structure that can hold multiple values. These values can be of various data types, making PHP arrays incredibly flexible. You can create indexed arrays, associative arrays, or even multidimensional arrays, depending on your needs.
Here’s a simple example of an indexed array in PHP:
php $fruits = ['apple', 'banana', 'cherry', 'banana', 'date'];
In this array, we have a list of fruits, including some duplicates. Suppose we want to remove these duplicates and get a unique list of fruits. This is where array_unique() comes into play.
2. Introduction to array_unique()
The array_unique() function in PHP is designed to remove duplicate values from an array while preserving the original order of elements. It is a handy tool when you need to process data without redundancy. The function takes an array as its argument and returns a new array with duplicate values removed.
2.1. Basic Syntax
Here’s the basic syntax of the array_unique() function:
php array_unique(array $array, int $flags = SORT_STRING)
- $array: The input array from which you want to remove duplicates.
- $flags (optional): A sorting type flag. This flag is optional and determines how the function treats elements. By default, it uses SORT_STRING, which compares elements as strings. You can change this behavior by passing other flags like SORT_REGULAR, SORT_NUMERIC, or SORT_LOCALE_STRING.
2.2. Using array_unique()
Let’s use the $fruits array from earlier to demonstrate how array_unique() works:
php $fruits = ['apple', 'banana', 'cherry', 'banana', 'date']; $uniqueFruits = array_unique($fruits);
After calling array_unique($fruits), the $uniqueFruits array will contain:
php ['apple', 'banana', 'cherry', 'date']
As you can see, the duplicate value “banana” has been removed from the resulting array. The order of the elements is preserved.
3. Understanding SORT_FLAG Options
The $flags parameter of array_unique() allows you to control how duplicate values are treated during comparison. Let’s explore the different SORT_FLAG options available:
3.1. SORT_REGULAR (Default)
When you don’t specify a flag or use SORT_REGULAR, the function performs a regular comparison. It treats elements as they are without changing their data types. This is the default behavior we’ve seen in the previous example.
3.2. SORT_NUMERIC
If you want to treat elements as numbers, you can use the SORT_NUMERIC flag. This means the function will convert elements to numbers and compare them accordingly:
php $numbers = [2, 4, '6', 8, '10']; $uniqueNumbers = array_unique($numbers, SORT_NUMERIC);
After calling array_unique($numbers, SORT_NUMERIC), the $uniqueNumbers array will contain:
php [2, 4, '6', 8]
Notice that “10” is considered a duplicate of “8” when treated as numbers, so it’s removed from the result.
3.3. SORT_STRING
The SORT_STRING flag (which is the default) treats elements as strings for comparison. This means that data types are preserved, and elements are compared as they are:
php $mixedData = [42, '42', 42.0, '42.0']; $uniqueMixedData = array_unique($mixedData, SORT_STRING);
After calling array_unique($mixedData, SORT_STRING), the $uniqueMixedData array will contain:
php [42, '42', 42.0, '42.0']
The function preserves all elements because they are considered different when treated as strings.
3.4. SORT_LOCALE_STRING
If you need to perform comparisons based on the current locale, you can use the SORT_LOCALE_STRING flag. This is useful for handling multilingual data:
php $localeData = ['café', 'cafe', 'cafeteria']; $uniqueLocaleData = array_unique($localeData, SORT_LOCALE_STRING);
After calling array_unique($localeData, SORT_LOCALE_STRING), the $uniqueLocaleData array will contain:
php ['café', 'cafe']
Here, “cafe” and “café” are considered duplicates when using locale-based comparison.
4. Handling Associative Arrays
So far, we’ve been working with indexed arrays, but array_unique() also works with associative arrays. However, there’s a caveat to keep in mind. When you use array_unique() on an associative array, it doesn’t consider the keys; it only operates on the values.
Let’s look at an example:
php $associativeData = [ 'one' => 'apple', 'two' => 'banana', 'three' => 'cherry', 'four' => 'banana', ]; $uniqueAssociativeData = array_unique($associativeData);
After calling array_unique($associativeData), the $uniqueAssociativeData array will contain:
php [ 'one' => 'apple', 'two' => 'banana', 'three' => 'cherry', ]
As you can see, the duplicate value “banana” was removed from the resulting array, but the keys (‘one’, ‘two’, ‘three’) were not affected.
5. Removing Duplicate Values from Multidimensional Arrays
In real-world applications, you may often encounter multidimensional arrays that contain nested arrays or associative arrays. Removing duplicates from such arrays requires a slightly different approach.
Let’s consider a multidimensional array with duplicate subarrays:
php $multiDimData = [ ['id' => 1, 'name' => 'John'], ['id' => 2, 'name' => 'Jane'], ['id' => 1, 'name' => 'John'], // Duplicate ['id' => 3, 'name' => 'Jim'], ];
To remove duplicates based on the subarray’s values, we can use a combination of array_map() and array_unique(). Here’s how you can do it:
php $uniqueMultiDimData = array_map('unserialize', array_unique(array_map('serialize', $multiDimData)));
In this code:
- We use array_map(‘serialize’, $multiDimData) to serialize each subarray. Serialization converts each subarray into a string representation that includes keys and values.
- We then use array_unique() to remove duplicates based on the serialized strings. This ensures that identical subarrays are considered duplicates and removed.
- Finally, we use array_map(‘unserialize’, …) to deserialize the unique serialized strings back into subarrays.
After running the above code, $uniqueMultiDimData will contain:
php [ ['id' => 1, 'name' => 'John'], ['id' => 2, 'name' => 'Jane'], ['id' => 3, 'name' => 'Jim'], ]
Now, we have a multidimensional array with duplicate subarrays removed.
6. Best Practices and Tips
When working with array_unique(), here are some best practices and tips to keep in mind:
6.1. Understand the SORT_FLAG Options
Be aware of the different SORT_FLAG options (SORT_REGULAR, SORT_NUMERIC, SORT_STRING, and SORT_LOCALE_STRING) and choose the one that suits your specific needs. Using the correct flag ensures accurate duplicate removal.
6.2. Remember the Key-Preservation Behavior
For associative arrays, remember that array_unique() only removes duplicate values and doesn’t consider the keys. If key preservation is essential, consider using a custom approach or iterating through the array to remove duplicates based on keys.
6.3. Handle Multidimensional Arrays Carefully
When dealing with multidimensional arrays, the process for removing duplicates becomes more complex. Utilize a combination of array_map() and serialize()/unserialize() to ensure accurate duplicate removal while preserving the structure of the array.
6.4. Maintain Original Array Order
One advantage of array_unique() is that it maintains the original order of elements. If the order of your array matters, array_unique() is a suitable choice for removing duplicates.
6.5. Keep Performance in Mind
array_unique() is a straightforward solution for removing duplicates, but it may not be the most efficient for very large arrays. If performance is a concern, consider alternative approaches, such as using loops and custom logic to eliminate duplicates.
Conclusion
PHP’s array_unique() function is a valuable tool for removing duplicate values from arrays in a simple and efficient way. By understanding the function’s usage and the available SORT_FLAG options, you can effectively manage arrays and ensure your data remains clean and free from redundancy. Whether you’re working with indexed arrays, associative arrays, or multidimensional arrays, array_unique() offers a convenient solution for maintaining data integrity in your PHP projects.
Incorporate these best practices and tips into your PHP development workflow, and you’ll be well-equipped to handle duplicate values in arrays with ease.
Table of Contents
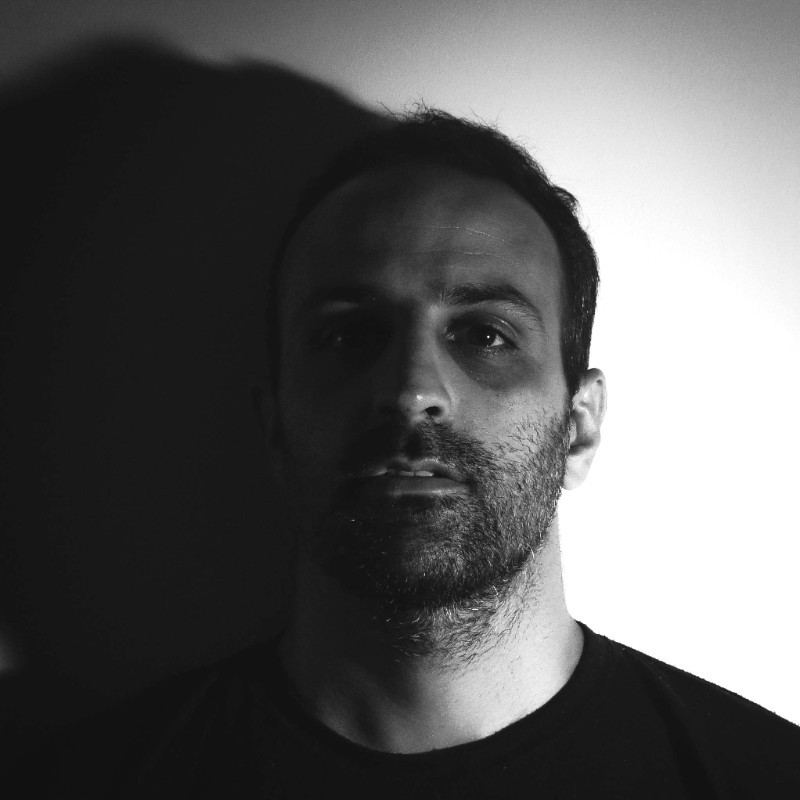
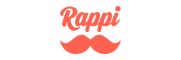