Unpacking the PHP’s array_unshift() Function
PHP offers a rich array of functions to manipulate arrays, and array_unshift() is one of the lesser-known gems. This powerful function allows you to effortlessly add one or more elements to the beginning of an array. In this blog, we will explore the ins and outs of array_unshift(), from its basic syntax to real-world use cases and code samples.
Table of Contents
1. Understanding array_unshift()
Before we dive into the practical applications, let’s break down the syntax and parameters of array_unshift().
1.1. Syntax
The basic syntax of the array_unshift() function is as follows:
php array_unshift(array &$array, mixed $value1 [, mixed $value2 [, mixed $... ]]) : int
- array &$array: A reference to the array to which you want to add elements.
- mixed $value1 [, mixed $value2 [, mixed $… ]]: One or more values to add to the beginning of the array.
- int: The new number of elements in the array after adding the values.
1.2. Key Points
- array_unshift() takes the input array by reference. This means that any changes made inside the function will affect the original array.
- It returns an integer representing the new number of elements in the array.
2. Adding Elements to the Beginning of an Array
Let’s start with a simple example to illustrate how array_unshift() works:
php $fruits = array("banana", "apple", "cherry"); array_unshift($fruits, "orange"); print_r($fruits);
Output:
csharp Array ( [0] => orange [1] => banana [2] => apple [3] => cherry )
In this example, we have an array $fruits containing three elements: “banana,” “apple,” and “cherry.” We use array_unshift() to add “orange” to the beginning of the array, resulting in a modified array with four elements.
3. Adding Multiple Elements
You can also add multiple elements to the beginning of an array using array_unshift(). Here’s an example:
php $numbers = array(4, 5, 6); array_unshift($numbers, 1, 2, 3); print_r($numbers);
Output:
csharp Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
In this case, we added three values (“1,” “2,” and “3”) to the beginning of the $numbers array, resulting in a modified array with six elements.
4. Use Cases for array_unshift()
Now that you understand the basic usage of array_unshift(), let’s explore some practical use cases where this function can come in handy.
4.1. Building a Queue
A common use case for array_unshift() is implementing a simple queue data structure. You can use an array to represent a queue, with array_unshift() for enqueueing items and array_pop() for dequeuing items. Here’s an example:
php $queue = array(); // Enqueue items array_unshift($queue, "item1"); array_unshift($queue, "item2"); array_unshift($queue, "item3"); // Dequeue items $dequeuedItem = array_pop($queue); echo $dequeuedItem; // Outputs: "item1"
In this example, we add items to the beginning of the $queue array using array_unshift(), and then dequeue them using array_pop(). This simulates a simple queue structure where items are processed in a first-in, first-out (FIFO) order.
4.2. Maintaining a History
You can use array_unshift() to maintain a history of actions or events. For instance, if you’re building a logging system, you can prepend log entries to an array to keep track of the most recent events:
php $log = array(); function logEvent($message) { global $log; $timestamp = date("Y-m-d H:i:s"); $logEntry = "$timestamp - $message"; array_unshift($log, $logEntry); } // Log some events logEvent("User login successful"); logEvent("File uploaded"); logEvent("Error: Invalid input"); // Print the recent log entries print_r($log);
In this example, the logEvent() function adds log entries to the beginning of the $log array using array_unshift(). This ensures that the most recent events appear at the top of the log.
4.3. Reversing an Array
You can quickly reverse the elements of an array using array_unshift() and a loop. Here’s how you can do it:
php $originalArray = array(1, 2, 3, 4, 5); $reversedArray = array(); foreach ($originalArray as $item) { array_unshift($reversedArray, $item); } print_r($reversedArray);
Output:
csharp Array ( [0] => 5 [1] => 4 [2] => 3 [3] => 2 [4] => 1 )
In this example, we start with an $originalArray and create an empty $reversedArray. We then iterate through the original array, adding each item to the beginning of the reversed array using array_unshift(). This effectively reverses the order of elements.
5. Error Handling and Considerations
While array_unshift() is a powerful function, it’s essential to handle potential issues gracefully.
5.1. Checking for Success
Since array_unshift() modifies the original array in place, you should check if the operation was successful. This can be done by verifying the return value, which is the new number of elements in the array after the operation. If the return value is not as expected, it may indicate an issue.
php $result = array_unshift($array, $value); if ($result !== false) { // Operation was successful } else { // Operation failed }
5.2. Array Size Limitations
Be cautious when adding elements to an array, especially if it’s extensive. PHP imposes memory limits, so adding a large number of elements can lead to memory exhaustion and performance issues. Consider your system’s memory constraints and optimize your code accordingly.
Conclusion
PHP’s array_unshift() function is a versatile tool for manipulating arrays. Whether you’re building data structures, logging events, or simply rearranging data, understanding how to add elements to the beginning of an array can be incredibly useful. By mastering this function, you’ll have another valuable tool in your PHP development toolkit. So, go ahead and experiment with array_unshift() to unlock its full potential in your PHP projects.
In this blog post, we covered the basic syntax of array_unshift(), demonstrated its usage with code examples, and explored various practical applications. Armed with this knowledge, you can take your PHP programming skills to the next level and make your code more efficient and versatile. Happy coding!
Table of Contents
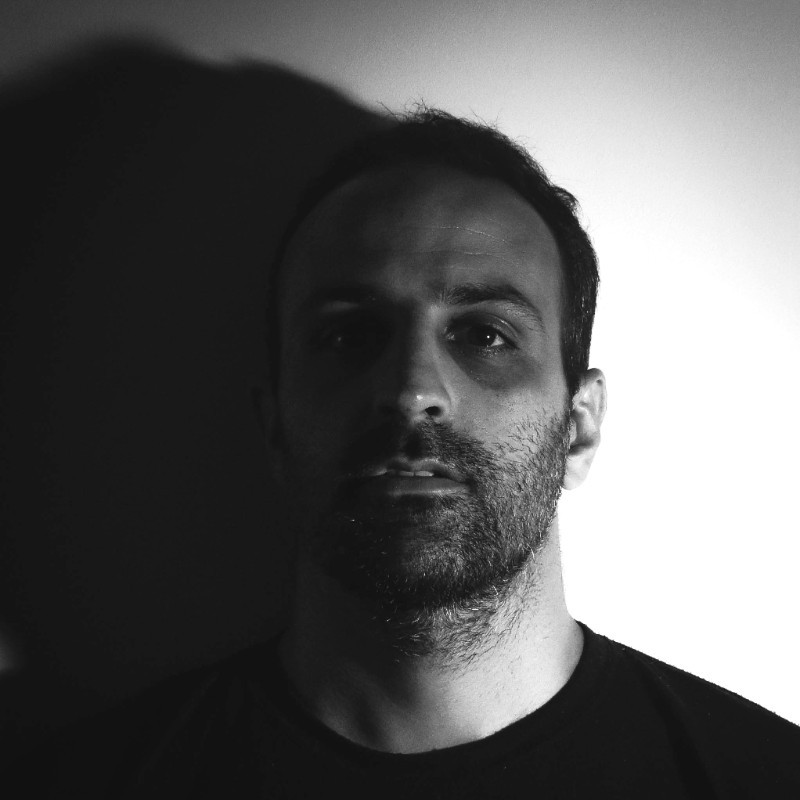
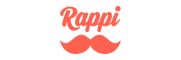