Making Sense of PHP’s array_values() Function
Arrays are fundamental data structures in PHP, and they play a crucial role in many web applications. Often, you may find yourself needing to extract the values from an array while preserving their order or simply reindexing an array. PHP provides a versatile function called array_values() that can help you achieve these tasks effortlessly.
Table of Contents
In this blog post, we’ll explore the array_values() function in depth. We’ll cover its purpose, syntax, and practical use cases through code examples. By the end, you’ll have a solid understanding of how to make the most of this function in your PHP projects.
1. Understanding array_values()
The array_values() function is used to extract all the values from an associative array and return them as a numerically indexed array. It effectively reindexes the array, starting from index 0 and incrementing sequentially. This function is particularly useful when you need to remove the keys associated with the values or when you want to ensure a consistent, zero-based index for your array elements.
1.1. Syntax
Here’s the syntax for the array_values() function:
php array array_values(array $array)
- array: The input array whose values you want to extract and reindex.
The function takes a single parameter, which is the array you want to process, and returns a new array containing only the values, reindexed numerically.
2. Basic Usage of array_values()
Let’s start with a simple example to demonstrate how the array_values() function works. Consider the following associative array:
php $studentScores = [ "Alice" => 95, "Bob" => 88, "Charlie" => 92, "David" => 78, ];
If we want to extract just the scores from this array and reindex them numerically, we can use array_values() like this:
php $scores = array_values($studentScores);
Now, let’s explore the contents of the $scores array:
php print_r($scores);
The output will be:
php Array ( [0] => 95 [1] => 88 [2] => 92 [3] => 78 )
As you can see, the array_values() function removed the keys (“Alice,” “Bob,” etc.) and reindexed the values with numeric keys, starting from 0.
3. Preserving Original Order
One of the important things to note about array_values() is that it preserves the original order of elements in the array. This means that the values in the resulting array will be in the same order as they were in the original associative array.
Consider this example:
php $fruits = [ "b" => "banana", "a" => "apple", "c" => "cherry", "d" => "date", ];
If we use array_values() on the $fruits array, like so:
php $indexedFruits = array_values($fruits);
The resulting $indexedFruits array will maintain the order of the values:
php print_r($indexedFruits);
Output:
php Array ( [0] => banana [1] => apple [2] => cherry [3] => date )
This behavior is particularly useful when you need to work with data that relies on the order of elements.
4. Practical Use Cases
Now that we have covered the basics, let’s dive into some practical use cases where the array_values() function can be incredibly handy.
4.1. Removing Keys from an Array
There are situations where you might want to remove the keys from an associative array and work with just the values. This can be helpful when you need to perform operations on the values without considering their associated keys.
Consider the following example, where we have an array of user roles:
php $userRoles = [ "admin" => "Administrator", "editor" => "Editor", "user" => "Regular User", ];
If you want to extract and work with just the role names (values), you can use array_values():
php $roles = array_values($userRoles);
Now, the $roles array contains only the role names, without the keys:
php print_r($roles);
Output:
php Array ( [0] => Administrator [1] => Editor [2] => Regular User )
This allows you to easily iterate through and manipulate the role names without the need to consider the keys.
4.2. Reindexing an Array
Another common use case for array_values() is reindexing an array with numeric keys, especially if you want to reset the keys to start from 0 and increment sequentially.
Consider this example, where we have an array of colors:
php $colors = [ "red", "green", "blue", "yellow", ];
If you want to reindex this array with numeric keys, you can use array_values():
php $indexedColors = array_values($colors);
Now, the $indexedColors array has numeric keys starting from 0:
php print_r($indexedColors);
Output:
php Array ( [0] => red [1] => green [2] => blue [3] => yellow )
This reindexing can be beneficial when you need to ensure a consistent and sequential key order for array elements.
4.3. Converting an Associative Array to a Numerically Indexed Array
In some scenarios, you might receive data in the form of an associative array but prefer to work with it as a numerically indexed array. array_values() makes this conversion straightforward.
Imagine you have an associative array representing a book:
php $book = [ "title" => "The Great Gatsby", "author" => "F. Scott Fitzgerald", "year" => 1925, ];
If you want to transform this associative array into a numerically indexed array, you can do so with array_values():
php $indexedBook = array_values($book);
The $indexedBook array will contain the values from the original associative array:
php print_r($indexedBook);
Output:
php Array ( [0] => The Great Gatsby [1] => F. Scott Fitzgerald [2] => 1925 )
This transformation can be beneficial when you need to pass the data to a function or library that expects a numerically indexed array.
5. Advanced Usage
While array_values() is straightforward and primarily used for extracting values and reindexing arrays, you can combine it with other PHP array functions to perform more complex operations.
5.1. Combining array_values() with array_filter()
Let’s say you have an associative array with both numeric and string keys, and you want to extract only the numeric values. You can achieve this by combining array_values() with array_filter().
Consider the following example:
php $data = [ 0 => "apple", "one" => "banana", 2 => "cherry", "three" => "date", ];
If you want to get an array containing only the numeric values, you can use array_filter() to filter the values and then apply array_values() to reindex the result:
php $numericValues = array_values(array_filter($data, 'is_numeric'));
Now, the $numericValues array contains only the numeric values:
php print_r($numericValues);
Output:
php Array ( [0] => apple [1] => cherry )
In this example, array_filter() is used to filter the numeric values, and array_values() is subsequently applied to reindex the result.
5.2. Combining array_values() with array_map()
Another powerful combination is using array_values() in conjunction with array_map() to perform operations on each element of an array while maintaining numeric keys.
Suppose you have an array of prices, and you want to apply a discount to each price. You can achieve this using array_map() along with array_values():
php $prices = [ 100, 75, 50, 25, ]; // Define a discount function function applyDiscount($price) { return $price * 0.9; // Apply a 10% discount } // Apply the discount to each price and reindex the array $discountedPrices = array_values(array_map('applyDiscount', $prices));
Now, the $discountedPrices array contains the discounted prices with numeric keys:
php print_r($discountedPrices);
Output:
php Array ( [0] => 90 [1] => 67.5 [2] => 45 [3] => 22.5 )
In this example, array_map() applies the applyDiscount() function to each element of the $prices array, and array_values() is used to reindex the result.
Conclusion
PHP’s array_values() function is a valuable tool for extracting values from associative arrays and reindexing arrays with numeric keys. It simplifies common tasks like removing keys from an array, preserving original order, and converting associative arrays to numerically indexed arrays. When combined with other array functions, it becomes even more powerful, allowing you to perform complex operations on your data.
By understanding and leveraging array_values(), you can write more efficient and readable PHP code, making your web development projects more manageable and maintainable. So, the next time you need to work with array values in PHP, remember the versatility of the array_values() function and how it can simplify your tasks.
Table of Contents
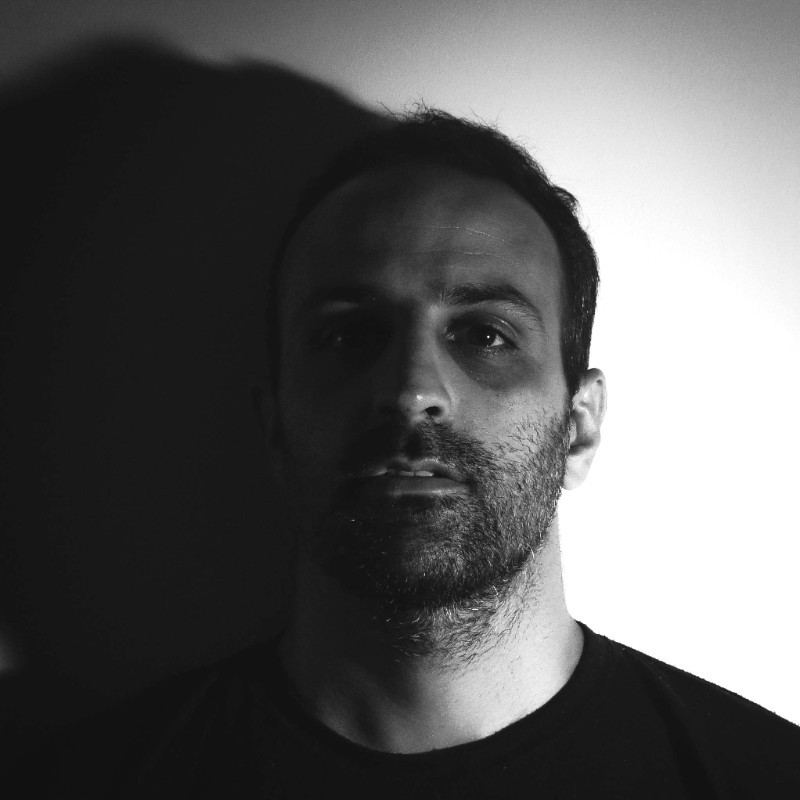
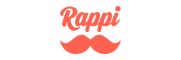