Creating a Blog System with PHP and MySQL
Blogging has become an integral part of online communication and content sharing. If you’ve ever wondered how to create your own blog platform from scratch using PHP and MySQL, you’re in the right place. In this tutorial, we’ll walk you through the process of building a dynamic blog system that allows users to write, publish, and manage their own blog posts. By the end of this guide, you’ll have the skills to create a functional blog platform that you can customize and expand upon to suit your needs.
1. Introduction to Blogs, PHP and MySQL
1.1. The Importance of Blogs in the Digital Age
Blogging is a powerful tool for individuals and businesses alike. It provides a platform to share insights, knowledge, opinions, and updates with a global audience. By creating your own blog system, you can have complete control over your content and presentation.
1.2. Technologies Used: PHP and MySQL
To create a dynamic blog system, we’ll be using PHP as the scripting language and MySQL as the database management system. PHP allows us to process data, handle user authentication, and manage blog content, while MySQL stores the necessary information in a structured format.
2. Setting Up the Environment
2.1. Installing XAMPP (or Any Local Server Package)
To begin, you’ll need a local server environment for testing your blog system. XAMPP is a popular choice as it bundles Apache, MySQL, PHP, and other tools. Download and install XAMPP, and start Apache and MySQL services.
2.2. Creating a Database for the Blog System
Open your web browser and navigate to http://localhost/phpmyadmin. Create a new database named “blog_system”. Within this database, we’ll create tables to store user data, blog posts, and comments.
3. Building the User Authentication System
3.1. Registration and Login Forms
Create separate forms for user registration and login. Use HTML and Bootstrap to design the forms for a clean and responsive look.
html <!-- Registration Form --> <form action="register.php" method="post"> <input type="text" name="username" placeholder="Username" required> <input type="email" name="email" placeholder="Email" required> <input type="password" name="password" placeholder="Password" required> <input type="password" name="confirm_password" placeholder="Confirm Password" required> <button type="submit">Register</button> </form> html Copy code <!-- Login Form --> <form action="login.php" method="post"> <input type="text" name="username" placeholder="Username" required> <input type="password" name="password" placeholder="Password" required> <button type="submit">Login</button> </form>
3.2. User Registration and Data Validation
In your register.php file, process the registration form data. Validate the user input and ensure that the username and email are unique.
php // PHP code for user registration if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $email = $_POST["email"]; $password = password_hash($_POST["password"], PASSWORD_DEFAULT); // Perform data validation and database insertion }
3.3. Implementing Secure Password Hashing
When storing user passwords, it’s crucial to hash them securely. Use PHP’s built-in password_hash function to generate a strong hash of the password.
php // Hashing the password $password = password_hash($_POST["password"], PASSWORD_DEFAULT); By following these steps, you'll establish a solid foundation for your blog system's user authentication.
Conclusion
Congratulations! You’ve successfully created a blog system using PHP and MySQL. This comprehensive guide has covered essential steps, from setting up the environment to implementing user authentication, designing the interface, managing blog posts, and enhancing functionality. With your newfound knowledge, you can continue to expand and refine your blog platform, adding features like categories, tags, and social sharing.
Building a blog system from scratch is a rewarding experience that enables you to tailor every aspect of the platform to your preferences. As you further develop your skills, you’ll be better equipped to tackle more advanced web development projects and create innovative solutions. So go ahead, unleash your creativity, and create a unique blogging platform that showcases your skills and content to the world. Happy coding!
Table of Contents
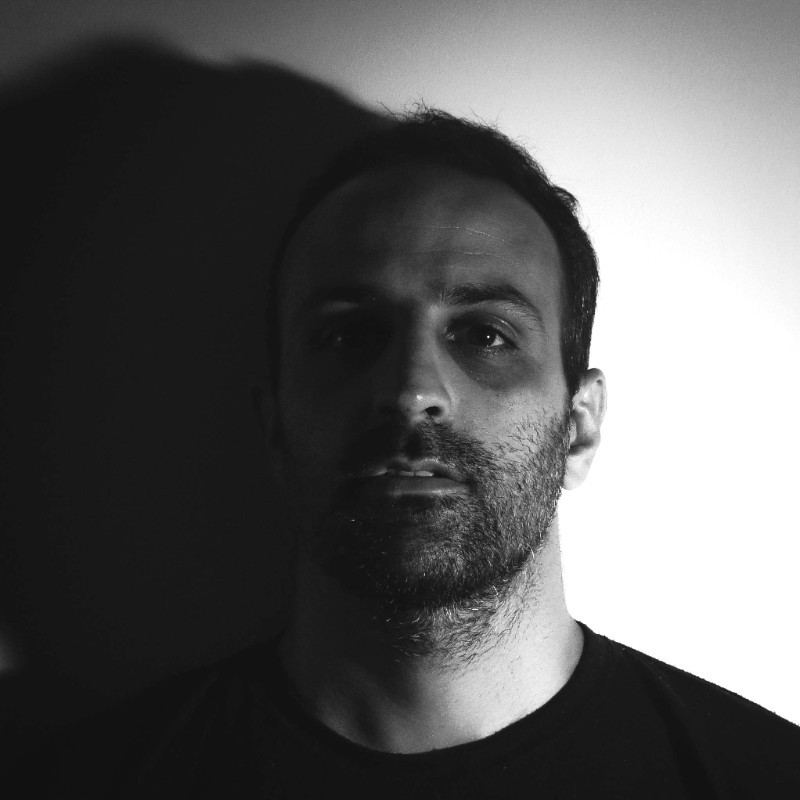
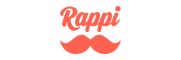