Taming PHP’s array_walk() Function: A Detailed Guide
PHP is a versatile and widely-used scripting language known for its rich array of built-in functions. Among these functions, array_walk() stands out as a powerful tool for manipulating arrays. If you want to take your PHP programming skills to the next level, mastering array_walk() is essential. In this detailed guide, we will delve into the depths of array_walk(), exploring its functionality, use cases, and best practices, all accompanied by practical examples.
Table of Contents
1. What is array_walk()?
array_walk() is a built-in PHP function that allows you to iterate over each element of an array and apply a user-defined callback function to each element. This callback function can perform various operations on the array elements, such as modifying their values, appending new elements, or even removing elements based on specific conditions.
2. The Anatomy of array_walk()
Before we dive into practical examples, let’s take a closer look at the syntax and parameters of array_walk().
php array_walk(array &$array, callable $callback [, mixed $userdata = NULL]): bool
- $array (required): The input array that you want to iterate over and modify.
- $callback (required): The user-defined callback function that will be applied to each element of the array.
- $userdata (optional): An optional parameter that allows you to pass additional data to the callback function.
The callback function should accept at least two parameters:
php function callback(&$value, $key [, $userdata = NULL]): void
- $value: The current element’s value.
- $key: The current element’s key (index).
- $userdata: Any user-defined data you want to pass.
Now that we have a basic understanding of array_walk(), let’s explore some practical use cases.
Use Case 1: Modifying Array Elements
One of the most common use cases for array_walk() is modifying the elements of an array. Let’s say you have an array of numbers, and you want to square each element. Here’s how you can do it using array_walk():
php // Sample array of numbers $numbers = [1, 2, 3, 4, 5]; // Callback function to square each element function square(&$value, $key) { $value = $value * $value; } // Apply the callback function to each element array_walk($numbers, 'square'); // $numbers now contains [1, 4, 9, 16, 25]
In this example, the square() callback function is applied to each element of the $numbers array, resulting in each element being squared.
Use Case 2: Filtering Array Elements
array_walk() can also be used to filter elements from an array based on specific conditions. Suppose you have an array of numbers, and you want to remove all odd numbers. Here’s how you can achieve it:
php // Sample array of numbers $numbers = [1, 2, 3, 4, 5]; // Callback function to filter out odd numbers function filter_odd(&$value, $key) { if ($value % 2 !== 0) { unset($value); } } // Apply the callback function to filter odd numbers array_walk($numbers, 'filter_odd'); // $numbers now contains [2, 4]
In this example, the filter_odd() callback function checks if the current element is odd and removes it from the array using unset().
Use Case 3: Adding Elements to an Array
You can also use array_walk() to add new elements to an array. Suppose you have an array of names, and you want to add a greeting before each name. Here’s how you can achieve it:
php // Sample array of names $names = ['Alice', 'Bob', 'Charlie']; // Callback function to add a greeting function add_greeting(&$value, $key) { $value = "Hello, $value!"; } // Apply the callback function to add greetings array_walk($names, 'add_greeting'); // $names now contains ['Hello, Alice!', 'Hello, Bob!', 'Hello, Charlie!']
In this example, the add_greeting() callback function adds a greeting before each name in the $names array.
Use Case 4: Custom Data Manipulation
Sometimes, you may need to perform custom data manipulation on an array. For example, you might want to calculate the sum of all values in an array. Here’s how you can do it using array_walk():
php // Sample array of numbers $numbers = [1, 2, 3, 4, 5]; // Initialize a variable to store the sum $sum = 0; // Callback function to calculate the sum function calculate_sum(&$value, $key) { global $sum; $sum += $value; } // Apply the callback function to calculate the sum array_walk($numbers, 'calculate_sum'); // $sum now contains 15 (1 + 2 + 3 + 4 + 5)
In this example, the calculate_sum() callback function updates the $sum variable with the cumulative sum of the array elements.
Use Case 5: Passing Additional Data to the Callback
In some cases, you may need to pass additional data to the callback function. The $userdata parameter of array_walk() allows you to do just that. Let’s say you have an array of prices, and you want to apply a discount to each price based on a fixed discount rate:
php // Sample array of prices $prices = [100, 200, 300, 400, 500]; // Fixed discount rate $discountRate = 0.1; // 10% // Callback function to apply a discount function apply_discount(&$value, $key, $discountRate) { $value = $value - ($value * $discountRate); } // Apply the callback function with additional data (discount rate) array_walk($prices, 'apply_discount', $discountRate); // $prices now contains [90, 180, 270, 360, 450]
In this example, we pass the $discountRate as additional data to the apply_discount() callback function using the $userdata parameter.
3. Best Practices and Tips
Now that you’ve seen some practical examples of how to use array_walk(), let’s discuss some best practices and tips to keep in mind when working with this function:
3.1. Use & to Modify Array Elements
To modify array elements within the callback function, make sure to pass the $value parameter by reference using &. This allows you to directly modify the original array.
3.2. Avoid Changing the Array Structure
When modifying an array within the callback function, be cautious not to change the array’s structure. Altering keys or reordering elements can lead to unexpected results.
3.3. Use a Meaningful Callback Function Name
Choose descriptive names for your callback functions to make your code more readable and maintainable. A well-named function makes it easier for others (and your future self) to understand its purpose.
3.4. Consider Using Anonymous Functions
Instead of defining separate named functions for simple operations, you can use anonymous functions (closures) directly as callbacks. This can lead to more concise and readable code.
3.5. Be Mindful of Performance
While array_walk() is a versatile function, it may not always be the most efficient choice for certain tasks. For large arrays or complex operations, consider alternative approaches like foreach loops.
Conclusion
PHP’s array_walk() function is a valuable tool for manipulating arrays in various ways. Whether you need to modify elements, filter data, add elements, or perform custom data manipulation, array_walk() provides the flexibility to achieve your goals. By following best practices and understanding its usage, you can harness the full potential of this function in your PHP projects.
In this guide, we’ve covered the basics of array_walk() and explored practical use cases with code samples. Armed with this knowledge, you can confidently incorporate array_walk() into your PHP development toolkit, making your code more efficient and expressive.
Remember that mastering array_walk() is just one step in becoming a proficient PHP developer. Continuously expanding your knowledge of PHP’s functions and features will empower you to tackle a wide range of programming challenges with ease. Happy coding!
Start taming array_walk() today and unlock the potential of array manipulation in PHP.
Table of Contents
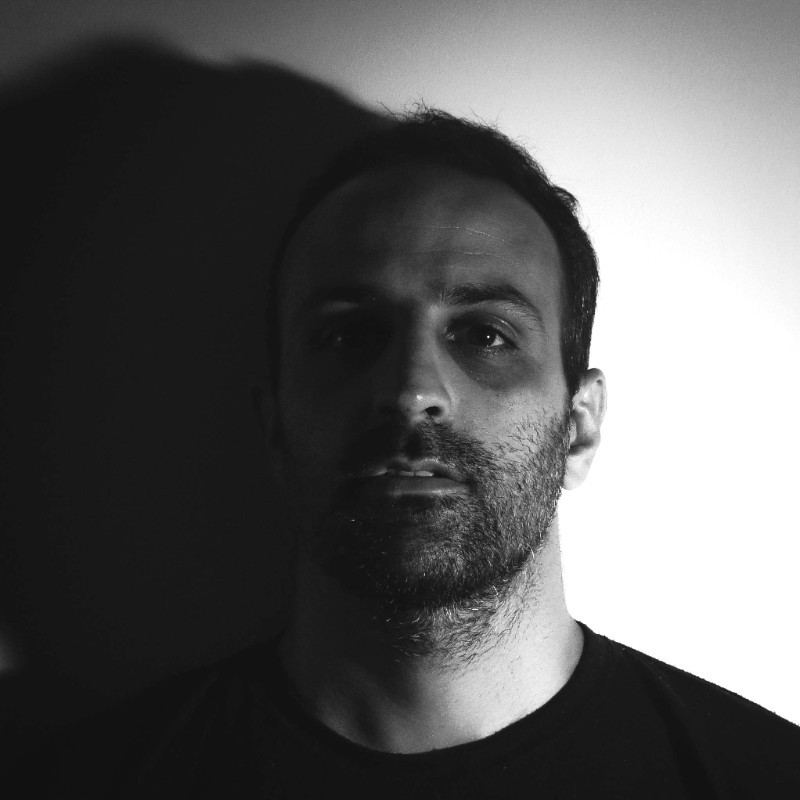
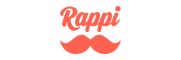