The Power of PHP: A Beginner’s Journey
In the realm of web development, PHP has established itself as a powerful and versatile programming language. Whether you are a seasoned developer or a beginner, PHP provides a robust foundation to build dynamic and interactive websites and applications. In this blog, we will embark on a beginner’s journey through PHP, exploring its key features, syntax, and functionality. We will delve into code samples to understand how PHP can be used effectively to enhance web development projects. Let’s dive in!
Getting Started with PHP
Before we begin exploring the intricacies of PHP, it is essential to set up a development environment. PHP is a server-side scripting language, which means it runs on the web server rather than the user’s browser. To start, you need a web server installed on your local machine, such as Apache or Nginx, along with PHP itself. Once the setup is complete, you’re ready to write your first PHP script.
php <?php echo "Hello, World!"; ?>
In the above example, we use the echo statement to display the famous “Hello, World!” message. PHP code is enclosed within <?php and ?> tags, allowing you to switch between HTML and PHP seamlessly.
Variables and Data Types
Like any programming language, PHP provides variables to store and manipulate data. Variables in PHP are dynamically typed, meaning their data type is determined at runtime. Let’s take a look at some code examples to understand how variables work in PHP.
php <?php $name = "John Doe"; $age = 25; $isStudent = true; echo "Name: " . $name . "<br>"; echo "Age: " . $age . "<br>"; echo "Is Student: " . ($isStudent ? "Yes" : "No") . "<br>"; ?>
In the above code, we define variables to store a name, age, and a boolean value indicating whether the person is a student. We then use the echo statement to display the values along with some additional text.
Control Structures and Loops
Control structures and loops play a vital role in shaping the flow of your PHP code. They enable you to make decisions and repeat actions based on specific conditions. Let’s explore a few control structures and loops commonly used in PHP.
php <?php $num = 10; if ($num > 0) { echo "The number is positive."; } elseif ($num < 0) { echo "The number is negative."; } else { echo "The number is zero."; } echo "<br>"; for ($i = 1; $i <= 5; $i++) { echo $i . " "; } echo "<br>"; $colors = ["red", "green", "blue"]; foreach ($colors as $color) { echo $color . " "; } ?>
In the above example, we use the if-elseif-else statement to check if a number is positive, negative, or zero. We then use a for loop to display numbers from 1 to 5 and a foreach loop to iterate over an array of colors.
Functions and Custom Code
Functions in PHP allow you to encapsulate a set of instructions into reusable blocks of code. They help improve code organization and maintainability. Let’s create a custom function to calculate the factorial of a number.
php <?php function factorial($n) { if ($n == 0 || $n == 1) { return 1; } else { return $n * factorial($n - 1); } } echo "Factorial of 5: " . factorial(5); ?>
In the above example, we define a recursive function called factorial that calculates the factorial of a given number. We then call the function and display the result.
Working with Databases
PHP has extensive support for working with databases, making it an excellent choice for developing dynamic web applications. Let’s explore how PHP interacts with databases using the popular MySQL database as an example.
php <?php // Establishing a database connection $conn = mysqli_connect("localhost", "username", "password", "database_name"); // Checking the connection if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } // Performing a database query $sql = "SELECT * FROM users"; $result = mysqli_query($conn, $sql); // Fetching and displaying data if (mysqli_num_rows($result) > 0) { while ($row = mysqli_fetch_assoc($result)) { echo "ID: " . $row["id"] . ", Name: " . $row["name"] . "<br>"; } } else { echo "No users found."; } // Closing the database connection mysqli_close($conn); ?>
In the above example, we establish a connection to a MySQL database, perform a SELECT query to retrieve user data, and display it using a loop. Finally, we close the database connection to free up resources.
Conclusion
In this beginner’s journey through PHP, we have only scratched the surface of this powerful programming language. PHP’s vast ecosystem, extensive documentation, and a vibrant community make it a compelling choice for web development projects of all sizes. With further exploration, you can leverage PHP’s features, libraries, and frameworks to build dynamic, secure, and scalable web applications. So, embark on your PHP journey today, embrace its power, and unlock your creativity in the world of web development!
Table of Contents
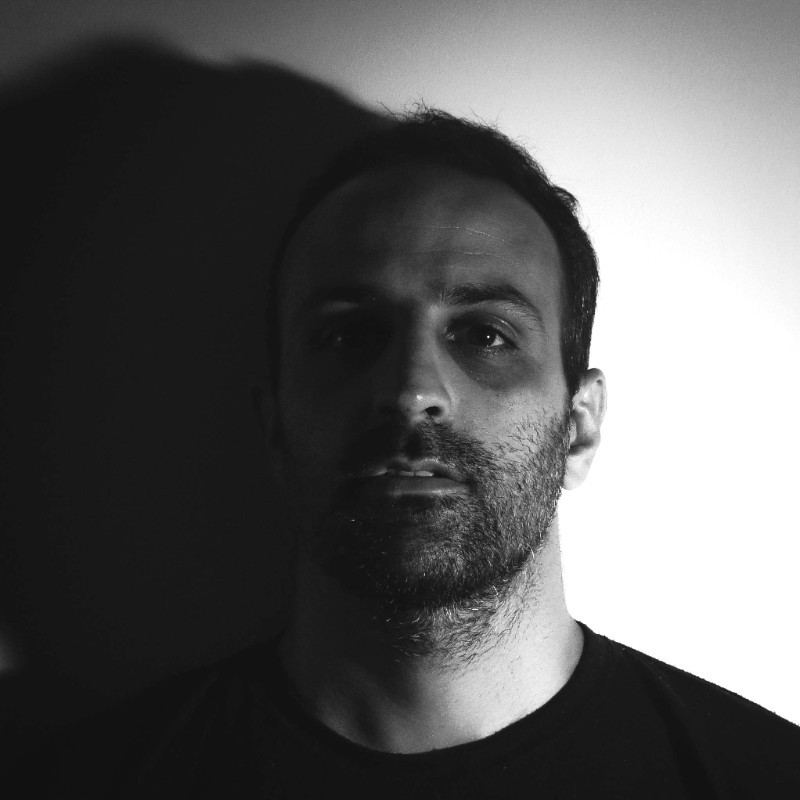
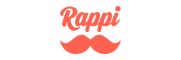